2. A stack can be used to print numbers in other bases (multibase output). Examples: a) (Bases) 2810 = 3 * 8¹ + 4* 8º = 348 b) (Base 4) 7210 = 1* 4³ + 0* 4² + 2 * 4¹ + 0* 4 = 10204 c) (Base 2) 5310 = 1* 25 + 1*24 + 0*2³ +1* 2² +0* 2¹ + 1* 2⁰ = 110101₂ Write a java program using stacks that takes 3 non-negative (base 10) long integer numbers and a base B (B is in the range 2-9) and writes the number to the screen as a base B number. The program prompts the user for 3 numbers and bases, and then outputs them. Use as input: a) 7210 b) 5310 c) 355310 Base 4 Base 2 Base 8
2. A stack can be used to print numbers in other bases (multibase output). Examples: a) (Bases) 2810 = 3 * 8¹ + 4* 8º = 348 b) (Base 4) 7210 = 1* 4³ + 0* 4² + 2 * 4¹ + 0* 4 = 10204 c) (Base 2) 5310 = 1* 25 + 1*24 + 0*2³ +1* 2² +0* 2¹ + 1* 2⁰ = 110101₂ Write a java program using stacks that takes 3 non-negative (base 10) long integer numbers and a base B (B is in the range 2-9) and writes the number to the screen as a base B number. The program prompts the user for 3 numbers and bases, and then outputs them. Use as input: a) 7210 b) 5310 c) 355310 Base 4 Base 2 Base 8
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The first image is the main question, the second image is the directions for the
![### Using Stacks to Print Numbers in Various Bases
Stacks can be used to print numbers in other bases (multibase output). Here are some examples to demonstrate this concept:
#### Examples:
**a) (Base<sub>8</sub>)**
\[ 28_{10} = 3 \times 8^1 + 4 \times 8^0 = 34_{8} \]
**b) (Base<sub>4</sub>)**
\[ 72_{10} = 1 \times 4^3 + 0 \times 4^2 + 2 \times 4^1 + 0 \times 4^0 = 1020_{4} \]
**c) (Base<sub>2</sub>)**
\[ 53_{10} = 1 \times 2^5 + 1 \times 2^4 + 0 \times 2^3 + 1 \times 2^2 + 0 \times 2^1 + 1 \times 2^0 = 110101_{2} \]
#### Task:
Write a Java program using stacks that takes 3 non-negative (base 10) long integer numbers and a base B (where B is in the range 2-9), and converts each number to its representation in base B. The program should prompt the user to input the numbers and the desired bases, then output the converted numbers.
#### Input Examples:
1. \( 72_{10} \) in Base 4
2. \( 53_{10} \) in Base 2
3. \( 355_{10} \) in Base 8
By following these examples and using the provided task description, you can create a versatile program to convert decimal numbers into various bases.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd3ed3f65-79b2-4bdf-866b-dcdc9cf257bd%2F65a64ffc-3d6d-4089-85fd-8b54336526d4%2F178ya5_processed.png&w=3840&q=75)
Transcribed Image Text:### Using Stacks to Print Numbers in Various Bases
Stacks can be used to print numbers in other bases (multibase output). Here are some examples to demonstrate this concept:
#### Examples:
**a) (Base<sub>8</sub>)**
\[ 28_{10} = 3 \times 8^1 + 4 \times 8^0 = 34_{8} \]
**b) (Base<sub>4</sub>)**
\[ 72_{10} = 1 \times 4^3 + 0 \times 4^2 + 2 \times 4^1 + 0 \times 4^0 = 1020_{4} \]
**c) (Base<sub>2</sub>)**
\[ 53_{10} = 1 \times 2^5 + 1 \times 2^4 + 0 \times 2^3 + 1 \times 2^2 + 0 \times 2^1 + 1 \times 2^0 = 110101_{2} \]
#### Task:
Write a Java program using stacks that takes 3 non-negative (base 10) long integer numbers and a base B (where B is in the range 2-9), and converts each number to its representation in base B. The program should prompt the user to input the numbers and the desired bases, then output the converted numbers.
#### Input Examples:
1. \( 72_{10} \) in Base 4
2. \( 53_{10} \) in Base 2
3. \( 355_{10} \) in Base 8
By following these examples and using the provided task description, you can create a versatile program to convert decimal numbers into various bases.
![---
## Program #2
1. **Show the `ListStackADT<T>` Interface**
2. **Create a `ListStackDataStrucClass<T>` with the following methods:**
- Default constructor
- Overloaded constructor
- Copy constructor
- `getTop`
- `setTop`
- `isEmpty`
- `ifEmpty` (if empty throw the exception)
- `push`
- `peek`
- `pop`
- `toString`
3. **Create a private inner class of `ListStack<T>` called `StackNode<T>` with the following methods:**
- Default constructor
- Overloaded constructor
- Copy constructor
- `getValue`
- `getNext`
- `setValue`
- `setNext`
4. **Create a `BaseConverter` class (non-generic) with the following methods:**
- Default constructor
- `inputPrompt`
- `convert` [converts a `BaseNumber` to a converted `String`]
- `convertAll` [instantiate a `String` object]
- `toString`
- `processAndPrint`
5. **Create a private inner class `BaseNumber`. The inner class has the following methods:**
- Default constructor
- Overloaded constructor
- `getNumber`
- `getBase`
- `setNumber`
- `setBase`
*Note: Make your private instance variables in the inner class `Long` type.*
6. **Create a `BaseConverterDemo` class that only has 2 statements:**
- Create a `BaseConverter` object
- Have it invoke `processAndPrint`
7. **Exception classes: `StackException`, `EmptyStackException`, `FullStackException`**
---](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd3ed3f65-79b2-4bdf-866b-dcdc9cf257bd%2F65a64ffc-3d6d-4089-85fd-8b54336526d4%2Fye15y8d_processed.png&w=3840&q=75)
Transcribed Image Text:---
## Program #2
1. **Show the `ListStackADT<T>` Interface**
2. **Create a `ListStackDataStrucClass<T>` with the following methods:**
- Default constructor
- Overloaded constructor
- Copy constructor
- `getTop`
- `setTop`
- `isEmpty`
- `ifEmpty` (if empty throw the exception)
- `push`
- `peek`
- `pop`
- `toString`
3. **Create a private inner class of `ListStack<T>` called `StackNode<T>` with the following methods:**
- Default constructor
- Overloaded constructor
- Copy constructor
- `getValue`
- `getNext`
- `setValue`
- `setNext`
4. **Create a `BaseConverter` class (non-generic) with the following methods:**
- Default constructor
- `inputPrompt`
- `convert` [converts a `BaseNumber` to a converted `String`]
- `convertAll` [instantiate a `String` object]
- `toString`
- `processAndPrint`
5. **Create a private inner class `BaseNumber`. The inner class has the following methods:**
- Default constructor
- Overloaded constructor
- `getNumber`
- `getBase`
- `setNumber`
- `setBase`
*Note: Make your private instance variables in the inner class `Long` type.*
6. **Create a `BaseConverterDemo` class that only has 2 statements:**
- Create a `BaseConverter` object
- Have it invoke `processAndPrint`
7. **Exception classes: `StackException`, `EmptyStackException`, `FullStackException`**
---
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 13 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
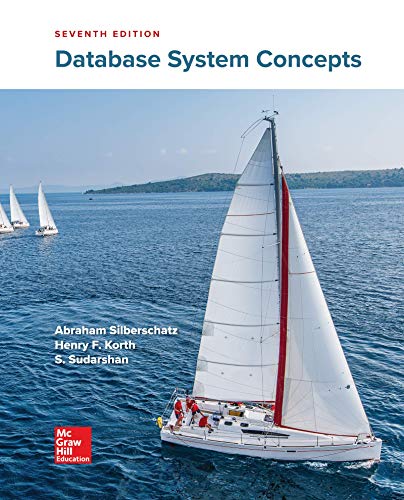
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
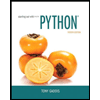
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
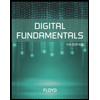
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
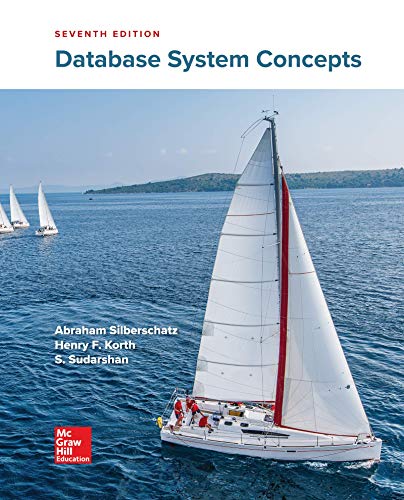
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
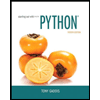
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
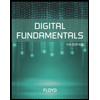
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
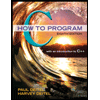
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
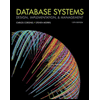
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
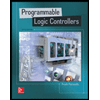
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education