2-3- Trees: Create the 2-3 tree with 11 nodes. Also, implement in-order traversal, and findltem functions. struct Node int small; int large; Node * left; Node* middle; Node* right; void inorder (Node *tree); Node *findItem (Node *tree, int target); 50 90 20 (70 120 150 10 30 40 60 80 100 110) (130 140 (160
2-3- Trees: Create the 2-3 tree with 11 nodes. Also, implement in-order traversal, and findltem functions. struct Node int small; int large; Node * left; Node* middle; Node* right; void inorder (Node *tree); Node *findItem (Node *tree, int target); 50 90 20 (70 120 150 10 30 40 60 80 100 110) (130 140 (160
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help with C++ question in image in C++ language.
Output sample in second image. Thank you.

Transcribed Image Text:## 2-3 Trees:
**Objective:**
Create a 2-3 tree with 11 nodes. Implement in-order traversal and `findItem` functions.
**Data Structure Definition:**
```c
struct Node
{
int small;
int large;
Node* left;
Node* middle;
Node* right;
}
void inOrder(Node *tree);
Node *findItem(Node *tree, int target);
```
**Tree Diagram Explanation:**
- The tree consists of a root node with values 50 and 90.
- The root has three children:
- The first child node contains the value 20, with its own children being 10, 30, and 40.
- The second child node contains the value 70, with its children being 60 and 80.
- The third child node contains values 120 and 150, with its children being 100, 110, 130, 140, and 160.
**Sample Run:**
The diagram illustrates a structured representation of the 2-3 tree, showing hierarchy and connections between different nodes with values ranging from 10 to 160.
- Nodes are distributed in such a way to maintain the properties of a 2-3 tree, where each internal node can have 2 or 3 children and will have either 1 key (in case of a 2-node) or 2 keys (in case of a 3-node).
- Leaf nodes are aligned to show the distribution of lesser, equal, and greater values in adherence to the tree structure requirements.

Transcribed Image Text:**Sample Run Description:**
The output shown in the image displays the results of a program execution that demonstrates two key operations on a data structure: in-order traversal and item search.
**Part 1: In-order Traversal**
- The program performs an in-order traversal of a binary search tree.
- The numbers displayed, "10 20 30 40 50 60 70 80 90 100 110 120 130 140 150 160", represent the nodes visited in sequence. In-order traversal visits nodes in ascending order for a binary search tree.
**Part 2: Find Item**
- The program searches for specific items in the data structure.
1. **Search 50**:
- The program searches for the item "50".
- Output: "Item was found."
- This indicates that the item "50" exists in the data structure.
2. **Search 100**:
- The program searches for the item "100".
- Output: "Item was found."
- This confirms the presence of the item "100" in the data structure.
3. **Search 75**:
- The program searches for the item "75".
- Output: "Item was not found."
- This denotes that the item "75" is absent from the data structure.
The message "Press any key to continue . . ." suggests the program is waiting for user interaction to proceed or terminate.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
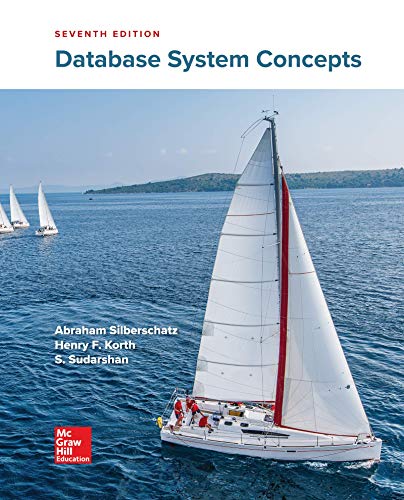
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
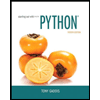
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
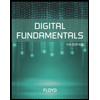
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
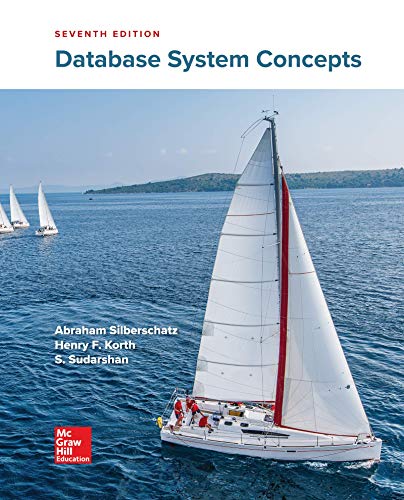
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
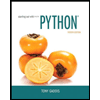
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
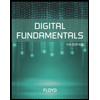
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
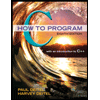
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
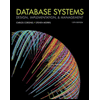
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
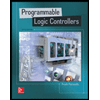
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education