As part of problem C, we're going to randomly select words to create sentences. However, we don't want it to be uniformly random. We will want to influence the random selection by having some words be more likely to appear than others. This is called weighted random selection. In this problem, we will build towards that goal by creating a function that does a weighted random selection from the keys of a dictionary, using the values as the weights. Create a function weighted_choice that takes as a parameter a dictionary of words and their corresponding counts. The function should randomly choose one of the words, using the counts as weights in the selection. For example, if I pass the dictionary {'green': 1, 'eggs': 1} To this function, it should return "green" half the time and "eggs" half the time. If I pass the dictionary {'green': 1, 'eggs': 3, 'ham': 2} It should return "green" one-sixth of the time, "eggs" half (3/6) of the time, and “ham” one-third (2/6) of the time. There are a number of ways to accomplish this task. Many solutions require you to iterate over the given dictionary to add up the counts to get the total of the counts before using a function from the random module to get a random number in the range from 1 to the total. Then, you can use that number to figure out which word it corresponds to and return the appropriate word. There's also a simple method that utilizes making a list with x copies of each key (where x is the value for said key), and then using random.choice. Examples (random, so won't necessarily match, but make sure that both come up about 50% of the time): >>> weighted_choice({'green': 1, 'eggs': 1}) 'green' >>> weighted_choice({'green': 1, 'eggs': 1}) 'eggs' >>> weighted_choice({ 'green': 1, 'eggs': 1}) 'eggs' 1
As part of problem C, we're going to randomly select words to create sentences. However, we don't want it to be uniformly random. We will want to influence the random selection by having some words be more likely to appear than others. This is called weighted random selection. In this problem, we will build towards that goal by creating a function that does a weighted random selection from the keys of a dictionary, using the values as the weights. Create a function weighted_choice that takes as a parameter a dictionary of words and their corresponding counts. The function should randomly choose one of the words, using the counts as weights in the selection. For example, if I pass the dictionary {'green': 1, 'eggs': 1} To this function, it should return "green" half the time and "eggs" half the time. If I pass the dictionary {'green': 1, 'eggs': 3, 'ham': 2} It should return "green" one-sixth of the time, "eggs" half (3/6) of the time, and “ham” one-third (2/6) of the time. There are a number of ways to accomplish this task. Many solutions require you to iterate over the given dictionary to add up the counts to get the total of the counts before using a function from the random module to get a random number in the range from 1 to the total. Then, you can use that number to figure out which word it corresponds to and return the appropriate word. There's also a simple method that utilizes making a list with x copies of each key (where x is the value for said key), and then using random.choice. Examples (random, so won't necessarily match, but make sure that both come up about 50% of the time): >>> weighted_choice({'green': 1, 'eggs': 1}) 'green' >>> weighted_choice({'green': 1, 'eggs': 1}) 'eggs' >>> weighted_choice({ 'green': 1, 'eggs': 1}) 'eggs' 1
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
In python

Transcribed Image Text:As part of problem C, we're going to randomly select
words to create sentences. However, we don't want it to
be uniformly random. We will want to influence the
random selection by having some words be more likely to
appear than others. This is called weighted random
selection. In this problem, we will build towards that goal
by creating a function that does a weighted random
selection from the keys of a dictionary, using the values
as the weights.
Create a function weighted_choice that takes as a
parameter a dictionary of words and their corresponding
counts. The function should randomly choose one of the
words, using the counts as weights in the selection. For
example, if I pass the dictionary
{'green': 1, 'eggs': 1}
To this function, it should return "green" half the time and
"eggs" half the time. If I pass the dictionary
{'green': 1, 'eggs': 3, 'ham': 2}
It should return "green" one-sixth of the time, "eggs" half
(3/6) of the time, and “ham” one-third (2/6) of the time.
There are a number of ways to accomplish this task.
Many solutions require you to iterate over the given
dictionary to add up the counts to get the total of the
counts before using a function from the random module
to get a random number in the range from 1 to the total.
hen, you can use that number to figure out which word
it corresponds to and return the appropriate word.
There's also a simple method that utilizes making a list
with x copies of each key (where x is the value for said
key), and then using random.choice.
Examples (random, so won't necessarily match, but
make sure that both come up about 50% of the time):
>>> weighted_choice({'green': 1, 'eggs':
1})
'green'
>>> weighted_choice({'green': 1, 'eggs':
1})
'eggs'
>>> weighted_choice({'green': 1, 'eggs':
1})
'eggs'
![Note that testing this function is a little tricky because it
relies on randomness. You will not see the same output
every time! We can control for this a bit by running the
function many times, to ensure that on average, the
probabilities roughly match the outcome. For example, if
we run
weighted_choice({'green': 1, 'eggs': 3,
'ham': 2})
600 times, then we would expect to get 'green' about 100
times, 'eggs' about 300 times, and 'ham' about 200 times.
[weighted_choice({'green':
>>> results =
1, 'eggs': 3, 'ham':2}) for i in
range (600)]
>>> results.count('green')
99
>>> results.count('eggs')
296
>>> results.count('ham')
205
If your results are consistently off by more than 20 or so,
you should probably check your algorithm.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F77fc5231-144e-4658-a4e4-843a776de72b%2F3d1b2c3f-494a-449d-be55-1e42216d1dd5%2F1k8rcsg_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Note that testing this function is a little tricky because it
relies on randomness. You will not see the same output
every time! We can control for this a bit by running the
function many times, to ensure that on average, the
probabilities roughly match the outcome. For example, if
we run
weighted_choice({'green': 1, 'eggs': 3,
'ham': 2})
600 times, then we would expect to get 'green' about 100
times, 'eggs' about 300 times, and 'ham' about 200 times.
[weighted_choice({'green':
>>> results =
1, 'eggs': 3, 'ham':2}) for i in
range (600)]
>>> results.count('green')
99
>>> results.count('eggs')
296
>>> results.count('ham')
205
If your results are consistently off by more than 20 or so,
you should probably check your algorithm.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
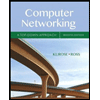
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
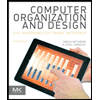
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
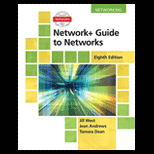
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
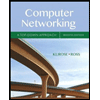
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
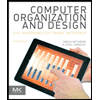
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
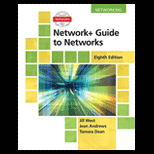
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
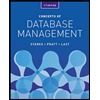
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
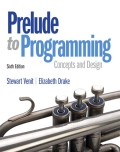
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
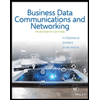
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY