6.17 LAB: Max magnitude Write a method maxMagnitude() with two integer input parameters that returns the largest magnitude value. Use the method in a program that takes two integer inputs, and outputs the largest magnitude value. Ex: If the inputs are: 5 7 the method returns: 7 Ex: If the inputs are: -8 -2 the method returns: -8 Note: The method does not just return the largest value, which for -8 -2 would be -2. Though not necessary, you may use the absolute-value built-in math method. Your program must define and call a method: public static int maxMagnitude(int userVal1, int userVal2) tested with 5,7 -8,-2, 25,-50 import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner input = new Scanner(System.in); int number1 = input.nextInt(); int number2 = input.nextInt(); System.out.println(maxMagnitude(number1, number2)); } public static int maxMagnitude(int number1, int number2){ number1 = Math.abs(number1); number2 = Math.abs(number2); if(number1 >= number2) return number1; else return number2; } } Enter program input (optional) Run program Input (from above)trending_flat LabProgram.java (Your program) trending_flatOutput (shown below) Program errors displayed here Exception in thread "main" java.util.InputMismatchException at java.base/java.util.Scanner.throwFor(Scanner.java:939) at java.base/java.util.Scanner.next(Scanner.java:1594) at java.base/java.util.Scanner.nextInt(Scanner.java:2258) at java.base/java.util.Scanner.nextInt(Scanner.java:2212) at LabProgram.main(LabProgram.java:9)
6.17 LAB: Max magnitude
Write a method maxMagnitude() with two integer input parameters that returns the largest magnitude value. Use the method in a
Ex: If the inputs are:
5 7the method returns:
7Ex: If the inputs are:
-8 -2the method returns:
-8Note: The method does not just return the largest value, which for -8 -2 would be -2. Though not necessary, you may use the absolute-value built-in math method.
Your program must define and call a method:
public static int maxMagnitude(int userVal1, int userVal2) tested with 5,7 -8,-2, 25,-50
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int number1 = input.nextInt();
int number2 = input.nextInt();
System.out.println(maxMagnitude(number1, number2));
}
public static int maxMagnitude(int number1, int number2){
number1 = Math.abs(number1);
number2 = Math.abs(number2);
if(number1 >= number2)
return number1;
else
return number2;
}
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

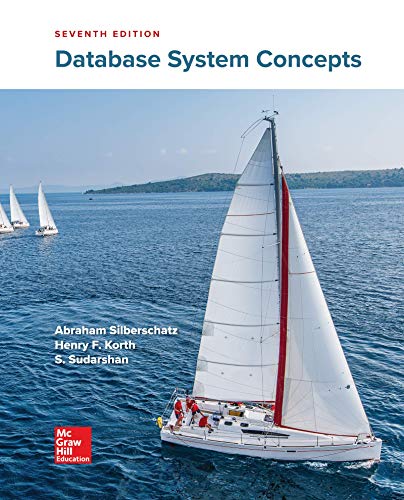
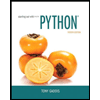
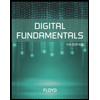
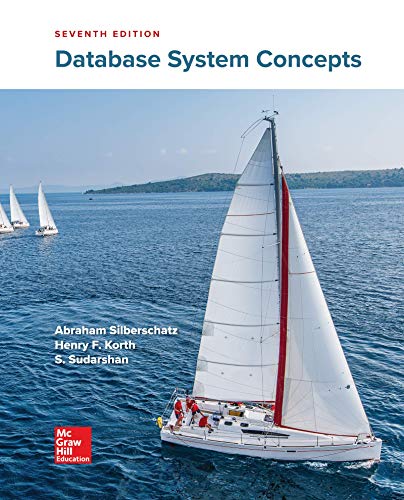
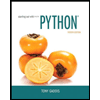
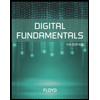
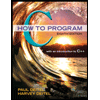
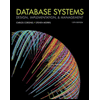
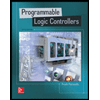