--- About initial programming in python --- Make a procedure called many_times() that simulates a game of two dice. Your procedure should count how many times the dice were rolled until repeated numbers came out. (To throw two dice count as a single throw.) What should your procedure return? Should return the number of throws made and the two [equal] numbers chosen --- put the three values into a tuple. Suppose your dice has 6 sides. Use the randint procedure of the random module to simulate the throw of a die. Documents for this procedure can be found at https://cutt.ly/dWsa2Rl. Note that there are restrictions on input values. Use randint, tuples and while to solve the problem. REMEMBER THAT THE THIRD ELEMENT IN THE TUPLE (THE NUMBER OF THROWS MADE) CANNOT BE 0 - Below is a print of my code that you should use as a basis to solve the challenge. My problem is that the third element of my tuple (number of dice rolls until repeated numbers appear) is returning 0 in some situations which is obviously wrong.
--- About initial programming in python ---
Make a procedure called many_times() that simulates
a game of two dice. Your procedure should count how many times
the dice were rolled until repeated numbers came out. (To throw
two dice count as a single throw.) What should your procedure return? Should return the number of throws made and the two [equal] numbers chosen --- put the three values into a tuple. Suppose your dice has 6 sides. Use the randint procedure of the random module to simulate the throw of a die. Documents for this procedure can be found at https://cutt.ly/dWsa2Rl. Note that there are restrictions on input values.
Use randint, tuples and while to solve the problem.
REMEMBER THAT THE THIRD ELEMENT IN THE TUPLE (THE NUMBER OF THROWS MADE) CANNOT BE 0
- Below is a print of my code that you should use as a basis to solve the challenge. My problem is that the third element of my tuple (number of dice rolls until repeated numbers appear) is returning 0 in some situations which is obviously wrong.


I have implemented the given requirements as above.
The issue is that there is no check for the value of count. There should be an "if" condition which checks if the value of count is greater than 0. Only in that case it will return the tuple.
The case where count is 0 is when the two dice rolled repeated number in the first throw which is to be ignored.
Code is as follows:
Step by step
Solved in 3 steps with 2 images

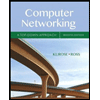
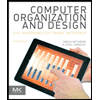
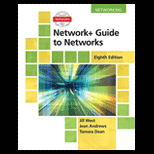
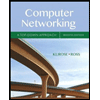
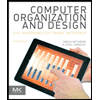
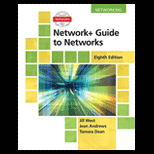
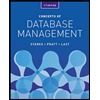
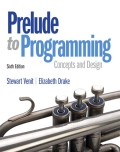
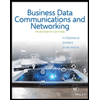