2 questions in Java. all grey lines of code can not be edited. must edit inbetween grey blocks of code.
2 questions in Java. all grey lines of code can not be edited. must edit inbetween grey blocks of code.
![Jump to level 1
Given the integer array yearlyMiles with the size of NUM_ELEMENTS, write a for loop to output the integers in the first half of
yearlyMiles in reverse order. Separate the integers with a dash surrounded by spaces (" - ").
Ex: If the input is 110 46 31 69 103 83 70 60, then the output is:
69 31 46 110
1 import java.util.Scanner;
2
3 public class MileData {
4
5
7
8
9
10
11
12
13
14
15
16
17
18}
public static void main(String[] args) {
Scanner scnr = new Scanner (System.in);
final int NUM ELEMENTS = 8;
int[] yearlyMiles = new int[NUM_ELEMENTS];
int i;
}
for (i = 0; i < yearlyMiles.length; ++i) {
yearlyMiles [i] = scnr.nextInt ();
for
System.out.println();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0cc153ae-b205-4fb2-9991-7cf6a21d5016%2Fd7f9f763-834e-43ec-8893-367fa6026f05%2F2u6b99d_processed.png&w=3840&q=75)
![Jump to level 1
Integer numElements is read from input and integer array userScore is declared with size numElements. Then, numElements
integers are read from input and stored into userScore. Assign integer sumVals with the sum of the first element and the last
element of the array.
Ex: If the input is:
4
11 43 77 35
then the output is:
46
I Import Java.util.scanner;
2
3 public class ScoreLog {
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
public static void main(String[] args) {
Scanner scnr = new Scanner (System.in);
int numElements;
int[] userScore;
int i;
numElements = scnr. nextInt();
userScore = new int[numElements];
for (i = 0; i < userScore.length; ++i) {
userScore [i] = scnr.nextInt();
}
int sumVals = 0;
System.out.println(sumVals);
«
1
2
3](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0cc153ae-b205-4fb2-9991-7cf6a21d5016%2Fd7f9f763-834e-43ec-8893-367fa6026f05%2Fjqioelh_processed.png&w=3840&q=75)

Program - 1
Import the Scanner class to read input from the user.
Define the
MileData
class, which contains the main method where the program execution starts.Create a
Scanner
object namedscnr
to read input from the standard input (keyboard).Declare a constant
NUM_ELEMENTS
with a value of 8, which represents the number of elements in theyearlyMiles
array.Declare an integer array
yearlyMiles
with a length ofNUM_ELEMENTS
(8 in this case) to store the user's input.Declare an integer variable
i
to be used as a loop counter.Use a
for
loop to read integers from the user and store them in theyearlyMiles
array. The loop iterates from 0 toyearlyMiles.length - 1
(from 0 to 7) using the variablei
. Inside the loop, it usesscnr.nextInt()
to read an integer and stores it inyearlyMiles[i]
.After reading all the integers, use another
for
loop to print the integers in the first half of theyearlyMiles
array in reverse order. The loop starts from the middle of the array (NUM_ELEMENTS / 2 - 1
) and goes backward until it reaches the first element (0). Inside the loop, it prints each element with a hyphen separator. The conditionif (i > 0)
is used to avoid printing a hyphen after the last element.After printing all the integers in reverse order, move to a new line to separate the output.
Close the
main
method and theMileData
class.
Program - 2
- Import the Scanner class to allow you to read input from the user.
- The
public static void main(String[] args)
method is the entry point of the program. - Initialize variables:
Scanner scr
is used to read user input.int numElements
will store the number of elements in the array.int[] userScore
is an array to store the user's scores.int i
is a loop counter.
- Use the
nextInt()
method of the Scanner to read an integer from the user. This will determine the size of theuserScore
array. - Use a for loop to read
numElements
integers from the user and store them in theuserScore
array. - Calculate and print the sum of the first and last elements:
- Calculate the sum of the first and last elements by accessing
userScore[0]
anduserScore[numElements - 1]
. - Print the result using
System.out.println()
.
- Calculate the sum of the first and last elements by accessing
Step by step
Solved in 5 steps with 6 images

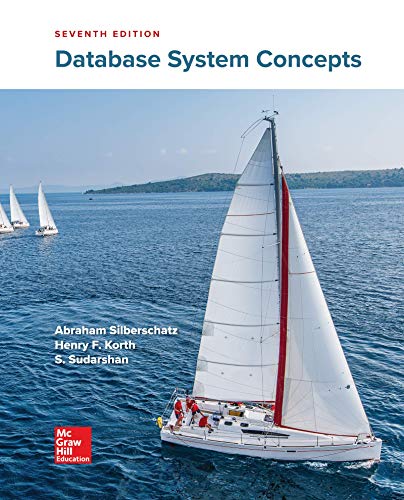
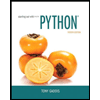
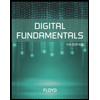
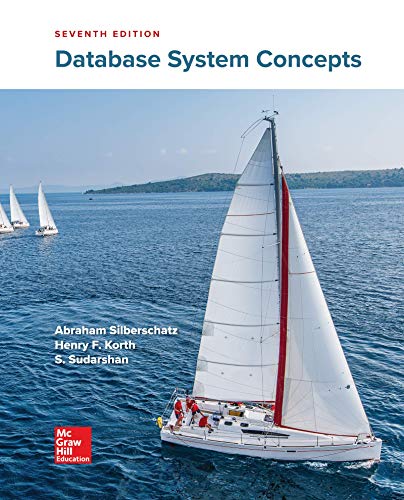
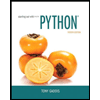
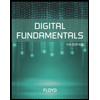
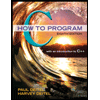
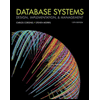
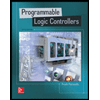