a method called printPowersOfN that accepts a base and a maximum exponent as arguments. The method prints each power of the base from 0 up to that maximum exponent, inclusive. For example: printPowersOfN(4, 3); // should output 1 4 16 64 This calculates 40, 41, 42, and 43. printPowersOfN(5, 6); // should output 1 5 25 125 625 3125 15625 This calculates 50, 51, 52, 53, 54, 55, and 56. printPowersOfN(–2, 8); // should output 1 –2 4 –8 16 -32 64 –128 256 Both arguments are integers. You may assume that the maximum exponent passed to printPowersOfN has a value of 0 or greater.
Write a method called printPowersOfN that accepts a base and a maximum exponent as arguments. The method prints each power of the base from 0 up to that maximum exponent, inclusive.
For example:
printPowersOfN(4, 3); // should output 1 4 16 64
This calculates 40, 41, 42, and 43.
printPowersOfN(5, 6); // should output 1 5 25 125 625 3125 15625
This calculates 50, 51, 52, 53, 54, 55, and 56.
printPowersOfN(–2, 8); // should output 1 –2 4 –8 16 -32 64 –128 256
Both arguments are integers. You may assume that the maximum exponent passed to printPowersOfN has a value of 0 or greater.
The Math class may help you with this problem! If you use it, you may need to cast its results from double to int so that you don’t see a .0 after each number in your output. You may also write this program without using the Math class. Either solution is acceptable so long as it displays the correct output.
Please use the three sample calls in your submitted program run. You are welcome to include additional calls, with other values, after these three. Your program must work for other valid integers besides those listed in the sample calls.
Rubric:
- Student name and date is in a comment on the first line of the program: -5 points if fails
- The three sample calls are included in program output: -3 points if fails
- printPowersOfN defined with appropriate argument data types: 3 points
- printPowersOfN loop: 2 points
- printPowersOfN calculations: 2 points
- Program structure, program works correctly: 3 point
*please follow rubric


Trending now
This is a popular solution!
Step by step
Solved in 3 steps

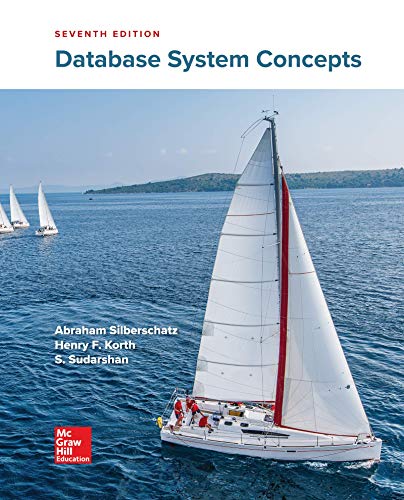
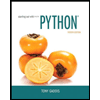
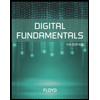
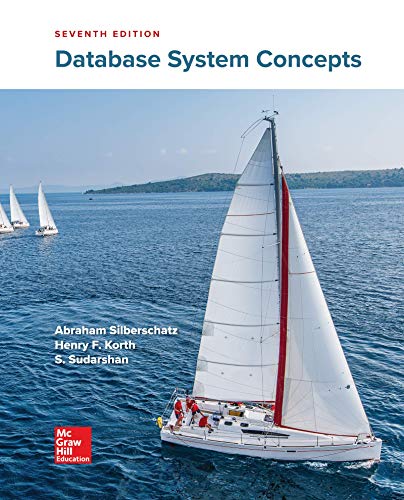
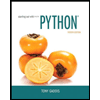
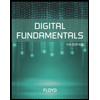
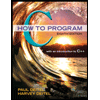
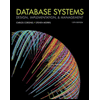
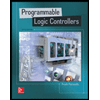