The problem is that the data file is corrupted. You do not know where the file is corrupted, but you know that if you reach a data column that is not an integer, that you should stop processing all years after that data column and move to the next row, because all the data after any corrupted data is untrustworthy or also corrupted. Your task is to write a function named calculate_total_production. Your function must take one parameter, a dictionary containing coal production of the same format returned by read_data_file. Your function must use a while loop to generate a dictionary where the key is the county name and the value is the total number of tons of coal produced in that county. Your function should return the generated dictionary. Verify that the function works as intended by adding statements that include a call to this function. D Run lab12_p1.py x Online (1) zbessant Bessant lab12_p5.py x lab12 p3.py x lab12 p2.pyx coal_production. 1 # Problem #5 2 3 # Read the contents of a coal data file and return the data in a dictionary format. 4 def read_data_file(filename): 5 6 7 8 data_file = open(filename, "r") header_line = data_file.readline() # Read the header line of the file to extract years years header_line.strip().split(",")[1:] # Extract the years from the header line 9 10 11 # Initialize an empty dictionary to store the data data_dict = {} 12 13 # Iterate over each line in the data file CONSOLE SHELL Hint: Using isinstance(x, int) will return True when x is and integer and False otherwise. 5. In lab12_p5.py a function read_data_file has been created. This function takes in one string parameter that represents the name of the file to be processed. This function reads data from the provided file and returns a dictionary representation of the data stored in this file. The data file is a comma-separated file with the following columns: • County name • Tons of coal produced in 1900 • Tons of coal produced in 1910 © Run Online (1) zbessant Bessant lab12_p1.py x lab12_p5.py x lab12 p3.pyx lab12_p2.py x coal production. 1 # Problem #5 2 3 4 5 6 7 8 # Read the contents of a coal data file and return the data in a dictionary format. def read_data_file(filename): data file open(filename, "r") header_line = data_file.readline () # Read the header line of the file to extract years years = header_line.strip().split(",")[1:] # Extract the years from the header line Tons of coal produced in 1920 9 10 Tons of coal produced in 1930 11 # Initialize an empty dictionary to store the data data_dict = {} Tons of coal produced in 1940 12 13 # Iterate over each line in the data file Tons of coal produced in 1950 • Tons of coal produced in 1960 • Tons of coal produced in 1970 • Tons of coal produced in 1980 • Tons of coal produced in 1990 Tons of coal produced in 2000 Tons of coal produced in 2010 The returned data structure is a nested dictionary. The key of the top-level (outer) dictionary is the name of the county, and its value is another dictionary. In the second- CONSOLE SHELL Check Answer Reset to Template Recent Submissions
The problem is that the data file is corrupted. You do not know where the file is corrupted, but you know that if you reach a data column that is not an integer, that you should stop processing all years after that data column and move to the next row, because all the data after any corrupted data is untrustworthy or also corrupted. Your task is to write a function named calculate_total_production. Your function must take one parameter, a dictionary containing coal production of the same format returned by read_data_file. Your function must use a while loop to generate a dictionary where the key is the county name and the value is the total number of tons of coal produced in that county. Your function should return the generated dictionary. Verify that the function works as intended by adding statements that include a call to this function. D Run lab12_p1.py x Online (1) zbessant Bessant lab12_p5.py x lab12 p3.py x lab12 p2.pyx coal_production. 1 # Problem #5 2 3 # Read the contents of a coal data file and return the data in a dictionary format. 4 def read_data_file(filename): 5 6 7 8 data_file = open(filename, "r") header_line = data_file.readline() # Read the header line of the file to extract years years header_line.strip().split(",")[1:] # Extract the years from the header line 9 10 11 # Initialize an empty dictionary to store the data data_dict = {} 12 13 # Iterate over each line in the data file CONSOLE SHELL Hint: Using isinstance(x, int) will return True when x is and integer and False otherwise. 5. In lab12_p5.py a function read_data_file has been created. This function takes in one string parameter that represents the name of the file to be processed. This function reads data from the provided file and returns a dictionary representation of the data stored in this file. The data file is a comma-separated file with the following columns: • County name • Tons of coal produced in 1900 • Tons of coal produced in 1910 © Run Online (1) zbessant Bessant lab12_p1.py x lab12_p5.py x lab12 p3.pyx lab12_p2.py x coal production. 1 # Problem #5 2 3 4 5 6 7 8 # Read the contents of a coal data file and return the data in a dictionary format. def read_data_file(filename): data file open(filename, "r") header_line = data_file.readline () # Read the header line of the file to extract years years = header_line.strip().split(",")[1:] # Extract the years from the header line Tons of coal produced in 1920 9 10 Tons of coal produced in 1930 11 # Initialize an empty dictionary to store the data data_dict = {} Tons of coal produced in 1940 12 13 # Iterate over each line in the data file Tons of coal produced in 1950 • Tons of coal produced in 1960 • Tons of coal produced in 1970 • Tons of coal produced in 1980 • Tons of coal produced in 1990 Tons of coal produced in 2000 Tons of coal produced in 2010 The returned data structure is a nested dictionary. The key of the top-level (outer) dictionary is the name of the county, and its value is another dictionary. In the second- CONSOLE SHELL Check Answer Reset to Template Recent Submissions
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![The problem is that the data file is corrupted. You do not
know where the file is corrupted, but you know that if you
reach a data column that is not an integer, that you should
stop processing all years after that data column and move to
the next row, because all the data after any corrupted data is
untrustworthy or also corrupted.
Your task is to write a function named
calculate_total_production.
Your function must take one parameter, a dictionary
containing coal production of the same format returned by
read_data_file.
Your function must use a while loop to generate a dictionary
where the key is the county name and the value is the total
number of tons of coal produced in that county. Your
function should return the generated dictionary. Verify that
the function works as intended by adding statements that
include a call to this function.
D Run
lab12_p1.py x
Online (1) zbessant Bessant
lab12_p5.py x lab12 p3.py x
lab12 p2.pyx
coal_production.
1 # Problem #5
2
3
# Read the contents of a coal data file and return the data in a dictionary
format.
4
def read_data_file(filename):
5
6
7
8
data_file = open(filename, "r")
header_line = data_file.readline() # Read the header line of the file to
extract years
years header_line.strip().split(",")[1:] # Extract the years from the
header line
9
10
11
# Initialize an empty dictionary to store the data
data_dict = {}
12
13
# Iterate over each line in the data file
CONSOLE
SHELL
Hint: Using isinstance(x, int) will return True when x is
and integer and False otherwise.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc158a850-76a9-4504-97b9-8593e0926539%2Fc765f232-0cf9-4a0f-963b-bd447727e6a9%2Fy35vx2n_processed.png&w=3840&q=75)
Transcribed Image Text:The problem is that the data file is corrupted. You do not
know where the file is corrupted, but you know that if you
reach a data column that is not an integer, that you should
stop processing all years after that data column and move to
the next row, because all the data after any corrupted data is
untrustworthy or also corrupted.
Your task is to write a function named
calculate_total_production.
Your function must take one parameter, a dictionary
containing coal production of the same format returned by
read_data_file.
Your function must use a while loop to generate a dictionary
where the key is the county name and the value is the total
number of tons of coal produced in that county. Your
function should return the generated dictionary. Verify that
the function works as intended by adding statements that
include a call to this function.
D Run
lab12_p1.py x
Online (1) zbessant Bessant
lab12_p5.py x lab12 p3.py x
lab12 p2.pyx
coal_production.
1 # Problem #5
2
3
# Read the contents of a coal data file and return the data in a dictionary
format.
4
def read_data_file(filename):
5
6
7
8
data_file = open(filename, "r")
header_line = data_file.readline() # Read the header line of the file to
extract years
years header_line.strip().split(",")[1:] # Extract the years from the
header line
9
10
11
# Initialize an empty dictionary to store the data
data_dict = {}
12
13
# Iterate over each line in the data file
CONSOLE
SHELL
Hint: Using isinstance(x, int) will return True when x is
and integer and False otherwise.
![5. In lab12_p5.py a function read_data_file has been
created. This function takes in one string parameter that
represents the name of the file to be processed. This
function reads data from the provided file and returns a
dictionary representation of the data stored in this file.
The data file is a comma-separated file with the following
columns:
• County name
• Tons of coal produced in 1900
• Tons of coal produced in 1910
©
Run
Online (1) zbessant Bessant
lab12_p1.py x lab12_p5.py x
lab12 p3.pyx
lab12_p2.py x
coal production.
1 # Problem #5
2
3
4
5
6
7
8
# Read the contents of a coal data file and return the data in a dictionary
format.
def read_data_file(filename):
data file open(filename, "r")
header_line = data_file.readline () # Read the header line of the file to
extract years
years = header_line.strip().split(",")[1:] # Extract the years from the
header line
Tons of coal produced in 1920
9
10
Tons of coal produced in 1930
11
# Initialize an empty dictionary to store the data
data_dict = {}
Tons of coal produced in 1940
12
13
# Iterate over each line in the data file
Tons of coal produced in 1950
• Tons of coal produced in 1960
• Tons of coal produced in 1970
• Tons of coal produced in 1980
• Tons of coal produced in 1990
Tons of coal produced in 2000
Tons of coal produced in 2010
The returned data structure is a nested dictionary. The key
of the top-level (outer) dictionary is the name of the
county, and its value is another dictionary. In the second-
CONSOLE
SHELL
Check Answer
Reset to Template Recent Submissions](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fc158a850-76a9-4504-97b9-8593e0926539%2Fc765f232-0cf9-4a0f-963b-bd447727e6a9%2Fxqyei9m_processed.png&w=3840&q=75)
Transcribed Image Text:5. In lab12_p5.py a function read_data_file has been
created. This function takes in one string parameter that
represents the name of the file to be processed. This
function reads data from the provided file and returns a
dictionary representation of the data stored in this file.
The data file is a comma-separated file with the following
columns:
• County name
• Tons of coal produced in 1900
• Tons of coal produced in 1910
©
Run
Online (1) zbessant Bessant
lab12_p1.py x lab12_p5.py x
lab12 p3.pyx
lab12_p2.py x
coal production.
1 # Problem #5
2
3
4
5
6
7
8
# Read the contents of a coal data file and return the data in a dictionary
format.
def read_data_file(filename):
data file open(filename, "r")
header_line = data_file.readline () # Read the header line of the file to
extract years
years = header_line.strip().split(",")[1:] # Extract the years from the
header line
Tons of coal produced in 1920
9
10
Tons of coal produced in 1930
11
# Initialize an empty dictionary to store the data
data_dict = {}
Tons of coal produced in 1940
12
13
# Iterate over each line in the data file
Tons of coal produced in 1950
• Tons of coal produced in 1960
• Tons of coal produced in 1970
• Tons of coal produced in 1980
• Tons of coal produced in 1990
Tons of coal produced in 2000
Tons of coal produced in 2010
The returned data structure is a nested dictionary. The key
of the top-level (outer) dictionary is the name of the
county, and its value is another dictionary. In the second-
CONSOLE
SHELL
Check Answer
Reset to Template Recent Submissions
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 4 images

Recommended textbooks for you
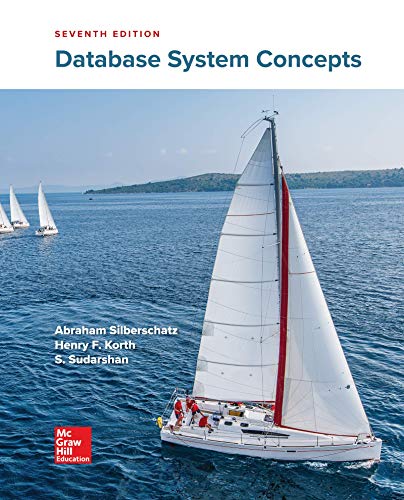
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
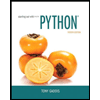
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
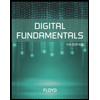
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
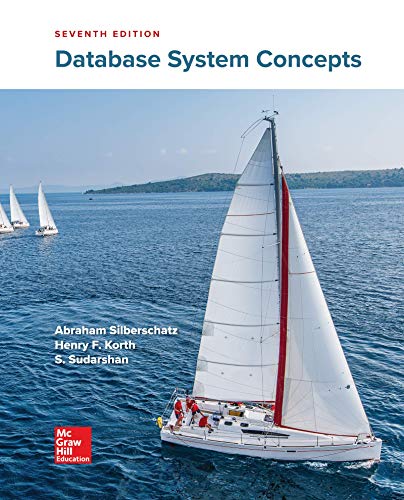
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
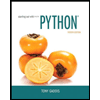
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
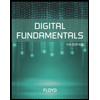
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
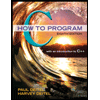
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
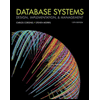
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
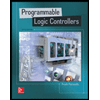
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education