6.23 LAB: Winning team (classes) Given main(), define the Team class (in files Team.h and Team.cpp). For class member function GetWinPercentage(), the formula is: wins (wins + losses). Note: Use casting to prevent integer division. For class member function PrintStanding(), output the win percentage of the team with two digits after the decimal point and whether the team has a winning or losing average. A team has a winning average if the win percentage is 0.5 or greater. Ex: If the input is Ravens 13 3, where Ravens is the team's name, 13 is the number of team wins, and 3 is the number of team losses, the output is: Win percentage: 0.81 Congratulations, Team Ravens has a winning average! Ex: If the input is Angels 80 82, the output is: Win percentage: 0.49 Team Angels has a losing average.
6.23 LAB: Winning team (classes) Given main(), define the Team class (in files Team.h and Team.cpp). For class member function GetWinPercentage(), the formula is: wins (wins + losses). Note: Use casting to prevent integer division. For class member function PrintStanding(), output the win percentage of the team with two digits after the decimal point and whether the team has a winning or losing average. A team has a winning average if the win percentage is 0.5 or greater. Ex: If the input is Ravens 13 3, where Ravens is the team's name, 13 is the number of team wins, and 3 is the number of team losses, the output is: Win percentage: 0.81 Congratulations, Team Ravens has a winning average! Ex: If the input is Angels 80 82, the output is: Win percentage: 0.49 Team Angels has a losing average.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Please use C++. I need to fill out "Team.H" and "Team.cpp." Main.cpp is given and you cannot edit it.

Transcribed Image Text:### 6.23 LAB: Winning team (classes)
Given `main()`, define the `Team` class (in files `Team.h` and `Team.cpp`). For class member function `GetWinPercentage()`, the formula is:
```
wins / (wins + losses). Note: Use casting to prevent integer division.
```
For class member function `PrintStanding()`, output the win percentage of the team with two digits after the decimal point and whether the team has a winning or losing average. A team has a winning average if the win percentage is 0.5 or greater.
**Example 1:**
If the input is `Ravens 13 3`, where Ravens is the team's name, 13 is the number of team wins, and 3 is the number of team losses, the output is:
```
Win percentage: 0.81
Congratulations, Team Ravens has a winning average!
```
**Example 2:**
If the input is `Angels 80 82`, the output is:
```
Win percentage: 0.49
Team Angels has a losing average.
```

Transcribed Image Text:### Basic C++ Program for Managing Team Information
This C++ code demonstrates how to manage basic information about a team, such as the team’s name, wins, and losses, using a simple class and standard input/output operations.
#### Code Explanation:
```cpp
#include <iostream>
#include <string>
#include "Team.h"
using namespace std;
int main() {
string name;
int wins;
int losses;
Team team;
cin >> name;
cin >> wins;
cin >> losses;
team.SetName(name);
team.SetWins(wins);
team.SetLosses(losses);
team.PrintStanding();
return 0;
}
```
### Detailed Breakdown:
1. **Header Inclusions and Namespace Declaration:**
```cpp
#include <iostream>
#include <string>
#include "Team.h"
using namespace std;
```
- The code starts by including the necessary headers: `<iostream>` and `<string>`. These headers allow the program to perform input/output operations and utilize the string class, respectively.
- `#include "Team.h"` suggests that the `Team` class is defined elsewhere, specifically in a header file named `Team.h`.
- `using namespace std;` allows the program to use standard library features without the `std::` prefix.
2. **Main Function and Variable Declaration:**
```cpp
int main() {
string name;
int wins;
int losses;
Team team;
```
- The `main()` function is the entry point of the program.
- Variables `name`, `wins`, and `losses` are declared to store the respective information.
- An object `team` of the `Team` class is created.
3. **Input Operations:**
```cpp
cin >> name;
cin >> wins;
cin >> losses;
```
- The program reads input values for the team name, number of wins, and number of losses from the user.
4. **Setting Attributes and Printing Standing:**
```cpp
team.SetName(name);
team.SetWins(wins);
team.SetLosses(losses);
team.PrintStanding();
```
- The `SetName`, `SetWins`, and `SetLosses` methods of the `Team` class are called to set the respective attributes of the team.
- Finally, the
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
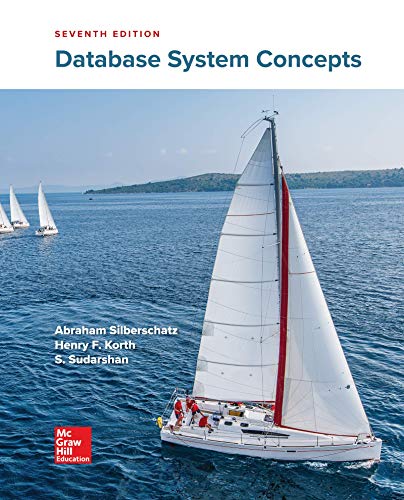
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
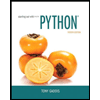
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
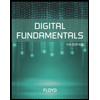
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
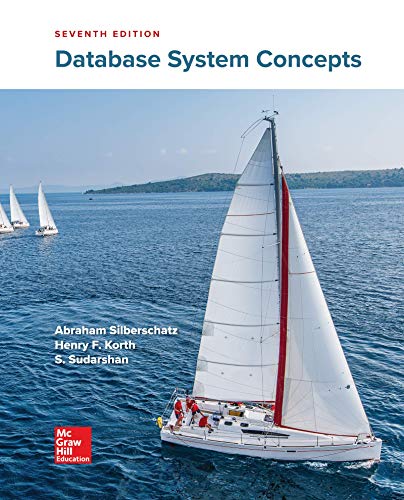
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
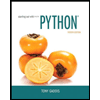
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
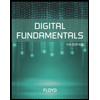
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
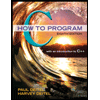
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
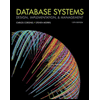
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
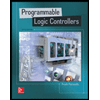
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education