Answer each question given this definition of a linked list. struct ListNode { // a data type double value; ListNode *next; }; ListNode *head; Write C++ statements to output the sum of the numbers in the list. Write C++ statements to output the value of the second-to-last node (the one preceding the last node). You may assume the list contains at least two nodes (maybe more). Given the following list, write C++ statements to remove the node containing 3.2 (and deallocate it). Assume p is of type ListNode * and is already pointing to 1.2. You may declare and use another pointer, but do not use a loop, do not change p, do not use head. head -> 1.2 -> 3.2 -> 7.7 -> null The template code below has already constructed this list. #include using namespace std; struct ListNode // the node data type { double value; // data ListNode *next; // ptr to next node }; int main() { //set up a list with 3 elements (done): ListNode *head = NULL; ListNode *newNode = new ListNode; newNode->value = 7.7; newNode->next = head; //NULL at this point head = newNode; newNode = new ListNode; newNode->value = 3.2; newNode->next = head; head = newNode; newNode = new ListNode; newNode->value = 1.2; newNode->next = head; head = newNode; //write c++ statements to output the sum of the numbers in the list double sum; /* add your code here for #1 */ cout << "The sum is: " << sum << endl << endl; //Write C++ statements to output the value of the second-to-last node double secondToLast; /* add your code here for #2 */ cout << "The value of the second-to-last node is: " << secondToLast << endl; cout << endl; //Write c++ statements to delete the node containing 3.2 //You may declare and use another pointer variable, but do not use a loop, // do not change p1, do not use head in your code ListNode *p1 = head; // now p is pointing to the 1.2 /* add your code here for #3 */ //display after the delete ListNode *ptr = head; while (ptr) { cout << ptr->value << " "; ptr = ptr->next; } cout << endl; }
Answer each question given this definition of a linked list.
struct ListNode { // a data type double value; ListNode *next; }; ListNode *head;-
Write C++ statements to output the sum of the numbers in the list.
-
Write C++ statements to output the value of the second-to-last node (the one preceding the last node). You may assume the list contains at least two nodes (maybe more).
-
Given the following list, write C++ statements to remove the node containing 3.2 (and deallocate it). Assume p is of type ListNode * and is already pointing to 1.2. You may declare and use another pointer, but do not use a loop, do not change p, do not use head.
The template code below has already constructed this list.
#include <iostream>
using namespace std;
struct ListNode // the node data type
{
double value; // data
ListNode *next; // ptr to next node
};
int main() {
//set up a list with 3 elements (done):
ListNode *head = NULL;
ListNode *newNode = new ListNode;
newNode->value = 7.7;
newNode->next = head; //NULL at this point
head = newNode;
newNode = new ListNode;
newNode->value = 3.2;
newNode->next = head;
head = newNode;
newNode = new ListNode;
newNode->value = 1.2;
newNode->next = head;
head = newNode;
//write c++ statements to output the sum of the numbers in the list
double sum;
/* add your code here for #1 */
cout << "The sum is: " << sum << endl << endl;
//Write C++ statements to output the value of the second-to-last node
double secondToLast;
/* add your code here for #2 */
cout << "The value of the second-to-last node is: " << secondToLast << endl;
cout << endl;
//Write c++ statements to delete the node containing 3.2
//You may declare and use another pointer variable, but do not use a loop,
// do not change p1, do not use head in your code
ListNode *p1 = head; // now p is pointing to the 1.2
/* add your code here for #3 */
//display after the delete
ListNode *ptr = head;
while (ptr) {
cout << ptr->value << " ";
ptr = ptr->next;
}
cout << endl;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

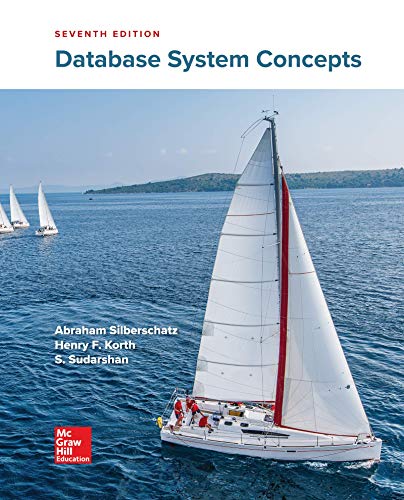
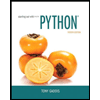
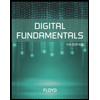
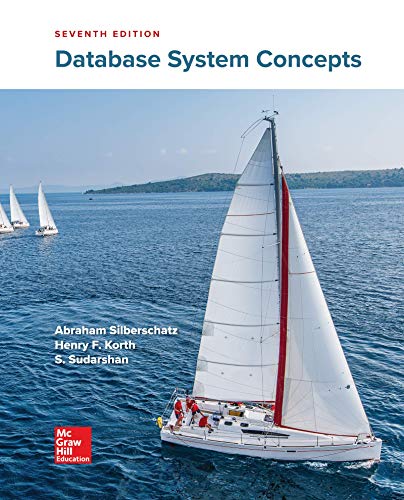
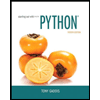
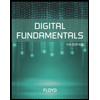
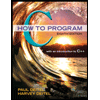
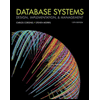
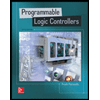