2: write code to draw the following picture 9 first 8 second 7 7 6 6 55 5 4 3 2 1 O 5 Mon 33 Tue Wed 5 Thur 44 Fri 5 Sat
2: write code to draw the following picture 9 first 8 second 7 7 6 6 55 5 4 3 2 1 O 5 Mon 33 Tue Wed 5 Thur 44 Fri 5 Sat
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Use pyt
![---
### Exercise 2: Write Code to Draw the Following Picture
In this exercise, you are asked to write code to generate a bar chart that looks like the one shown in the image. Below is a detailed explanation of the graph.
#### Graph Description:
- The chart is a clustered bar graph displaying data for two categories (labeled "first" and "second") over a week (from Monday to Saturday).
- The y-axis represents values ranging from 0 to 9.
- The x-axis lists the days of the week (Mon, Tue, Wed, Thur, Fri, Sat).
#### Data Representation:
- The "first" category is represented by green bars.
- The "second" category is represented by pink bars.
#### Values for Each Day:
- **Monday**: 6 (first), 5 (second)
- **Tuesday**: 3 (first), 3 (second)
- **Wednesday**: 5 (first), 5 (second)
- **Thursday**: 7 (first), 5 (second)
- **Friday**: 4 (first), 4 (second)
- **Saturday**: 6 (first), 5 (second)
#### Legend:
- There's a legend in the top right corner of the graph indicating which color corresponds to which category:
- Green: "first"
- Pink: "second"
To complete this exercise, you will need to use a programming language that supports graph plotting, like Python with the Matplotlib library, to replicate this bar graph.
The code snippet below provides a basic structure using Python's Matplotlib library:
```python
import matplotlib.pyplot as plt
import numpy as np
# Data
days = ['Mon', 'Tue', 'Wed', 'Thur', 'Fri', 'Sat']
first = [6, 3, 5, 7, 4, 6]
second = [5, 3, 5, 5, 4, 5]
# Position of bars on x-axis
x = np.arange(len(days))
# Width of a bar
width = 0.4
# Plotting the bars
fig, ax = plt.subplots()
bar1 = ax.bar(x - width/2, first, width, label='first', color='green')
bar2 = ax.bar(x + width/2, second, width, label='second', color='pink')
# Adding the legend](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fce5cea56-9cd3-471d-b739-11e3ad279d5e%2F91c64f8b-95be-4c01-b44c-9e16934559b7%2Fg9hf3ks_processed.png&w=3840&q=75)
Transcribed Image Text:---
### Exercise 2: Write Code to Draw the Following Picture
In this exercise, you are asked to write code to generate a bar chart that looks like the one shown in the image. Below is a detailed explanation of the graph.
#### Graph Description:
- The chart is a clustered bar graph displaying data for two categories (labeled "first" and "second") over a week (from Monday to Saturday).
- The y-axis represents values ranging from 0 to 9.
- The x-axis lists the days of the week (Mon, Tue, Wed, Thur, Fri, Sat).
#### Data Representation:
- The "first" category is represented by green bars.
- The "second" category is represented by pink bars.
#### Values for Each Day:
- **Monday**: 6 (first), 5 (second)
- **Tuesday**: 3 (first), 3 (second)
- **Wednesday**: 5 (first), 5 (second)
- **Thursday**: 7 (first), 5 (second)
- **Friday**: 4 (first), 4 (second)
- **Saturday**: 6 (first), 5 (second)
#### Legend:
- There's a legend in the top right corner of the graph indicating which color corresponds to which category:
- Green: "first"
- Pink: "second"
To complete this exercise, you will need to use a programming language that supports graph plotting, like Python with the Matplotlib library, to replicate this bar graph.
The code snippet below provides a basic structure using Python's Matplotlib library:
```python
import matplotlib.pyplot as plt
import numpy as np
# Data
days = ['Mon', 'Tue', 'Wed', 'Thur', 'Fri', 'Sat']
first = [6, 3, 5, 7, 4, 6]
second = [5, 3, 5, 5, 4, 5]
# Position of bars on x-axis
x = np.arange(len(days))
# Width of a bar
width = 0.4
# Plotting the bars
fig, ax = plt.subplots()
bar1 = ax.bar(x - width/2, first, width, label='first', color='green')
bar2 = ax.bar(x + width/2, second, width, label='second', color='pink')
# Adding the legend
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
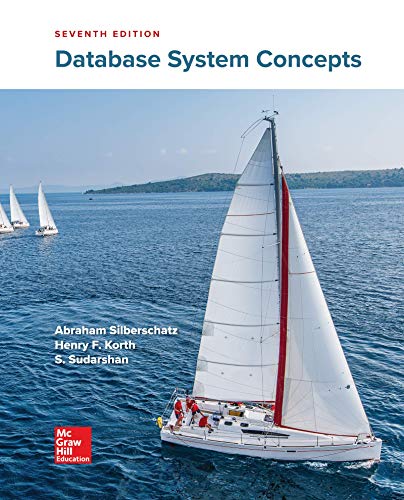
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
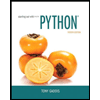
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
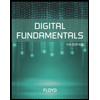
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
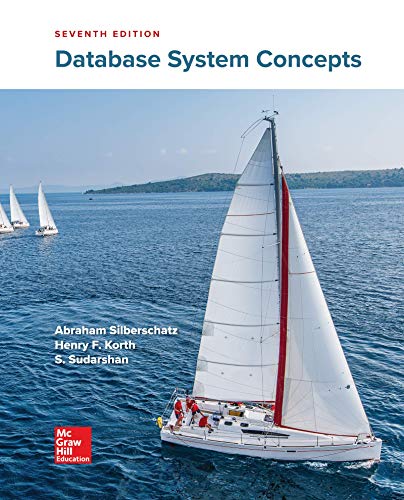
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
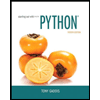
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
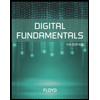
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
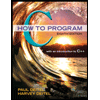
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
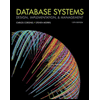
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
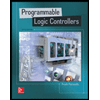
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education