2. Now implement the other bottom method generateCharacters. The method takes as input (param- eters) the staring and ending numbers of a sequence of contiguous Unicode-characters we want to generate. The method concatenates all the characters into a String and returns the String. public static String generateChars(int ch1, int ch2) { String chars = ""; for ( int i = ch1; i < ch2; ++i) chars += (char) 30 31 E 32 33 %3D 34 35 return chars; } 36 37 3. Inside main(), comment out the call to method menu() and test the method generateChars by call- ing as follows: String s = generateChars( 65 , 65+26); System.out.printIn( s ); Test the method for Greek and Cyrillic. Note that the Cyrillic alphabet starts at 410 in hex, and has 32 characters. Note that the Greek Alphabet starts at 391 in hex, and has 24 characters. However, use +25 instead of +24, as one of the characters in the sequence is not a Greek letter.
2. Now implement the other bottom method generateCharacters. The method takes as input (param- eters) the staring and ending numbers of a sequence of contiguous Unicode-characters we want to generate. The method concatenates all the characters into a String and returns the String. public static String generateChars(int ch1, int ch2) { String chars = ""; for ( int i = ch1; i < ch2; ++i) chars += (char) 30 31 E 32 33 %3D 34 35 return chars; } 36 37 3. Inside main(), comment out the call to method menu() and test the method generateChars by call- ing as follows: String s = generateChars( 65 , 65+26); System.out.printIn( s ); Test the method for Greek and Cyrillic. Note that the Cyrillic alphabet starts at 410 in hex, and has 32 characters. Note that the Greek Alphabet starts at 391 in hex, and has 24 characters. However, use +25 instead of +24, as one of the characters in the sequence is not a Greek letter.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Java Netbeans

Transcribed Image Text:### Implementing the `generateCharacters` Method
#### Step-by-Step Guide
1. **Objective**:
The method `generateCharacters` is designed to generate a sequence of contiguous Unicode characters. The method receives two integer parameters representing the starting and ending Unicode values and returns a concatenated string of the characters.
2. **Code Implementation**:
```java
public static String generateChars(int ch1, int ch2) {
String chars = "";
for (int i = ch1; i < ch2; ++i) {
chars += (char) i + " ";
}
return chars;
}
```
- **Lines 31-37**:
- **Line 31**: Declaration of the `generateChars` method with input parameters `ch1` and `ch2`.
- **Line 32**: Initializes an empty string `chars`.
- **Lines 33-34**: A `for` loop iterates from `ch1` to `ch2`, casting each integer `i` to a character, appending it to the `chars` string followed by a space.
- **Line 36**: Returns the concatenated string of characters.
3. **Testing in `main()`**:
- **Instructions**:
- Comment out the existing call to the method `menu()`.
- Test the `generateChars` method by invoking:
```java
String s = generateChars(65, 65+26);
System.out.println(s);
```
#### Special Notes for Greek and Cyrillic Alphabets
- **Cyrillic Alphabet**:
- Begins at Unicode `410` in hexadecimal.
- Contains 32 characters.
- **Greek Alphabet**:
- Begins at Unicode `391` in hexadecimal.
- Contains 24 characters.
- Use `+25` instead of `+24` when specifying the range due to a non-Greek character present in the sequence.
This method enables generating a sequence of characters for diverse alphabets by altering the Unicode range parameters.

Transcribed Image Text:The bottom method `menu()` of the diagram in the previous page is implemented first. This is why it is called Bottom Up Design. Type in the code as shown below and then test it from main by calling it.
```java
public class Lab15
{
public static String menu()
{
String s = "";
do
{
System.out.println("\n\t0 to quit this program ");
System.out.println("\t1 --> Latin alphabet capitals ");
System.out.println("\t2 --> Latin alphabet lower case ");
System.out.println("\t3 --> Cyrillic alphabet capitals ");
System.out.println("\t4 --> Cyrillic alphabet lower case ");
System.out.println("\t5 --> Greek alphabet capitals ");
System.out.println("\t6 --> Greek alphabet lower case ");
System.out.print("your choice -------->");
s = new Scanner( System.in ).next();
if ( s.compareTo("0") < 0 || s.compareTo("6") > 0 )
System.out.println("\tplease type 0 to 6" );
}
while (s.compareTo("0") < 0 || s.compareTo("6") > 0 );
return s;
}
}
```
**Explanation:**
This Java code defines a method called `menu()` within a public class named `Lab15`. The `menu()` method:
- Displays a menu to the user, offering choices to display different alphabet scripts and a quit option.
- Accepts user input via the `Scanner` class.
- Validates the input to ensure it is between 0 and 6.
- Returns the user's choice as a string once a valid input is received.
Expert Solution

Basic
Call the generate char function from the main method to get results.
Solution below.
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
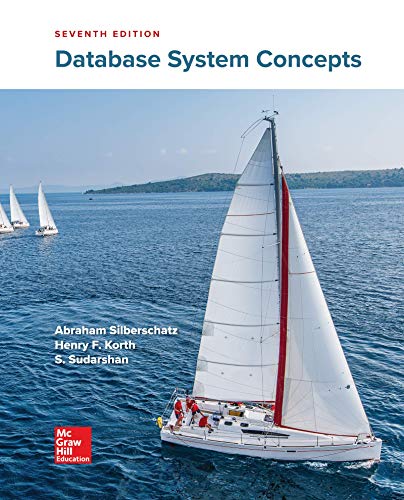
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
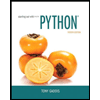
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
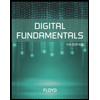
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
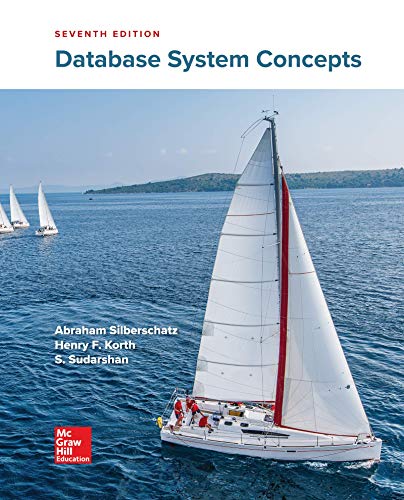
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
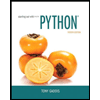
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
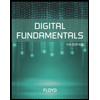
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
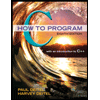
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
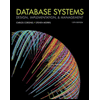
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
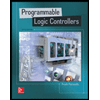
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education