2. Each of the following programs contains exactly one syntax error. Find the error and correct it. a) public static void main(String[] args) { BankAsseunt a = new BankAsSeunt (500); Bankacseunt b = new Bankacseuet () ; double c Sxsten.eut intin( "The combined balance is $" + c); b) public static void main(String[] args) Bankaeseunt a = new BaakāEseurt (500); a += 300; { Sxsten.eut intln( "The new balance is $" + augetkalance () ) ; c) public static void main(String[] args) BankAeseuet a Fnderesit (500); GuBaakAEseunt () ; Sxstem.eutuRKintle (argetNane () + { = new BankaEGRUrt (100); now has a 0 balance");
2. Each of the following programs contains exactly one syntax error. Find the error and correct it. a) public static void main(String[] args) { BankAsseunt a = new BankAsSeunt (500); Bankacseunt b = new Bankacseuet () ; double c Sxsten.eut intin( "The combined balance is $" + c); b) public static void main(String[] args) Bankaeseunt a = new BaakāEseurt (500); a += 300; { Sxsten.eut intln( "The new balance is $" + augetkalance () ) ; c) public static void main(String[] args) BankAeseuet a Fnderesit (500); GuBaakAEseunt () ; Sxstem.eutuRKintle (argetNane () + { = new BankaEGRUrt (100); now has a 0 balance");
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
JAVA
need help with question 2

Transcribed Image Text:1. The Bankdccount class models changes to the balance of a person's savings account.
public class Bankacseuet
{
public BankaAcseunt ( double initbah)
public double getRalanse ( )
public String getNang ()
public void deposit (double amount)
public void withdraw (double amount)
public void ttansfexte (BaakāesLuet atheKAset, double amt)
public boglean equals (Object other)
private String asstlane;
private double balance;
}
a) Provide the code for the transferTo(...) method.
balance = balance + amt;
QtherAcct.withdraw(amt);
}
![2. Each of the following programs contains exactly one syntax error. Find the error and correct it.
a) public static void main (String[] args)
{
BankAeseunt a
= new BankAESeurt (500);
BankAcseunt b = new BankAcSQunt () ;
double c =
Sxsteneutrrintin( "The combined balance is
$" + c);
b) public static void main (String[] args)
Baakaeseurt a = new BaakAeseurt (500);
a += 300;
{
Sxsteneut iatle( "The new balance is
$" + augetRalanse () ) ;
}
c) public static void main (String[] args)
= new BankAcseunt (100);
BankAeseunt a
auderesit (500);
aBRakAEseunt, () ;
SYstameutuKİntlz (argetNane ( )
{
%3D
now has a 0 balance");](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fb4deeaa2-280e-4afd-b08f-ce2df53e06a4%2F71ae118c-e7f0-4f5c-a695-7f6d391dd19a%2Fgt7wzfe_processed.png&w=3840&q=75)
Transcribed Image Text:2. Each of the following programs contains exactly one syntax error. Find the error and correct it.
a) public static void main (String[] args)
{
BankAeseunt a
= new BankAESeurt (500);
BankAcseunt b = new BankAcSQunt () ;
double c =
Sxsteneutrrintin( "The combined balance is
$" + c);
b) public static void main (String[] args)
Baakaeseurt a = new BaakAeseurt (500);
a += 300;
{
Sxsteneut iatle( "The new balance is
$" + augetRalanse () ) ;
}
c) public static void main (String[] args)
= new BankAcseunt (100);
BankAeseunt a
auderesit (500);
aBRakAEseunt, () ;
SYstameutuKİntlz (argetNane ( )
{
%3D
now has a 0 balance");
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
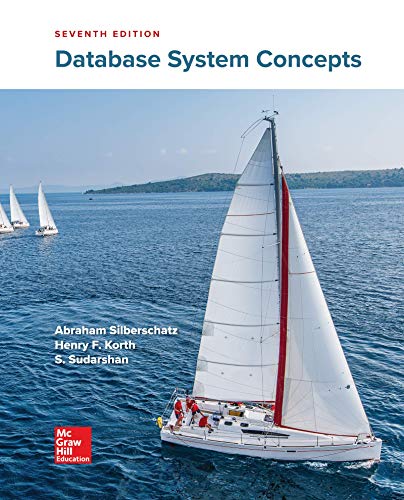
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
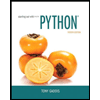
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
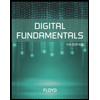
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
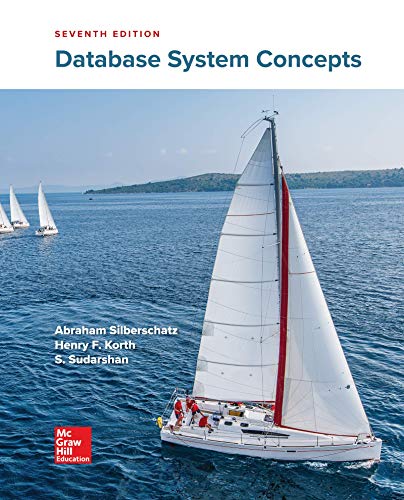
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
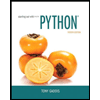
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
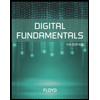
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
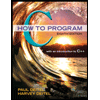
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
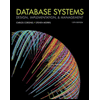
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
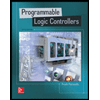
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education