777 Simulating the Election Your program will run a series of simulations of the same election. Each simulation will be run as follows: 1. For each district: 1. Randomly determine how many people voted in this district. 2. Randomly determine what percentage of the vote our candidate received in this district. 3. Multiply these two values together to compute how many votes our candidate received in this district. 4. Add the number of votes our candidate received and the total number of votes cast in this district to the overall totals. 2. Compute the overall percentage of the vote our candidate received across all districts. 3. Print out the results (see below) 4. Add the turnout and votes earned in this simulation to the overall totals (see below) Your program should execute this entire procedure (including the output) for each simulation. After all simulations have been run, your program should all print out the average vote percentage our candidate received across all simulations. (If we've done things right, that should be pretty close to the polling average, though it won't exactly match.)
Running 5 simulations of 10 districts
see the requirements on the image


Python programming language:
The initial version of Python was launched in 1990 and was developed by Dutch programmer Guido van Rossum. Because they are frequently created to complete a single objective, programming languages have a limited range of applications. But the multi-paradigm language is Python. Although it excels as an object-oriented programming language, functional programming is also an option if that's what you choose to use when creating applications. Python has established itself as the standard language for numerous disciplines and sectors as a result of its flexibility.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Thank you. With these two more requirements what should the codes be
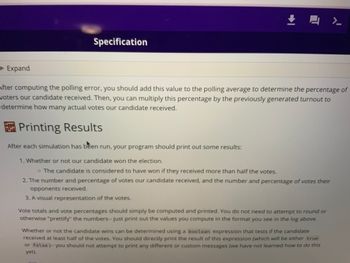
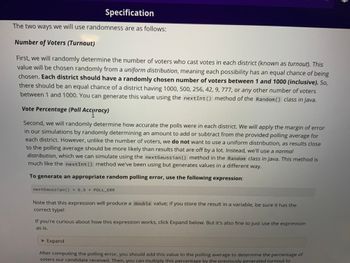
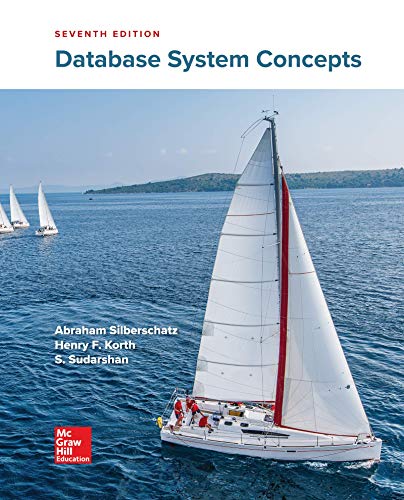
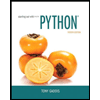
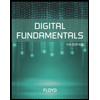
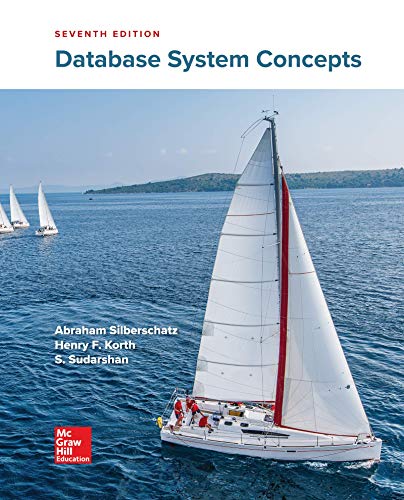
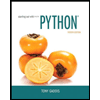
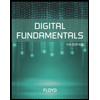
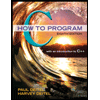
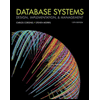
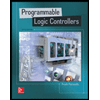