Part 1 Given 4 integers, output their product and their average, using integer arithmetic. Ex: If the input is: 8 10 5 4 the output is: 1600 6 Note: Integer division discards the fraction. Hence the average of 8 10 5 4 is output as 6, not 6.75. Note: The test cases include four very large input values whose product results in overflow. You do not need to do anything special, but just observe that the output does not represent the correct product (in fact, four positive numbers yield a negative output; wow). Submit the above for grading. Your program will fail the last test cases (which is expected), until you complete part 2 below. Part 2 Also output the product and average, using floating-point arithmetic. Output each floating-point value with three digits after the decimal point, which can be achieved by executing cout << fixed << setprecision(3); once before all other cout statements. Ex: If the input is: 8 10 5 4 the output is: 1600 6 1600.000 6.750 Note that fractions aren't discarded, and that overflow does not occur for the test case with large values.
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
Part 1
Given 4 integers, output their product and their average, using integer arithmetic.
Ex: If the input is:
8 10 5 4
the output is:
1600 6
Note: Integer division discards the fraction. Hence the average of 8 10 5 4 is output as 6, not 6.75.
Note: The test cases include four very large input values whose product results in overflow. You do not need to do anything special, but just observe that the output does not represent the correct product (in fact, four positive numbers yield a negative output; wow).
Submit the above for grading. Your
Part 2
Also output the product and average, using floating-point arithmetic.
Output each floating-point value with three digits after the decimal point, which can be achieved by executing
cout << fixed << setprecision(3); once before all other cout statements.
Ex: If the input is:
8 10 5 4
the output is:
1600 6 1600.000 6.750
Note that fractions aren't discarded, and that overflow does not occur for the test case with large values.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Where did I go wrong?
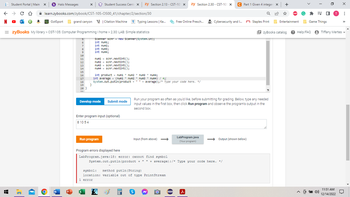
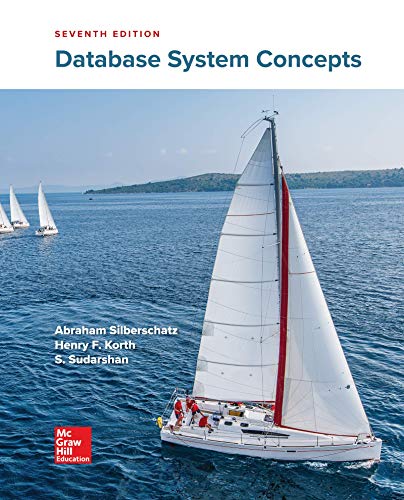
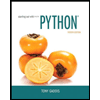
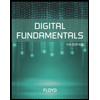
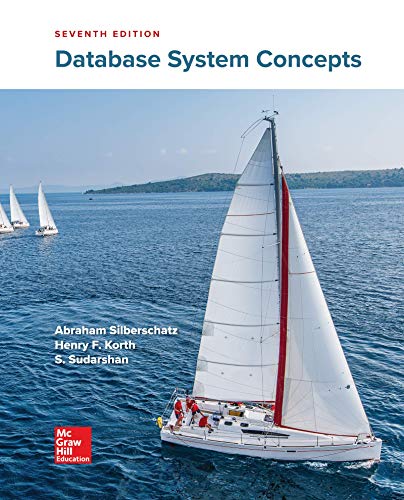
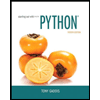
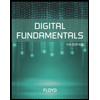
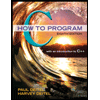
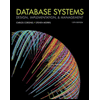
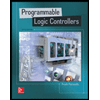