Analyze the below code and answer the questions:
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.
Analyze the below code and answer the questions:
#include <stdio.h>
void add10 (int A[],int m);
int main(void)
{
int Array2 [5]={11,21,31,41,51};
add10(Array2,5);
for (int i=0;i<5; i++)
{
printf("Array2 [%d] after modification = %d \n", i,Array2 [i]);
}
return 0;
}
void add10 (int A[],int m){
for (int a = 0;a < m; a++)
{
A[a] = A[a]+10;
}
return;
}
- Explain what does this code do?
- What is the size of Array2 [] ?
- What is the Array2 [4] value after the modification ?
- Change the size of Array2 to be 7 elements {……61,71}?

Step by step
Solved in 4 steps with 1 images

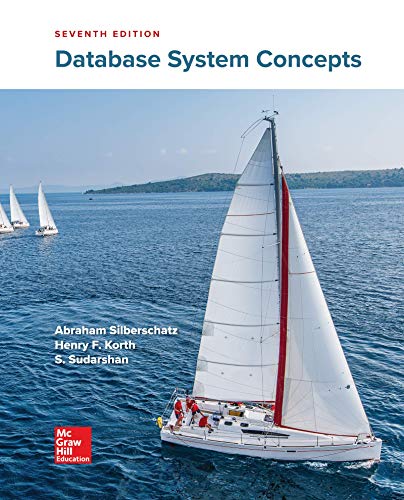
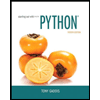
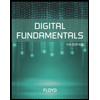
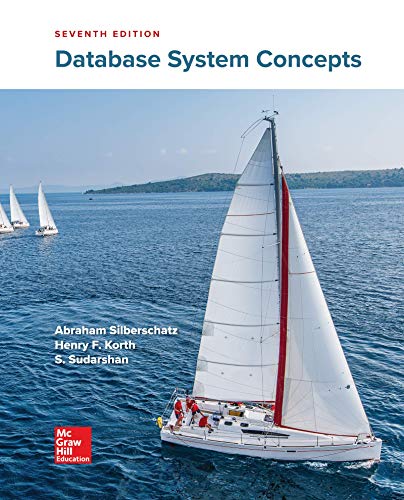
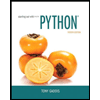
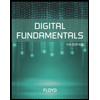
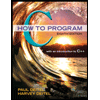
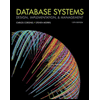
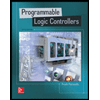