Assume a two-dimensional integer array has been declared in main(). Create a Java method named largestRowElement that returns a String and has the following parameters: A two-dimensional array of type integer An integer that represents the number of the row to be processed This method will find the largest element in the desired row and return a String containing the location and value of the largest element. A sample output would look similar to this: Largest element of row 0 is 25 in column 1
Assume a two-dimensional integer array has been declared in main(). Create a Java method named largestRowElement that returns a String and has the following parameters: A two-dimensional array of type integer An integer that represents the number of the row to be processed This method will find the largest element in the desired row and return a String containing the location and value of the largest element. A sample output would look similar to this: Largest element of row 0 is 25 in column 1
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
need help with my java code
![### Java Programming: Finding the Largest Element in a Row of a Two-Dimensional Array
Assume a two-dimensional integer array has been declared in the `main()` method.
#### Task
Create a Java method named `largestRowElement` that returns a `String` and has the following parameters:
1. **A two-dimensional array** of type integer.
2. **An integer** that represents the number of the row to be processed.
#### Method Functionality
This method will find the largest element in the specified row and return a `String` containing the location and value of the largest element.
**Sample Output:**
```
Largest element of row 0 is 25 in column 1
```
### Method Signature Example
```java
public String largestRowElement(int[][] array, int rowNum) {
// Method implementation goes here
}
```
**Explanation:**
- `int[][] array`: Represents the two-dimensional integer array.
- `int rowNum`: Represents the row number to be processed for finding the largest element.
- The method will identify the largest element in the specified row, determine its column index, and return this information in a formatted string.
### Example Usage of the Method
```java
public class Main {
public static void main(String[] args) {
int[][] array = {
{10, 25, 3},
{7, 18, 5},
{14, 6, 30}
};
Main obj = new Main();
String result = obj.largestRowElement(array, 0);
System.out.println(result); // Output: Largest element of row 0 is 25 in column 1
}
public String largestRowElement(int[][] array, int rowNum) {
int largestElement = array[rowNum][0];
int columnIndex = 0;
for (int i = 1; i < array[rowNum].length; i++) {
if (array[rowNum][i] > largestElement) {
largestElement = array[rowNum][i];
columnIndex = i;
}
}
return "Largest element of row " + rowNum + " is " + largestElement + " in column " + columnIndex;
}
}
```
In this implementation:
- We initialize `largestElement` to the first element in the specified row.
- We iterate through the row to find the](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F01257b92-4ba0-4433-8b92-a1a481280a93%2Fdd511050-0437-4644-95e5-0b1221bcf665%2Fsyh85mq.png&w=3840&q=75)
Transcribed Image Text:### Java Programming: Finding the Largest Element in a Row of a Two-Dimensional Array
Assume a two-dimensional integer array has been declared in the `main()` method.
#### Task
Create a Java method named `largestRowElement` that returns a `String` and has the following parameters:
1. **A two-dimensional array** of type integer.
2. **An integer** that represents the number of the row to be processed.
#### Method Functionality
This method will find the largest element in the specified row and return a `String` containing the location and value of the largest element.
**Sample Output:**
```
Largest element of row 0 is 25 in column 1
```
### Method Signature Example
```java
public String largestRowElement(int[][] array, int rowNum) {
// Method implementation goes here
}
```
**Explanation:**
- `int[][] array`: Represents the two-dimensional integer array.
- `int rowNum`: Represents the row number to be processed for finding the largest element.
- The method will identify the largest element in the specified row, determine its column index, and return this information in a formatted string.
### Example Usage of the Method
```java
public class Main {
public static void main(String[] args) {
int[][] array = {
{10, 25, 3},
{7, 18, 5},
{14, 6, 30}
};
Main obj = new Main();
String result = obj.largestRowElement(array, 0);
System.out.println(result); // Output: Largest element of row 0 is 25 in column 1
}
public String largestRowElement(int[][] array, int rowNum) {
int largestElement = array[rowNum][0];
int columnIndex = 0;
for (int i = 1; i < array[rowNum].length; i++) {
if (array[rowNum][i] > largestElement) {
largestElement = array[rowNum][i];
columnIndex = i;
}
}
return "Largest element of row " + rowNum + " is " + largestElement + " in column " + columnIndex;
}
}
```
In this implementation:
- We initialize `largestElement` to the first element in the specified row.
- We iterate through the row to find the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
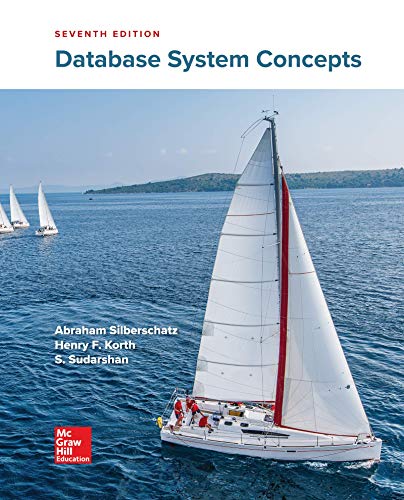
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
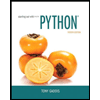
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
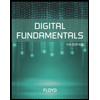
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
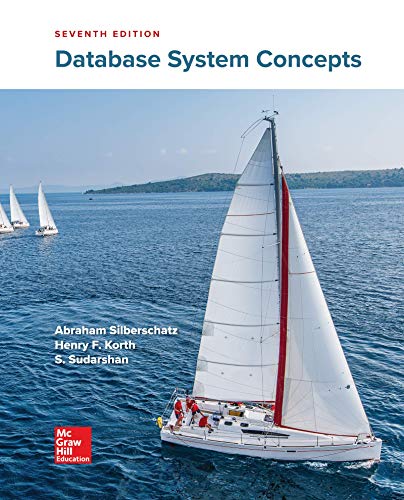
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
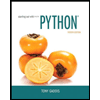
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
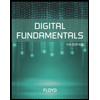
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
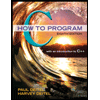
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
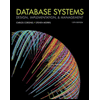
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
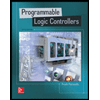
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education