(a) Write the static method numberOfLeapYears, which returns the number of leap years between year1 and year2, inclusive. In order to calculate this value, a helper method is provided for you. isLeapYear(year) returns true if year is a leap year and false otherwise. Complete method numberOfLeapYears below. You must use isLeapYear appropriately to receive full credit. /** Returns the number of leap years between year1 and year2, inclusive. * Precondition: 0 <= year1 <= year2 */ public static int numberOfLeapYears(int year1, int year2) ================================== public class APCalendar { private static boolean isLeapYear(int year) { if (year % 4 == 0) { return true; } return false; } public static int numberOfLeapYears(int year1, int year2) { int leapYears = 0; for(int y = year1; y <= year2; y++) if(isLeapYear(y)) leapYears++; return leapYears; } } ============ public class APCalendarRunner { public static void main(String[] args) { APCalendar ap = new APCalendar(); //Test-1 System.out.println(ap.numberOfLeapYears(1900, 2000)); //Test-2 System.out.println(ap.numberOfLeapYears(1801, 1850)); //Test-3 System.out.println(ap.numberOfLeapYears(2010, 2020)); //Test-4 System.out.println(ap.numberOfLeapYears(1850, 2000)); } }
(a) Write the static method numberOfLeapYears, which returns the number of leap years between year1 and year2, inclusive.
In order to calculate this value, a helper method is provided for you.
- isLeapYear(year) returns true if year is a leap year and false otherwise.
Complete method numberOfLeapYears below. You must use isLeapYear appropriately to receive full credit.
/** Returns the number of leap years between year1 and year2, inclusive. * Precondition: 0 <= year1 <= year2 */ public static int numberOfLeapYears(int year1, int year2)
==================================
public class APCalendar
{
private static boolean isLeapYear(int year)
{
if (year % 4 == 0)
{
return true;
}
return false;
}
public static int numberOfLeapYears(int year1, int year2)
{
int leapYears = 0;
for(int y = year1; y <= year2; y++)
if(isLeapYear(y))
leapYears++;
return leapYears;
}
}
============
public class APCalendarRunner {
public static void main(String[] args) {
APCalendar ap = new APCalendar();
//Test-1
System.out.println(ap.numberOfLeapYears(1900, 2000));
//Test-2
System.out.println(ap.numberOfLeapYears(1801, 1850));
//Test-3
System.out.println(ap.numberOfLeapYears(2010, 2020));
//Test-4
System.out.println(ap.numberOfLeapYears(1850, 2000));
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

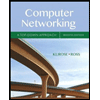
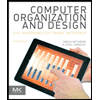
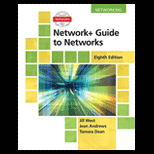
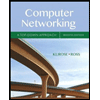
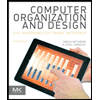
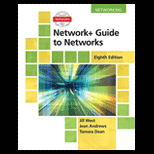
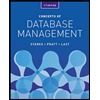
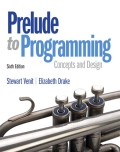
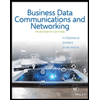