4. Write a function that adds two polynomials. Ensure that the polynomial produced matches the requirements. addPoly :: (Num a, Eg a) => Poly a -> Poly a -> Poly a For example: > addPoly (P [1]) (P [-1,1]) P [0,1] > addPoly (P [17]) (P [0,0,0,1]) P [17,0,0,1] >addPoly (P [1,-1]) (P [0,1]) P [1] You may find it helpful to write addPoly using two helper functions: one to combine the lists of coefficients, and one to trim trailing zeroes. Your implementation of addPoly should have this property: addPoly p q $$ x == (p sS x) + (a Ss x)
4. Write a function that adds two polynomials. Ensure that the polynomial produced matches the requirements. addPoly :: (Num a, Eg a) => Poly a -> Poly a -> Poly a For example: > addPoly (P [1]) (P [-1,1]) P [0,1] > addPoly (P [17]) (P [0,0,0,1]) P [17,0,0,1] >addPoly (P [1,-1]) (P [0,1]) P [1] You may find it helpful to write addPoly using two helper functions: one to combine the lists of coefficients, and one to trim trailing zeroes. Your implementation of addPoly should have this property: addPoly p q $$ x == (p sS x) + (a Ss x)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Has to be in HASKELL
![### Adding Two Polynomials
To write a function that adds two polynomials, ensure that the resulting polynomial meets the specified requirements.
#### Function Signature
```haskell
addPoly :: (Num a, Eq a) => Poly a -> Poly a -> Poly a
```
#### Example Usage
- **Example 1:**
```haskell
> addPoly (P [1]) (P [-1,1])
P [0,1]
```
- **Example 2:**
```haskell
> addPoly (P [17]) (P [0,0,0,1])
P [17,0,0,1]
```
- **Example 3:**
```haskell
> addPoly (P [1,-1]) (P [0,1])
P [1]
```
#### Implementation Suggestions
Consider writing `addPoly` using two helper functions:
1. One to combine the lists of coefficients.
2. One to trim trailing zeroes.
#### Property of addPoly
Your implementation of `addPoly` should satisfy the following property:
```haskell
addPoly p q $$ x == (p $$ x) + (q $$ x)
```
This property states that evaluating the sum of two polynomials at any value `x` should be equivalent to adding the results of evaluating each polynomial individually at `x`.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ff26fda3f-4323-495f-b28b-f131dcfa5992%2F0c57ab72-f084-4588-aec5-d1510852e241%2Fwaq4f8d_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Adding Two Polynomials
To write a function that adds two polynomials, ensure that the resulting polynomial meets the specified requirements.
#### Function Signature
```haskell
addPoly :: (Num a, Eq a) => Poly a -> Poly a -> Poly a
```
#### Example Usage
- **Example 1:**
```haskell
> addPoly (P [1]) (P [-1,1])
P [0,1]
```
- **Example 2:**
```haskell
> addPoly (P [17]) (P [0,0,0,1])
P [17,0,0,1]
```
- **Example 3:**
```haskell
> addPoly (P [1,-1]) (P [0,1])
P [1]
```
#### Implementation Suggestions
Consider writing `addPoly` using two helper functions:
1. One to combine the lists of coefficients.
2. One to trim trailing zeroes.
#### Property of addPoly
Your implementation of `addPoly` should satisfy the following property:
```haskell
addPoly p q $$ x == (p $$ x) + (q $$ x)
```
This property states that evaluating the sum of two polynomials at any value `x` should be equivalent to adding the results of evaluating each polynomial individually at `x`.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Recommended textbooks for you
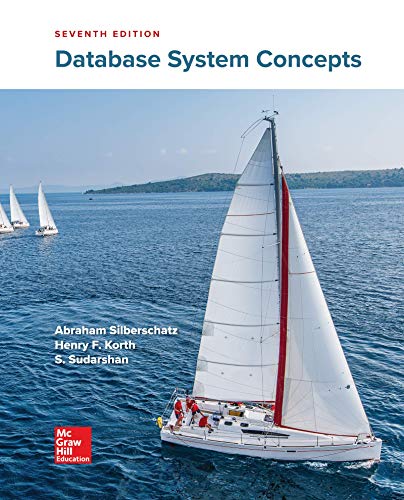
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
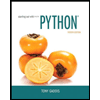
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
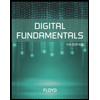
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
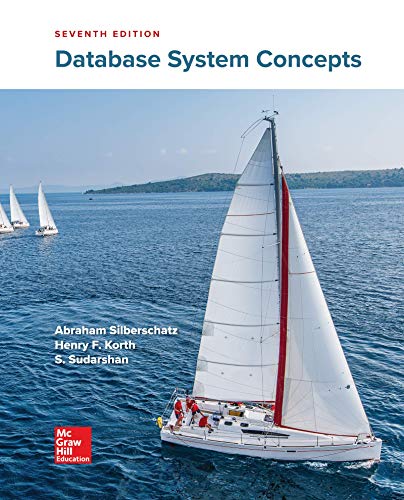
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
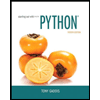
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
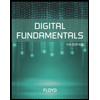
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
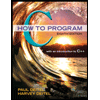
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
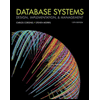
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
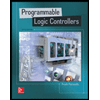
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education