Add Boolean instance variables to indicate whether a node pointer is a thread or regular pointer. You may not add any additional pointers to BSTNode. The whole idea of threads is that they take advantage of unused BSTNode pointers and thereby reduce the binary tree’s wasted overhead. Add setter/getter methods to access the Boolean instance variables or modify existing setters/getters as necessary.
Add Boolean instance variables to indicate whether a node pointer is a thread or regular pointer. You may not add any additional pointers to BSTNode. The whole idea of threads is that they take advantage of unused BSTNode pointers and thereby reduce the binary tree’s wasted overhead. Add setter/getter methods to access the Boolean instance variables or modify existing setters/getters as necessary.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
- Add Boolean instance variables to indicate whether a node pointer is a thread or regular pointer. You may not add any additional pointers to BSTNode. The whole idea of threads is that they take advantage of unused BSTNode pointers and thereby reduce the binary tree’s wasted overhead.
- Add setter/getter methods to access the Boolean instance variables or modify existing setters/getters as necessary.

Transcribed Image Text:9
#include "book.h"
10
#include "BinNode.h"
11
12
#ifndef BSTNODE H
13
#define BSTNODE H
14
// Simple binary tree node implementation
template
15
16
<typename Key, typename E>
17 eclass BSTNode : public BinNode<E> {
18
private:
Кey k;
E it;
BSTNode* lc;
// The node's key
// The node's value
// Pointer to left child
// Pointer to right child
19
20
21
22
BSTNode* rc;
23
public:
// Two constructors
24
25
with and without initial values
26
BSTNode ()
{ lc =
rc = NULL; }
27
BSTNode (Key K, E e, BSTNode* 1 =NULL, BSTNode* r =NULL)
28
{ k = K; it = e; lc = 1; rc = r; }
29
~BSTNode () {}
// Destructor
30
// Functions to set and return the value and key
E& element () { return it; }
31
32
33
void setElement(const E& e)
{ it = e; }
Key& key(O { return k; }
void setKey(const Key& K)
34
35
{ k = K; }
36
// Functions to set and return the children
inline BSTNode* left() const { return lc; }
void setLeft(BinNode<E>* b)
37
38
39
{ lc =
(BSTNode*)b; }
inline BSTNode* right() const { return rc; }
void setRight(BinNode<E>* b) { rc =
40
41
(BSTNode*) b; }
42
// Return true if it is a leaf, false otherwise
bool isLeaf()
43
44
{ return (lc
NULL) &&
(rc
== NULL); }
==
45
} ;
46
47
#endif
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
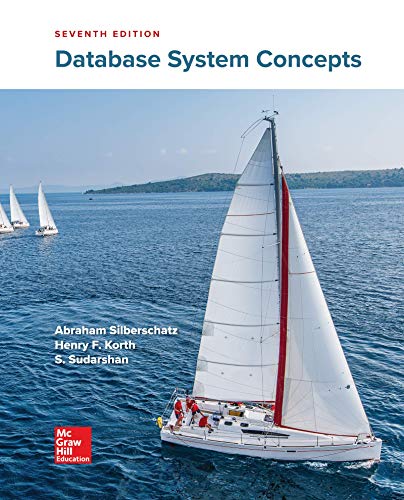
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
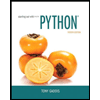
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
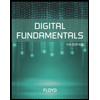
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
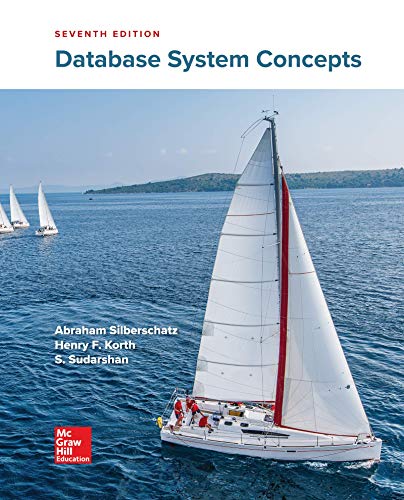
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
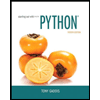
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
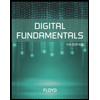
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
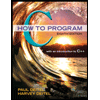
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
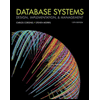
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
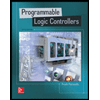
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education