An acronym is a word formed from the initial letters of words in a set phrase. Write a program whose input is a 4 word phrase and whose output is an acronym of the input. If a word begins with a lower case letter, don't include that letter in the acronym. Assume there will be at least one upper case letter in the input. Ex: If the input is: Association for Computing Machinery the output should is: ACM Your program must define and call a function thats returns the acronym created for the given userPhrase. string CreateAcronym (string wordl, string word2, string word3, string word4) Hint 1: Use isupper() to check if a letter is upper case. isUpper(ch) returns true if ch is a char variable containing an uppercase letter (A"..Z'), otherwise it returns false. You can use it in the if condition. Hint 2: Use str.append(1, ch) to append a character in char variable ch to the end of the string str. The 1 tells the function to append exactly 1 character. Hint 3: Do not use getline.
An acronym is a word formed from the initial letters of words in a set phrase. Write a program whose input is a 4 word phrase and whose output is an acronym of the input. If a word begins with a lower case letter, don't include that letter in the acronym. Assume there will be at least one upper case letter in the input. Ex: If the input is: Association for Computing Machinery the output should is: ACM Your program must define and call a function thats returns the acronym created for the given userPhrase. string CreateAcronym (string wordl, string word2, string word3, string word4) Hint 1: Use isupper() to check if a letter is upper case. isUpper(ch) returns true if ch is a char variable containing an uppercase letter (A"..Z'), otherwise it returns false. You can use it in the if condition. Hint 2: Use str.append(1, ch) to append a character in char variable ch to the end of the string str. The 1 tells the function to append exactly 1 character. Hint 3: Do not use getline.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C++

Transcribed Image Text:5.17 LAB: Acronyms
An acronym is a word formed from the initial letters of words in a set phrase. Write a program whose input is a 4-word phrase and whose output is an acronym of the input. If a word begins with a lowercase letter, don't include that letter in the acronym. Assume there will be at least one uppercase letter in the input.
Example: If the input is:
Association for Computing Machinery
the output should be:
ACM
Your program must define and call a function that returns the acronym created for the given userPhrase.
```cpp
string CreateAcronym(string word1, string word2, string word3, string word4)
```
Hints:
1. Use `isupper()` to check if a letter is uppercase. `isupper(ch)` returns true if `ch` is a char variable containing an uppercase letter ('A'..'Z'), otherwise it returns false. You can use it in the if condition.
2. Use `str.append(1, ch)` to append a character in char variable `ch` to the end of the string `str`. The `1` tells the function to append exactly 1 character.
3. Do **not** use `getline`.
---
### Lab Activity: Code Template
```cpp
#include <iostream>
using namespace std;
/* Define your function here */
```
(Note: The code segment above serves as a template for writing your program. Replace comments with actual code.)

Transcribed Image Text:### Educational Content on C++ Programming: Creating Acronyms
#### Hints for the Lab Activity:
1. **Hint 2**: Use `str.append(1, ch)` to append a character in the char variable `ch` to the end of the string `str`. The `1` specifies that the function should append exactly one character.
2. **Hint 3**: Avoid using `getline`.
---
#### Lab Activity: 5.17.1 LAB: Acronyms
This section involves writing a C++ program to create acronyms using given words. Below is the skeleton code you will be working with:
```cpp
#include <iostream>
using namespace std;
/* Define your function here */
int main() {
string word1;
string word2;
string word3;
string word4;
/* Type your code here */
return 0;
}
```
- **Lines 1-2**: The `#include <iostream>` directive includes the Input/Output stream library for standard I/O operations. The `using namespace std;` line allows you to use elements from the standard library without prefixing `std::`.
- **Lines 5-13**: This is where you will define your main function and begin writing your code to generate acronyms from strings entered by the user.
#### Developing and Submitting Code:
- **Develop Mode**: Allows you to write and test your code. You can run the program as many times as needed to ensure accuracy before submitting.
- **Enter Program Input (Optional)**: If your code requires input values, you can specify them here.
- **Run and Submit**: After entering any necessary inputs, click on "Run program" to observe the output. When satisfied, switch to "Submit mode" for grading.
---
This activity is designed to enhance your understanding of string manipulation in C++ by using functions like `str.append()`. It provides a practical way to reinforce your learning through hands-on experience.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
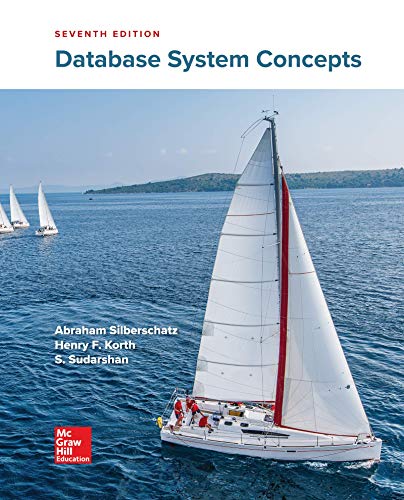
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
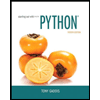
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
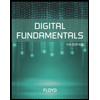
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
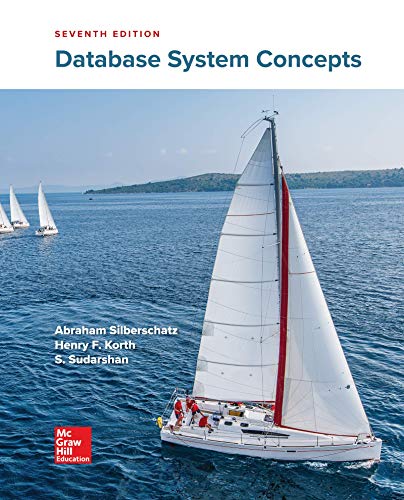
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
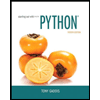
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
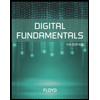
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
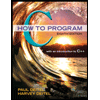
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
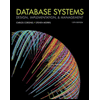
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
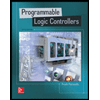
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education