A palindrome is a word or a phrase that is the same when read both forward and backward. Examples are: "bob," "sees," or "never odd or even" (ignoring spaces). Write a program whose input is a word or phrase, and that outputs whether the input is a palindrome.
Question:
A palindrome is a word or a phrase that is the same when read both forward and backward. Examples are: "bob," "sees," or "never odd or even" (ignoring spaces). Write a program whose input is a word or phrase, and that outputs whether the input is a palindrome.
Ex: If the input is:
bob
the output is:
palindrome: bob
Ex: If the input is:
bobby
the output is:
not a palindrome: bobby
Hint: Start by just handling single-word input, and submit for grading. Once passing single-word test cases, extend the program to handle phrases. If the input is a phrase, remove or ignore spaces.
My Issue:
I'm unable to use replaceAll(" ", "") as the java program wouldn't allow me as we're working on loops this week and haven't touch upon it. I've attached a screenshot where replaceAll method resulted in no output with the error displayed "Exception in thread "main" java.lang.StringIndexOutOfBoundsException: String index out of range: 16". Regardless,I don't know how to include the space in the string variable to include the entire sentence as a palindrone.
My code:
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
/* Type your code here. */String word="";
String opposite = "";
Scanner scnr= new Scanner(System.in);
word = scnr.nextLine();
for(int i = word.length() - 1; i >= 0; i--){
opposite = opposite + word.charAt(i);
}
if(word.equals(opposite)){
System.out.println(“palindrome: " +word);
}
else{
System.out.println(“not a palindrome: "+word);
}
}
}
My initial code with replaceAll:
String word = "";
String oppos = "";
Scanner scnr = new Scanner(System.in);
word = scnr.nextLine();
String str = word.replaceAll(" ","");
for(int i = word.length() - 1; i >= 0; i--){
oppos = oppos + str.charAt(i);
}
if(str.equals(oppos)){
System.out.println(“palindrome: " +word);
}
else{
System.out.println(“not a palindrome: "+word);
}
}
}



Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

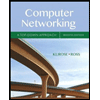
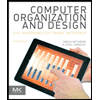
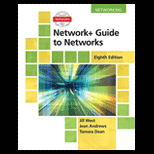
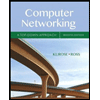
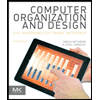
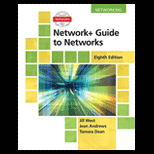
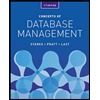
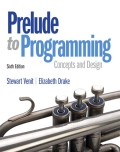
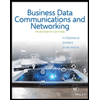