A palindrome is a sequence that is the same as its reverse. For example, the word "RACECAR" is a palindrome. For this problem we will define an almost palindrome to be a sequence that can be transformed into its reverse with 2 or fewer character additions, deletions, replacements, or swaps. A character swap swaps two adjacent characters. For instance "MARJORAM" can be reversed by swapping the 'J' and the 'O'. In addition, "FOOLPROOF" can be reversed by replacing the 'R' with and 'L' and vice versa. Finally, the word "BONOBO" can be reversed by deleting the final 'O' and reinserting it at the beginning. Devise a dynamic programming algorithm that will determine the number of edits necessary to to transform a sequence into its reverse. a) State this problem with formal input and output conditions. b) State a self-reduction for this problem. c) State a dynamic programming algorithm based off of your self-reduction that computes the minimum number of edits to transform a sequence into its reverse. d) Use your algorithm to determine the minimum number of edits to transform "MARJORAM", "FOOLPROOF" and "BONOBO" into their reverses. Show your work.
A palindrome is a sequence that is the same as its reverse. For example, the word "RACECAR" is a palindrome. For this problem we will define an almost palindrome to be a sequence that can be transformed into its reverse with 2 or fewer character additions, deletions, replacements, or swaps. A character swap swaps two adjacent characters. For instance "MARJORAM" can be reversed by swapping the 'J' and the 'O'. In addition, "FOOLPROOF" can be reversed by replacing the 'R' with and 'L' and vice versa. Finally, the word "BONOBO" can be reversed by deleting the final 'O' and reinserting it at the beginning. Devise a dynamic programming algorithm that will determine the number of edits necessary to to transform a sequence into its reverse. a) State this problem with formal input and output conditions. b) State a self-reduction for this problem. c) State a dynamic programming algorithm based off of your self-reduction that computes the minimum number of edits to transform a sequence into its reverse. d) Use your algorithm to determine the minimum number of edits to transform "MARJORAM", "FOOLPROOF" and "BONOBO" into their reverses. Show your work.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
![### Understanding Palindromes and Nearly-Palindromes
A **palindrome** is a sequence that reads the same forwards and backwards. For example, the word **"RACECAR"** is a palindrome.
For this problem, we introduce the concept of an **almost palindrome**. An almost palindrome is a sequence that can be transformed into its reverse with 2 or fewer character additions, deletions, replacements, or swaps. A character swap involves swapping two adjacent characters.
#### Examples:
- **"MARJORAM"** can be reversed by swapping the 'J' and the 'O'.
- **"FOOLPROOF"** can be reversed by replacing 'R' with 'L' and vice versa.
- The word **"BONOBO"** can be reversed by deleting the final 'O' and reinserting it at the beginning.
---
### Dynamic Programming Algorithm
The goal is to devise a dynamic programming algorithm to determine the number of edits necessary to transform a sequence into its reverse. Here are the steps:
1. **State the Problem with Formal Input and Output Conditions.**
2. **State a Self-Reduction for This Problem.**
3. **Develop a Dynamic Programming Algorithm Based on the Self-Reduction.**
4. **Determine the Minimum Number of Edits for Given Sequences Using the Algorithm.**
#### a) Problem Statement
**Input:** A string `S` of length `n`.
**Output:** The minimum number of edits (additions, deletions, replacements, swaps) required to transform `S` into its reverse, and whether it qualifies as an almost palindrome.
#### b) Self-Reduction
- **Base Case:** If the length of `S` is 0 or 1, `S` is already a palindrome, so the required edits are 0.
- **Recursive Case:**
- If the first and last characters of `S` are the same, the problem reduces to the same problem on the substring `S[1:-1]`.
- Otherwise, consider the following possible edits:
- Replace the first character with the last character, or vice versa.
- Delete the first character or the last character.
- Swap the first two characters, then solve the subproblem for `S[2:]`.
#### c) Dynamic Programming Algorithm
Let `dp[i][j]` represent the minimum number of edits required to transform the substring `S[i:j+1](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa0c2266a-9a17-4b5f-a3a4-de1bbbae4b7e%2F0b614819-f5d0-4524-b4cc-b41a12fba1fd%2Ffticw0l_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Understanding Palindromes and Nearly-Palindromes
A **palindrome** is a sequence that reads the same forwards and backwards. For example, the word **"RACECAR"** is a palindrome.
For this problem, we introduce the concept of an **almost palindrome**. An almost palindrome is a sequence that can be transformed into its reverse with 2 or fewer character additions, deletions, replacements, or swaps. A character swap involves swapping two adjacent characters.
#### Examples:
- **"MARJORAM"** can be reversed by swapping the 'J' and the 'O'.
- **"FOOLPROOF"** can be reversed by replacing 'R' with 'L' and vice versa.
- The word **"BONOBO"** can be reversed by deleting the final 'O' and reinserting it at the beginning.
---
### Dynamic Programming Algorithm
The goal is to devise a dynamic programming algorithm to determine the number of edits necessary to transform a sequence into its reverse. Here are the steps:
1. **State the Problem with Formal Input and Output Conditions.**
2. **State a Self-Reduction for This Problem.**
3. **Develop a Dynamic Programming Algorithm Based on the Self-Reduction.**
4. **Determine the Minimum Number of Edits for Given Sequences Using the Algorithm.**
#### a) Problem Statement
**Input:** A string `S` of length `n`.
**Output:** The minimum number of edits (additions, deletions, replacements, swaps) required to transform `S` into its reverse, and whether it qualifies as an almost palindrome.
#### b) Self-Reduction
- **Base Case:** If the length of `S` is 0 or 1, `S` is already a palindrome, so the required edits are 0.
- **Recursive Case:**
- If the first and last characters of `S` are the same, the problem reduces to the same problem on the substring `S[1:-1]`.
- Otherwise, consider the following possible edits:
- Replace the first character with the last character, or vice versa.
- Delete the first character or the last character.
- Swap the first two characters, then solve the subproblem for `S[2:]`.
#### c) Dynamic Programming Algorithm
Let `dp[i][j]` represent the minimum number of edits required to transform the substring `S[i:j+1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
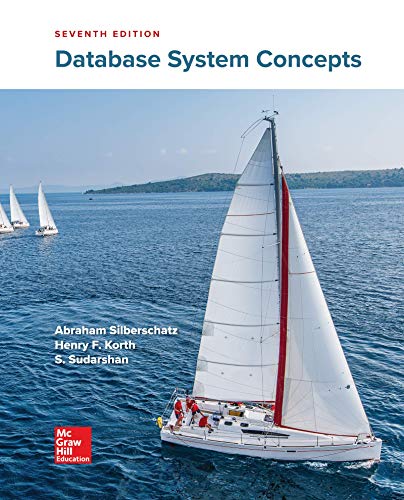
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
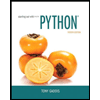
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
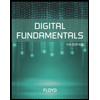
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
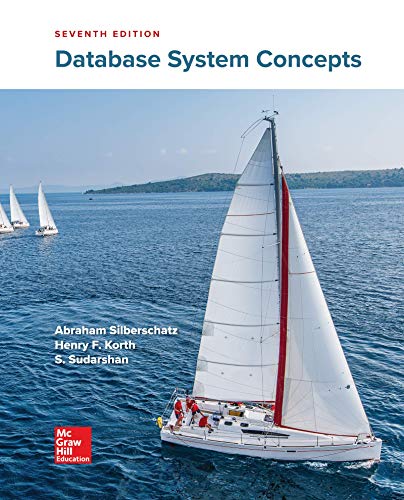
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
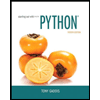
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
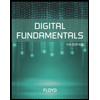
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
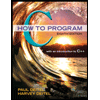
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
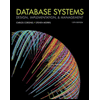
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
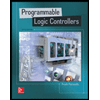
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education