A-G, determine the output displayed by the lines of code. Save your code as PE4 1.pyv. str - "Python 123" print (str) print (str[0]) print (str[-1]) print (str[:]) print("Python") print ("Python" [0]) print ("Python"[-1]) print ("Python"[:1) в |Output Output strNum = "0, 1, 2, 3, 4, 5, 6, 7, 8, 9" print (strNum [1], strNum[-1], len(strNum)) print (strNum [:len (strNum) ]) print (strNum [1]+strNum[-3])
A-G, determine the output displayed by the lines of code. Save your code as PE4 1.pyv. str - "Python 123" print (str) print (str[0]) print (str[-1]) print (str[:]) print("Python") print ("Python" [0]) print ("Python"[-1]) print ("Python"[:1) в |Output Output strNum = "0, 1, 2, 3, 4, 5, 6, 7, 8, 9" print (strNum [1], strNum[-1], len(strNum)) print (strNum [:len (strNum) ]) print (strNum [1]+strNum[-3])
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Need help with Python. Answer all parts
![Certainly! Below is the transcription of the image, formatted as it would appear on an educational website.
---
**Exercise 1: Determine the Output**
For each block A–G, determine the output displayed by the lines of code. Save your code as `PE4_1.py`.
### A
```python
print("Python")
print("Python"[0])
print("Python"[-1])
print("Python"[:])
```
**Output:**
```
Python
P
n
Python
```
### B
```python
str = "Python 123"
print(str)
print(str[0])
print(str[-1])
print(str[:])
```
**Output:**
```
Python 123
P
3
Python 123
```
### C
```python
strNum = "0, 1, 2, 3, 4, 5, 6, 7, 8, 9"
print(strNum[1], strNum[-1], len(strNum))
print(strNum[:len(strNum)])
print(strNum[1]+strNum[-3])
```
**Output:**
```
, 9 29
0, 1, 2, 3, 4, 5, 6, 7, 8, 9
,7
```
### D
```python
n = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(n)
print(n[0], n[1], n[9])
print(n[3:])
print(n[:5])
print(n[-5:-1])
print(n[-1] + n[-2])
print(len(n), n[:len(n)], sep = '\n')
print(min(n), max(n), type(n))
```
**Output:**
```
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
1 2 10
[4, 5, 6, 7, 8, 9, 10]
[1, 2, 3, 4, 5]
[6, 7, 8, 9]
19
10
10
[1, 2, 3, 4, 5, 6,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F31e55ccf-8f70-4a32-a28b-4816d60dca82%2F74b62afc-d846-46ef-80b3-f4944a23e39c%2Fw0rmmr_processed.jpeg&w=3840&q=75)
Transcribed Image Text:Certainly! Below is the transcription of the image, formatted as it would appear on an educational website.
---
**Exercise 1: Determine the Output**
For each block A–G, determine the output displayed by the lines of code. Save your code as `PE4_1.py`.
### A
```python
print("Python")
print("Python"[0])
print("Python"[-1])
print("Python"[:])
```
**Output:**
```
Python
P
n
Python
```
### B
```python
str = "Python 123"
print(str)
print(str[0])
print(str[-1])
print(str[:])
```
**Output:**
```
Python 123
P
3
Python 123
```
### C
```python
strNum = "0, 1, 2, 3, 4, 5, 6, 7, 8, 9"
print(strNum[1], strNum[-1], len(strNum))
print(strNum[:len(strNum)])
print(strNum[1]+strNum[-3])
```
**Output:**
```
, 9 29
0, 1, 2, 3, 4, 5, 6, 7, 8, 9
,7
```
### D
```python
n = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
print(n)
print(n[0], n[1], n[9])
print(n[3:])
print(n[:5])
print(n[-5:-1])
print(n[-1] + n[-2])
print(len(n), n[:len(n)], sep = '\n')
print(min(n), max(n), type(n))
```
**Output:**
```
[1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
1 2 10
[4, 5, 6, 7, 8, 9, 10]
[1, 2, 3, 4, 5]
[6, 7, 8, 9]
19
10
10
[1, 2, 3, 4, 5, 6,
![The image provides two code examples that demonstrate sorting a list of fruits using Python. Let's explore each example:
### Example H
```python
fruits = ['kiwi', 'pear', 'orange', 'apple', 'cherry']
fruits.sort()
print(fruits)
fruits.sort(reverse=True)
print(fruits[0])
```
**Explanation:**
- A list of fruits is initialized.
- The `sort()` method is called on the list, which sorts the list in ascending order.
- The sorted list is then printed.
- The `sort(reverse=True)` method is called, which sorts the list in descending order.
- The first element of the descending list is printed.
### Output for Example H
There is a labeled section for output, but the actual results are not shown in the image.
---
### Example I
```python
fruits = ['kiwi', 'pear', 'orange', 'apple', 'cherry']
print(sorted(fruits))
print(fruits[0])
print(sorted(fruits, reverse=True))
print(fruits[0])
```
**Explanation:**
- A list of fruits is initialized.
- The `sorted()` function is used to return a new list that is sorted in ascending order. This does not modify the original list.
- The sorted list is printed.
- The first element of the original list is printed.
- The `sorted()` function is used again, this time with the `reverse=True` parameter to return a new list sorted in descending order.
- The descending sorted list is printed.
- Finally, the first element of the original list is printed again.
### Output for Example I
As with Example H, the output is labeled but not displayed in the image.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F31e55ccf-8f70-4a32-a28b-4816d60dca82%2F74b62afc-d846-46ef-80b3-f4944a23e39c%2Ftez4ydt_processed.jpeg&w=3840&q=75)
Transcribed Image Text:The image provides two code examples that demonstrate sorting a list of fruits using Python. Let's explore each example:
### Example H
```python
fruits = ['kiwi', 'pear', 'orange', 'apple', 'cherry']
fruits.sort()
print(fruits)
fruits.sort(reverse=True)
print(fruits[0])
```
**Explanation:**
- A list of fruits is initialized.
- The `sort()` method is called on the list, which sorts the list in ascending order.
- The sorted list is then printed.
- The `sort(reverse=True)` method is called, which sorts the list in descending order.
- The first element of the descending list is printed.
### Output for Example H
There is a labeled section for output, but the actual results are not shown in the image.
---
### Example I
```python
fruits = ['kiwi', 'pear', 'orange', 'apple', 'cherry']
print(sorted(fruits))
print(fruits[0])
print(sorted(fruits, reverse=True))
print(fruits[0])
```
**Explanation:**
- A list of fruits is initialized.
- The `sorted()` function is used to return a new list that is sorted in ascending order. This does not modify the original list.
- The sorted list is printed.
- The first element of the original list is printed.
- The `sorted()` function is used again, this time with the `reverse=True` parameter to return a new list sorted in descending order.
- The descending sorted list is printed.
- Finally, the first element of the original list is printed again.
### Output for Example I
As with Example H, the output is labeled but not displayed in the image.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Recommended textbooks for you
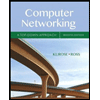
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
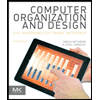
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
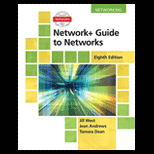
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
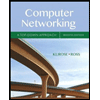
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
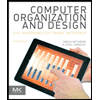
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
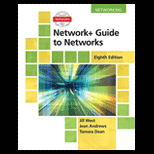
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
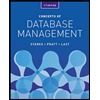
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
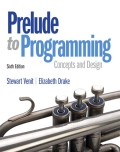
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
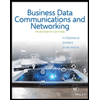
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY