4.17 LAB: Brute force equation solver Numerous engineering and scientific applications require finding solutions to a set of equations. Ex: 8x + 7y=38 and 3x - 5y = -1 have a solution x = 3, y=2. Given integer coefficients of two linear equations with variables x and y, use brute force to find an integer solution for x and y in the range-10 to 10. Ex: If the input is: 87 38 3 -5 -1 the output is: 32 because 8x3 +7x2-38 and 3x3 +-5x2=-1. Use this brute force approach: For every value of x from -10 to 10 For every value of y from -10 to 10 Check if the current x and y satisfy both equations. If so, output the solution, and finish. Ex: If no solution is found, output: No solution You can assume the two equations have no more than one solution. Note: Elegant mathematical techniques exist to solve such linear equations. However, for other kinds of equations or situations, brute force can be handy. LAB ACTIVITY 4.17.1: LAB: Brute force equation solver 0/28
4.17 LAB: Brute force equation solver Numerous engineering and scientific applications require finding solutions to a set of equations. Ex: 8x + 7y=38 and 3x - 5y = -1 have a solution x = 3, y=2. Given integer coefficients of two linear equations with variables x and y, use brute force to find an integer solution for x and y in the range-10 to 10. Ex: If the input is: 87 38 3 -5 -1 the output is: 32 because 8x3 +7x2-38 and 3x3 +-5x2=-1. Use this brute force approach: For every value of x from -10 to 10 For every value of y from -10 to 10 Check if the current x and y satisfy both equations. If so, output the solution, and finish. Ex: If no solution is found, output: No solution You can assume the two equations have no more than one solution. Note: Elegant mathematical techniques exist to solve such linear equations. However, for other kinds of equations or situations, brute force can be handy. LAB ACTIVITY 4.17.1: LAB: Brute force equation solver 0/28
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
In C++

Transcribed Image Text:---
**4.17 LAB: Brute force equation solver**
Numerous engineering and scientific applications require finding solutions to a set of equations. For example:
8x + 7y = 38 and 3x - 5y = -1
These equations have a solution x = 3, y = 2. Given integer coefficients of two linear equations with variables x and y, use brute force to find an integer solution for x and y in the range -10 to 10.
**Example:**
If the input is:
```
8 7 38
3 -5 -1
```
The output is:
```
3 2
```
because 8*3 + 7*2 = 38 and 3*3 + -5*2 = -1.
**Use this brute force approach:**
```
For every value of x from -10 to 10
For every value of y from -10 to 10
Check if the current x and y satisfy both equations. If so, output the solution, and finish.
```
**Example:**
If no solution is found, output:
```
No solution
```
You can assume the two equations have no more than one solution.
**Note:** Elegant mathematical techniques exist to solve such linear equations. However, for other kinds of equations or situations, brute force can be handy.
---
![Certainly! Below is a transcription of the image, formatted for an educational website context.
---
**LAB ACTIVITY**
**4.17.1: LAB: Brute force equation solver**
**main.cpp**
```cpp
#include <iostream>
using namespace std;
int main() {
/* Type your code here. */
return 0;
}
```
**Instructions:**
- You will develop a C++ program to solve equations using a brute force method. The provided code template is a starting point for your implementation.
**Interface:**
- **Develop mode**: Use this mode to continually work on your code.
- **Submit mode**: Use this mode to finalize and turn in your solution.
**Input Section:**
- Enter any required inputs for your program here. This is necessary if your code depends on specific inputs to run effectively.
**Execution Process:**
- Click the **Run program** button to compile and execute your code.
- The diagram below illustrates the execution flow:
- **Input (from above)** → **main.cpp (Your program)** → **Output (shown below)**
- Please note that the program output will be displayed in the designated area beneath the run button.
**Program Output:**
- The results of your program execution will be displayed here for review.
**Coding trail of your work:** [What is this?]
---
This setup is designed to facilitate your understanding of how to implement, test, and submit solutions effectively for automated grading. Good luck with your coding and problem-solving!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fda17b6d9-f7ff-4a56-9763-458bee0c35b1%2Fcde3e929-f441-45ee-afb4-1be9892a5cf2%2Fs5pcm0q_processed.png&w=3840&q=75)
Transcribed Image Text:Certainly! Below is a transcription of the image, formatted for an educational website context.
---
**LAB ACTIVITY**
**4.17.1: LAB: Brute force equation solver**
**main.cpp**
```cpp
#include <iostream>
using namespace std;
int main() {
/* Type your code here. */
return 0;
}
```
**Instructions:**
- You will develop a C++ program to solve equations using a brute force method. The provided code template is a starting point for your implementation.
**Interface:**
- **Develop mode**: Use this mode to continually work on your code.
- **Submit mode**: Use this mode to finalize and turn in your solution.
**Input Section:**
- Enter any required inputs for your program here. This is necessary if your code depends on specific inputs to run effectively.
**Execution Process:**
- Click the **Run program** button to compile and execute your code.
- The diagram below illustrates the execution flow:
- **Input (from above)** → **main.cpp (Your program)** → **Output (shown below)**
- Please note that the program output will be displayed in the designated area beneath the run button.
**Program Output:**
- The results of your program execution will be displayed here for review.
**Coding trail of your work:** [What is this?]
---
This setup is designed to facilitate your understanding of how to implement, test, and submit solutions effectively for automated grading. Good luck with your coding and problem-solving!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
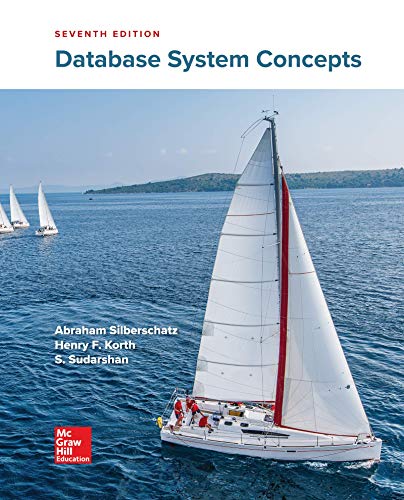
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
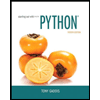
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
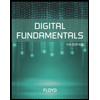
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
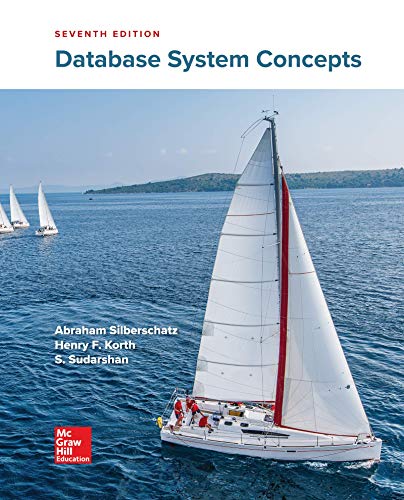
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
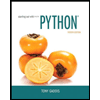
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
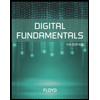
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
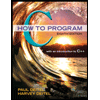
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
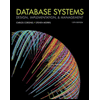
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
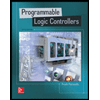
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education