2.15 LAB: Using math functions Given three floating-point numbers x, y, and z, output x to the power of z, x to the power of (y to the power of z), the absolute value of (x minus y), and the square root of (x to the power of z). Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print('{:.2f} {:.2f} {:.2f} {:.2f}'.format(your_value1, your_value2, your_value3, your_value4)) Ex: If the input is: 5.0 1.5 3.2 Then the output is: 172.47 361.66 3.50 13.13 317624.1960076.qx3zqy7 LAB 2.15.1: LAB: Using math functions 0/ 10 ACTIVITY main.py Load default template... 1 import math Type your code here. ''|
2.15 LAB: Using math functions Given three floating-point numbers x, y, and z, output x to the power of z, x to the power of (y to the power of z), the absolute value of (x minus y), and the square root of (x to the power of z). Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print('{:.2f} {:.2f} {:.2f} {:.2f}'.format(your_value1, your_value2, your_value3, your_value4)) Ex: If the input is: 5.0 1.5 3.2 Then the output is: 172.47 361.66 3.50 13.13 317624.1960076.qx3zqy7 LAB 2.15.1: LAB: Using math functions 0/ 10 ACTIVITY main.py Load default template... 1 import math Type your code here. ''|
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Python

Transcribed Image Text:# LAB 2.15: Using Math Functions
## Exercise Overview
Given three floating-point numbers \( x \), \( y \), and \( z \), this lab requires you to output:
1. \( x \) raised to the power of \( z \)
2. \( x \) raised to the power of \( (y \) raised to the power of \( z \))
3. The absolute value of \( (x - y) \)
4. The square root of \( (x \) raised to the power of \( z \))
Each floating-point value should be formatted to two decimal places. You can achieve this formatting with the code:
```python
print('{:.2f} {:.2f} {:.2f} {:.2f}'.format(your_value1, your_value2, your_value3, your_value4))
```
### Example
If the input values are:
```
5.0
1.5
3.2
```
Then the output should be:
```
172.47 361.66 3.50 13.13
```
## Starting Code
```python
import math
'''Type your code here.'''
```
Use the code template to write your solution, and remember to use the math functions provided by the Python standard library.
## LAB Activity
### Activity 2.15.1: LAB: Using Math Functions
#### Instructions
1. **Complete the script**: Implement the solution within the provided code template.
2. **Run the script**: Test it with different inputs to ensure it works as expected.
3. **Verify Output**: Ensure the output matches the required format with two decimal places.
You can load the default code template to start working on the problem. Good luck and happy coding!
## Summary
Understanding and using math functions in Python can be very powerful for a wide range of applications. This exercise helps you practice working with powers, absolute values, and square roots, while also focusing on formatting output to a specified precision.

Transcribed Image Text:## Lab Activity: Using Math Functions
### Overview
In this lab, you will work with math functions in a Python program. You will learn how to import the math module and how to use its functions to perform various mathematical operations.
### Instructions
1. **Code Editor (main.py)**:
- The provided code editor displays a code file named `main.py`.
- It contains an import statement for the `math` module and a placeholder for your code.
- Here is how it looks:
```python
import math
''' Type your code here. '''
```
2. **Develop/Submit Mode**:
- **Develop mode**: Allows you to run your program as often as needed before submitting it for grading.
- **Submit mode**: Use this mode to submit your completed code for grading.
3. **Program Input**:
- If your code requires input values, you can provide them in the "Enter program input (optional)" text box.
- These input values will be used when you run the program.
4. **Running the Program**:
- Click on the **Run program** button to execute your code.
- Your code will read input (if any) from the provided input box, process it using `main.py`, and produce output.
### Example Input and Output Flow
The diagram below represents the flow of input and output in the program:
```
Input (from above) --> main.py (Your program) --> Output (shown below)
```
### Initial Setup
- Begin by exploring basic functions from the `math` module such as `math.sqrt()`, `math.sin()`, `math.cos()`, and more.
- Write your code inside `main.py` to perform calculations or processes as required by your lab tasks.
### Example Task
Suppose you want to compute the square root of a number:
In `main.py`, you would write:
```python
import math
# Example: Calculate square root
number = float(input("Enter a number: "))
sqrt_value = math.sqrt(number)
print(f"The square root of {number} is {sqrt_value}")
```
Provide the input in the "Enter program input (optional)" box, for example:
```
25
```
When you click **Run program**, the output will be displayed showing:
```
The square root of 25 is 5.0
```
### Additional Information
You can
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
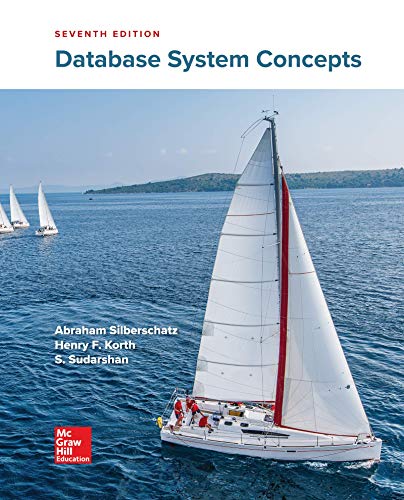
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
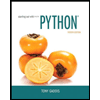
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
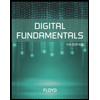
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
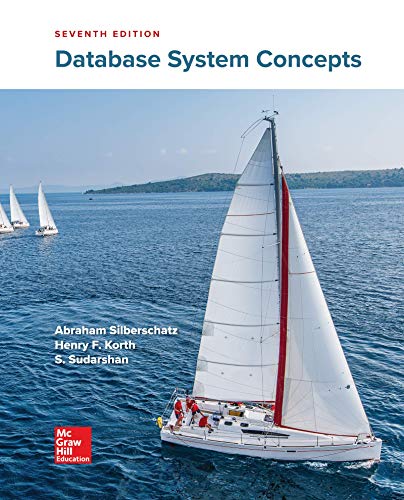
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
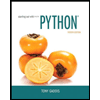
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
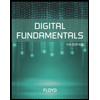
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
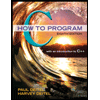
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
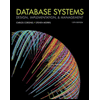
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
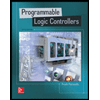
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education