A prime number is an integer number greater than 1 that has no positive divisors other than one and itself. The table below are examples of some primes numbers. First 100 Prime Numbers 2 31 73 127 179 233 *** 283 293 3 37 79 131 181 5 7 13 17 19 53 59 61 89 97 101 103 107 139 149 151 157 163 193 197 199 211 263 269 ** 317 331 43 11 47 431 433 439 487 491 499 83 137 191 223 241 239 251 257 271 257 313 *** 311 337 379 383 397 401 307 353 359 367 373 419 421 467 479 443 457 461 503 509 521 523 Accept This Assignment B Purpose 399 449 This assignment has the following purposes: . Practice analyzing a problem . Practice implementing a solution in Python 23 67 109 Enter an integer to test: 101 101 is a prime number Do you want to test another integer (y/n): y Enter an integer to test: $#y6@ Invalid integer value! Try again 167 227 277 40 347 Assignment Description Write a program that will prompt the user for an integer n that will be tested to determine if it is a prime number. The best approach is to start by assuming the integer n is a prime number (isPrime=True) and attempting to prove it is not prime by testing it to find a number i that divides it evenly using the modulo (remainder) operator (i.e. n%i==0). 29 113 173 229 . Practice using iteration structures to create repeated decision structures to control program execution flow • Practice using try-except statement to handle exceptions caused by ValueError Solution Requirements and Approach Use a loop (a for loop is easiest) to repeatedly test the integer n to see if an i=2. 3. 4. 5.... etc. up to n-1, is a divisor of the integer. If you find a number that divides n evenly then you have proven that the number is not prime (isPrime=False) and the program breaks from the loop. If a divisor is found to prove that the integer n is not prime, then output the smallest number that evenly divides the integer n. If all possibilities are exhausted and no divisor was found that evenly divides integer n then the number must be prime (isPrime==True). 281 349 409 463 541 Your program should also handle ValueErrors caused by invalid data entered by the user. When a ValueError occurs you can use the continue statement to return to the top of the while loop containing the input prompt collecting the test value from the user. The program should also check with the user to see if they wish to test another number. If yes, then the program should clear the screen and then prompt the user for the number to test. If they answer no then the program should terminate. Sample Output Prime Number Checker Enter an integer to test: 25 25 is a composite number because it is divisible by 5 Do you want to test another integer (y/n): y Enter an integer to test: 10013 10013 is a composite number because it is divisible by 17 Do you want to test another integer (y/n): n Bye!
A prime number is an integer number greater than 1 that has no positive divisors other than one and itself. The table below are examples of some primes numbers. First 100 Prime Numbers 2 31 73 127 179 233 *** 283 293 3 37 79 131 181 5 7 13 17 19 53 59 61 89 97 101 103 107 139 149 151 157 163 193 197 199 211 263 269 ** 317 331 43 11 47 431 433 439 487 491 499 83 137 191 223 241 239 251 257 271 257 313 *** 311 337 379 383 397 401 307 353 359 367 373 419 421 467 479 443 457 461 503 509 521 523 Accept This Assignment B Purpose 399 449 This assignment has the following purposes: . Practice analyzing a problem . Practice implementing a solution in Python 23 67 109 Enter an integer to test: 101 101 is a prime number Do you want to test another integer (y/n): y Enter an integer to test: $#y6@ Invalid integer value! Try again 167 227 277 40 347 Assignment Description Write a program that will prompt the user for an integer n that will be tested to determine if it is a prime number. The best approach is to start by assuming the integer n is a prime number (isPrime=True) and attempting to prove it is not prime by testing it to find a number i that divides it evenly using the modulo (remainder) operator (i.e. n%i==0). 29 113 173 229 . Practice using iteration structures to create repeated decision structures to control program execution flow • Practice using try-except statement to handle exceptions caused by ValueError Solution Requirements and Approach Use a loop (a for loop is easiest) to repeatedly test the integer n to see if an i=2. 3. 4. 5.... etc. up to n-1, is a divisor of the integer. If you find a number that divides n evenly then you have proven that the number is not prime (isPrime=False) and the program breaks from the loop. If a divisor is found to prove that the integer n is not prime, then output the smallest number that evenly divides the integer n. If all possibilities are exhausted and no divisor was found that evenly divides integer n then the number must be prime (isPrime==True). 281 349 409 463 541 Your program should also handle ValueErrors caused by invalid data entered by the user. When a ValueError occurs you can use the continue statement to return to the top of the while loop containing the input prompt collecting the test value from the user. The program should also check with the user to see if they wish to test another number. If yes, then the program should clear the screen and then prompt the user for the number to test. If they answer no then the program should terminate. Sample Output Prime Number Checker Enter an integer to test: 25 25 is a composite number because it is divisible by 5 Do you want to test another integer (y/n): y Enter an integer to test: 10013 10013 is a composite number because it is divisible by 17 Do you want to test another integer (y/n): n Bye!
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Need help and understanding on creating and starting the python/pycharm

Transcribed Image Text:# Prime Number Checker
## A prime number is an integer greater than 1 that has no positive divisors other than one and itself. The table below are examples of some prime numbers.
```
First 100 Prime Numbers
2 3 5 7 11 13 17 19 23 29
31 37 41 43 47 53 59 61 67 71
73 79 83 89 97 101 103 107 109 113
127 131 137 139 149 151 157 163 167 173
179 181 191 193 197 199 211 223 227 229
233 239 241 251 257 263 269 271 277 281
283 293 307 311 313 317 331 337 347 349
353 359 367 373 379 383 389 397 401 409
419 421 431 433 439 443 449 457 461 463
467 479 487 491 499 503 509 521 523 541
```
## Assignment Description
Write a program that will prompt the user for an integer *n* that will be tested to determine if it is a prime number. The best approach is to start by assuming the integer *n* is a prime number (`isPrime=True`) and attempting to prove it is not prime by testing it to find a number 1 that divides it evenly using the modulo (remainder) operator (i.e. `n%i==0`).
**Accept This Assignment**
## Purpose
This assignment has the following purposes:
- Practice analyzing a problem
- Practice implementing a solution in Python
- Practice using iteration structures to create repeated decision structures to control program execution flow
- Practice using try-except statement to handle exceptions caused by `ValueError`
## Solution Requirements and Approach
Use a loop (a for loop is easiest) to repeatedly test the integer *n* to see if any i = 2, 3, 4, 5, . . . etc. up to n-1, is a divisor of the integer. If you find a number that divides *n* evenly then you have proven that the number is not prime (`isPrime=False`) and the program breaks from the loop. If a divisor is
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
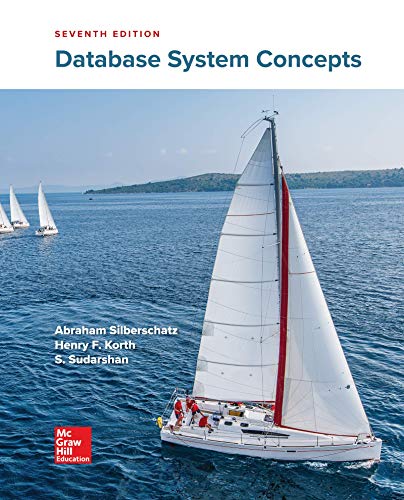
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
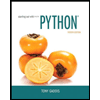
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
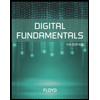
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
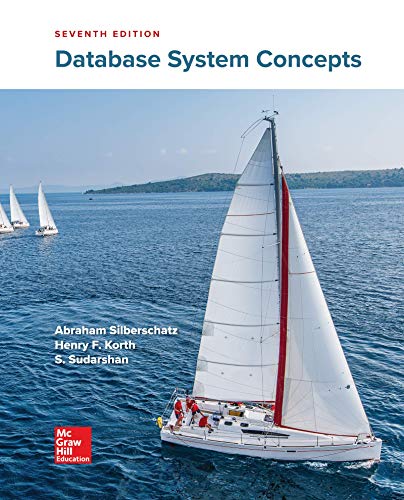
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
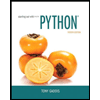
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
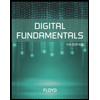
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
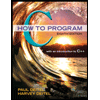
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
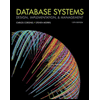
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
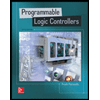
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education