am attempting to create 3 individual plots A plot of HARPS-N CCF data. This data is freely available on the DACE website. I have been able to create this plot and it produces a signature "V" shape. A plot of the Mean of the CCF data, same shape. A residuals plot. This is where I am having the difficulty, I have coded as much can. The shape of the plot should be "dumbbell" in shape. At the moment I am getting an empty plot. This is the code that I have so far.. #import libs import matplotlib.pyplot as plt import glob from astropy.io import fits from astropy.wcs import WCS import numpy as np # Initial plot fig, ax = plt.subplots(figsize=(10, 10)) # data from the FITS data_dir = glob.glob('/Users/xxxxx/Desktop/harpn_sun_release_package_ccf_2018/2018-01-18/*.fits') # empty lists for storage lams = [] fluxs = [] # read the the FITS for file_path in data_dir: hdul = fits.open(file_path) data = hdul[1].data h1 = hdul[1].header flux = data[1] w = WCS(h1, naxis=1, relax=False, fix=False) lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0] lams.append(lam) fluxs.append(flux) ax.plot(lam, flux) # Calc mean flux mean_ccf = np.mean(fluxs, axis=0) # New fig for main plot fig_mean, ax_mean = plt.subplots(figsize=(10, 10)) # plot the mean data ax_mean.plot(lams[1], mean_ccf, color='k', linewidth=1) # Mean ax_mean.set_xlabel('RV [km/s]') ax_mean.set_ylabel('Normalized CCF') ax_mean.set_title('Mean CCF') # Residuals residuals = fluxs - mean_ccf fig_res, ax_res = plt.subplots(figsize=(10, 10)) ax_res.plot(lams[1], residuals, color='k', linewidth=1) # Plot residuals ax_res.set_xlabel('RV [km/s]') ax_res.set_ylabel('CCF Residuals') ax_res.set_title('CCF Residuals') plt.show()
am attempting to create 3 individual plots
-
A plot of HARPS-N CCF data. This data is freely available on the DACE website. I have been able to create this plot and it produces a signature "V" shape.
-
A plot of the Mean of the CCF data, same shape.
-
A residuals plot. This is where I am having the difficulty, I have coded as much can. The shape of the plot should be "dumbbell" in shape. At the moment I am getting an empty plot.
This is the code that I have so far..
#import libs
import matplotlib.pyplot as plt
import glob
from astropy.io import fits
from astropy.wcs import WCS
import numpy as np
# Initial plot
fig, ax = plt.subplots(figsize=(10, 10))
# data from the FITS
data_dir = glob.glob('/Users/xxxxx/Desktop/harpn_sun_release_package_ccf_2018/2018-01-18/*.fits')
# empty lists for storage
lams = []
fluxs = []
# read the the FITS
for file_path in data_dir:
hdul = fits.open(file_path)
data = hdul[1].data
h1 = hdul[1].header
flux = data[1]
w = WCS(h1, naxis=1, relax=False, fix=False)
lam = w.wcs_pix2world(np.arange(len(flux)), 0)[0]
lams.append(lam)
fluxs.append(flux)
ax.plot(lam, flux)
# Calc mean flux
mean_ccf = np.mean(fluxs, axis=0)
# New fig for main plot
fig_mean, ax_mean = plt.subplots(figsize=(10, 10))
# plot the mean data
ax_mean.plot(lams[1], mean_ccf, color='k', linewidth=1)
# Mean
ax_mean.set_xlabel('RV [km/s]')
ax_mean.set_ylabel('Normalized CCF')
ax_mean.set_title('Mean CCF')
# Residuals
residuals = fluxs - mean_ccf
fig_res, ax_res = plt.subplots(figsize=(10, 10))
ax_res.plot(lams[1], residuals, color='k', linewidth=1)
# Plot residuals
ax_res.set_xlabel('RV [km/s]')
ax_res.set_ylabel('CCF Residuals')
ax_res.set_title('CCF Residuals')
plt.show()

Step by step
Solved in 3 steps

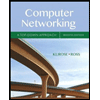
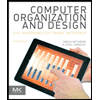
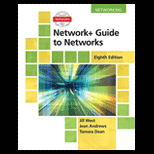
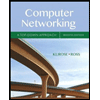
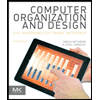
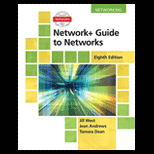
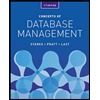
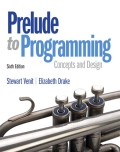
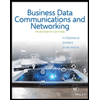