A1.2 Bean Machine The bean machine is a device for statistical experiments. It consists of an upright board with evenly spaced nails (or pegs) in a triangular form,. Balls are dropped from the opening at the top of the board. Every time a ball hits a nail, it has a 50% chance of falling to the left or to the right. The piles of balls are accumulated in the slots at the bottom of the board. Write a program to simulate the bean machine that has 8 slots as shown in the figure. Your program should prompt the user to enter the number of balls to drop. Simulate the falling of each ball by printing its path. For example, the path for the ball in LLRRLLR and the path for the ball in RLRRLRR. Note that there are 7 levels of nails, so your path should be 7 letters (not 8). Create an array called slots. Each element in slots store the number of balls in a slot. Each ball falls into a slot via a path. The number of “R”s in a path is the position of the slot where the ball falls. For example, for the path LRLRLRR, the ball falls into slots[4] and for the path RRLLLLL, the ball falls into slots[2]. You should create a method to randomly drop a ball and return the position in the array that the ball dropped into. You will pass in the number of slots as that will determine how many levels of nails the ball must pass through (numberOfSlots-1). This method should also print out the path that the ball took (e.g. LRLLRLL). Here is the suggested method header: public static int dropBall(int numberOfSlots) - Generate the path the ball took using the random method from the Math class - Print the path on the screen - Return the slot number the ball fell into INFO 2313 Assignment #1 Page 4 of 5 Display the final buildup of the balls in the slots using a histogram. Create a method with the following header: public static void printGameResults(int[] slots) Below is a sample run of my program using 10 balls and the desired output. Note that the slot number displayed is +1 more than the array index which is zero-based. In this example, 6 balls fell into slot 3 (index 2 in the array, the path would have two “R”s and five “L”s). Finally, utilize a do-while loop to allow the user to try the program again (without having to re-run the program from your IDE), displaying the following message after displaying the results of the game: // Ask user if they want to play again System.out.print("\nDo you want to play again (y/n)? ");
A1.2 Bean Machine
The bean machine is a device for statistical experiments. It consists of an upright board with evenly spaced nails (or pegs) in a triangular form,.
Balls are dropped from the opening at the top of the board. Every time a ball hits a nail, it has a 50% chance of falling to the left or to the right. The piles of balls are accumulated in the slots at the bottom of the board.
Write a
Create an array called slots. Each element in slots store the number of balls in a slot. Each ball falls into a slot via a path. The number of “R”s in a path is the position of the slot where the ball falls. For example, for the path LRLRLRR, the ball falls into slots[4] and for the path RRLLLLL, the ball falls into slots[2].
You should create a method to randomly drop a ball and return the position in the array that the ball dropped into. You will pass in the number of slots as that will determine how many levels of nails the ball must pass through (numberOfSlots-1). This method should also print out the path that the ball took (e.g. LRLLRLL). Here is the suggested method header:
public static int dropBall(int numberOfSlots)
- Generate the path the ball took using the random method from the Math class
- Print the path on the screen
- Return the slot number the ball fell into
INFO 2313 Assignment #1 Page 4 of 5
Display the final buildup of the balls in the slots using a histogram. Create a method with the following header:
public static void printGameResults(int[] slots)
Below is a sample run of my program using 10 balls and the desired output. Note that the slot number displayed is +1 more than the array index which is zero-based. In this example, 6 balls fell into slot 3 (index 2 in the array, the path would have two “R”s and five “L”s).
Finally, utilize a do-while loop to allow the user to try the program again (without having to re-run the program from your IDE), displaying the following message after displaying the results of the game:
// Ask user if they want to play again System.out.print("\nDo you want to play again (y/n)? ");

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

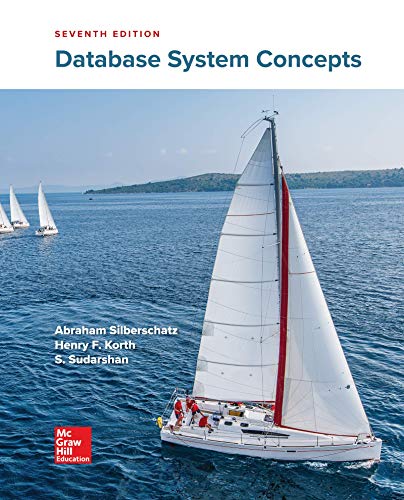
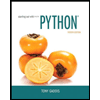
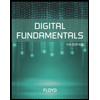
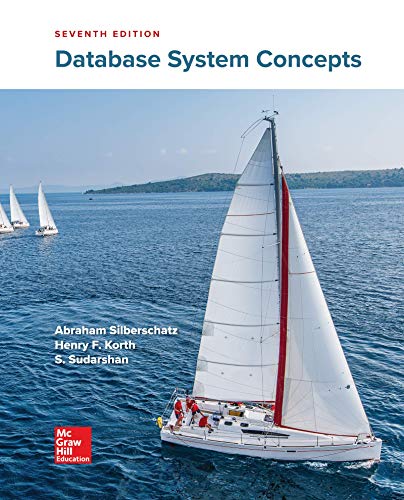
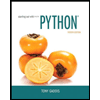
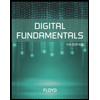
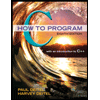
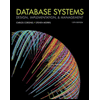
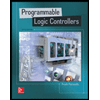