4.13 LAB: Car value (classes) Instructor note: Important Coding Guidelines: Use comments, and whitespaces around operators and assignments. Use line breaks and indent your code. Use naming conventions for variables, functions, methods, and more. This makes it easier to understand the code. Write simple code and do not over complicate the logic. Code exhibits simplicity when it’s well organized, logically minimal, and easily readable. C++ Given main(), complete the Car class (in files Car.h and Car.cpp) with member functions to set and get the purchase price of a car (SetPurchasePrice(), GetPurchasePrice()), and to output the car's information (PrintInfo()). Ex: If the input is: 2011 18000 2018 where 2011 is the car's model year, 18000 is the purchase price, and 2018 is the current year, the output is: Car's information: Model year: 2011 Purchase price: 18000 Current value: 5770 #include #include #include "Car.h" using namespace std; void Car::SetModelYear(int userYear){ modelYear = userYear; } int Car::GetModelYear() const { return modelYear; } int setPurchasePrice() { return price;// TODO: Implement SetPurchasePrice() function } }; int getPurchasePrice() { return price; } };// TODO: Implement GetPurchasePrice() function void Car::CalcCurrentValue(int currentYear) { double depreciationRate = 0.15; int carAge = currentYear - modelYear; // Car depreciation formula currentValue = (int) round(purchasePrice * pow((1 - depreciationRate), carAge)); } // TODO: Implement PrintInfo() function to output modelYear, purchasePrice, and // currentValue #ifndef CARH #define CARH class Car { private: int modelYear; // TODO: Declare purchasePrice member (int) int currentValue; public: void SetModelYear(int userYear); int GetModelYear() const; // TODO: Declare SetPurchasePrice() function // TODO: Declare GetPurchasePrice() function void CalcCurrentValue(int currentYear); // TODO: Declare PrintInfo() method to output modelYear, purchasePrice, and // currentValue }; #endif
-
4.13 LAB: Car value (classes)
Instructor note:Important Coding Guidelines:
- Use comments, and whitespaces around operators and assignments.
- Use line breaks and indent your code.
- Use naming conventions for variables, functions, methods, and more. This makes it easier to understand the code.
- Write simple code and do not over complicate the logic. Code exhibits simplicity when it’s well organized, logically minimal, and easily readable.
C++
Given main(), complete the Car class (in files Car.h and Car.cpp) with member functions to set and get the purchase price of a car (SetPurchasePrice(), GetPurchasePrice()), and to output the car's information (PrintInfo()).
Ex: If the input is:
2011 18000 2018where 2011 is the car's model year, 18000 is the purchase price, and 2018 is the current year, the output is:
Car's information: Model year: 2011 Purchase price: 18000 Current value: 5770#include <iostream>
#include <math.h>
#include "Car.h"
using namespace std;void Car::SetModelYear(int userYear){
modelYear = userYear;
}int Car::GetModelYear() const {
return modelYear;
}int setPurchasePrice() {
return price;// TODO: Implement SetPurchasePrice() function
}
};
int getPurchasePrice() {
return price;
}
};// TODO: Implement GetPurchasePrice() functionvoid Car::CalcCurrentValue(int currentYear) {
double depreciationRate = 0.15;
int carAge = currentYear - modelYear;// Car depreciation formula
currentValue = (int)
round(purchasePrice * pow((1 - depreciationRate), carAge));
}// TODO: Implement PrintInfo() function to output modelYear, purchasePrice, and
// currentValue#ifndef CARH
#define CARHclass Car {
private:
int modelYear;
// TODO: Declare purchasePrice member (int)
int currentValue;public:
void SetModelYear(int userYear);int GetModelYear() const;
// TODO: Declare SetPurchasePrice() function
// TODO: Declare GetPurchasePrice() function
void CalcCurrentValue(int currentYear);
// TODO: Declare PrintInfo() method to output modelYear, purchasePrice, and
// currentValue};
#endif

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

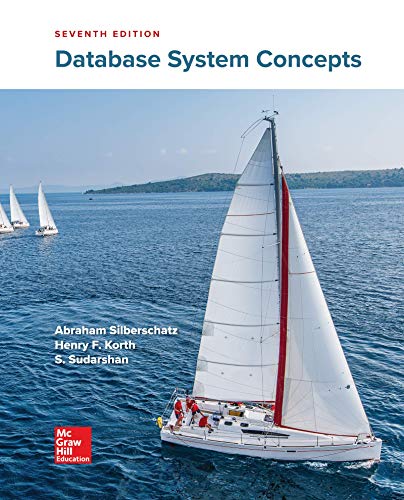
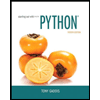
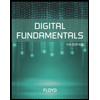
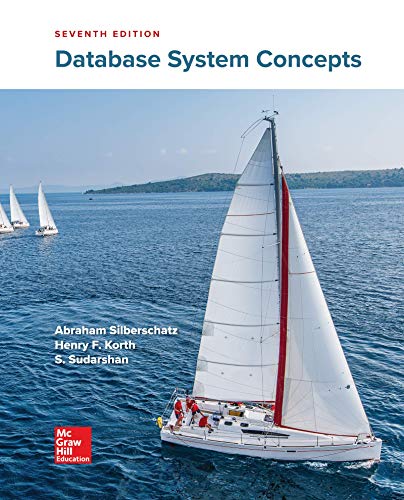
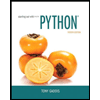
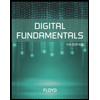
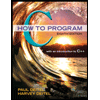
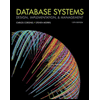
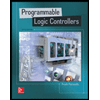