A county collects property taxes on the assessment value of property, which is 60 percent of the property’s actual value. For example, if an acre of land is valued at $10,000, its assessment value is $6,000. The property tax is then 72¢ for each $100 of the assessment value. The tax for the acre assessed at $6,000 will be $43.20. Write a Python program using functions that asks for the actual value of a piece of property and displays the assessment value and property tax This is Python Programming. I don't know where to go from here.
A county collects property taxes on the assessment value of property, which is 60 percent of the property’s actual value. For example, if an acre of land is valued at $10,000, its assessment value is $6,000. The property tax is then 72¢ for each $100 of the assessment value. The tax for the acre assessed at $6,000 will be $43.20. Write a Python program using functions that asks for the actual value of a piece of property and displays the assessment value and property tax This is Python Programming. I don't know where to go from here.
Programming with Microsoft Visual Basic 2017
8th Edition
ISBN:9781337102124
Author:Diane Zak
Publisher:Diane Zak
Chapter1: An Introduction To Visual Studio 2017 And Visual Basic
Section: Chapter Questions
Problem 4MQ7
Related questions
Question
100%
A county collects property taxes on the assessment value of property, which is 60 percent of the property’s actual
value. For example, if an acre of land is valued at $10,000, its assessment value is $6,000. The property tax is then
72¢ for each $100 of the assessment value. The tax for the acre assessed at $6,000 will be $43.20. Write a Python
program using functions that asks for the actual value of a piece of property and displays the assessment value and
property tax
This is Python

Transcribed Image Text:LabExercise7B – LabExercise7B.py
LabExercise7B LabExercise7B.py
LabExercise7B
Project
LabExercise7B.py
LabExercise7B ~/PycharmProjects/LabExercise7E 1
def main():
91 A 2 A 3
venv
2
# Ask for the property's assessment value.
bin
propertyvalue = input("Enter the property's actual value: ")
>
lib
4
prop(propertyvalue)
fo -gitignore
E pyvenv.cfg
LabExercise7B.py
Edef prop():
> ulı External Libraries
7
# Calculate the property's assessment value using the actual value.
assessmentvalue = propertyvalue * 0.6
print("The assessment value is $%.2f" % assessmentvalue)
8.
>
Scratches and Consoles
10
assessment(assessmentvalue)
11
12
Edef assessment(assessmentvalue):
propertytax = assessmentvalue * 0.0064
print("The property tax is $%.2f" % propertytax)
13
14
15
main()
16
asessment()
|
17
Run:
LabExercise7B x
/Users/gracemcdonald/PycharmProjects/LabExercise7B/venv/bin/python /Users/gracemcdonald/PycharmProjects/LabExercise7B/LabExercise7B.py
Enter the property's actual value: 10000
Traceback (most recent call last):
File "/Users/gracemcdonald/PycharmProjects/LabExercise7B/LabExercise7B.py", line 15, in <module>
%3D
main()
File "/Users/gracemcdonald/PycharmProjects/LabExercise7B/LabExercise7B.py", line 4, in main
prop(propertyvalue)
TypeError: prop() takes 0 positional arguments but 1 was given
Process finished with exit code 1
Run
E TODO
O Problems
- Terminal
Python Packages
Python Console
Event Log
17:1
LF UTF-8
4 spaces Python 3.9 (LabExercise7B)
I Project
* Favorites
H. Structure
LO
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
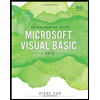
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
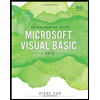
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning