14)Write nested for loops to produce the following output: 11111 2222 333 44 5
14)Write nested for loops to produce the following output: 11111 2222 333 44 5
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
How do you do this? JAVA

Transcribed Image Text:### Nested For Loops to Produce a Specific Output
**Problem Statement:**
Write nested for loops to produce the following output:
```
11111
2222
333
44
5
```
**Detailed Explanation:**
To achieve the given pattern using nested for loops, we need a two-level loop structure:
1. **Outer Loop:** This loop will iterate through each line, controlling the current number being printed. It also determines how many times the number is repeated on the current line.
2. **Inner Loop:** This loop will handle the printing of the current number in each iteration of the outer loop.
**Pseudocode:**
Here is a conceptual representation of the nested loops:
```python
for i in range(1, 6): # Outer loop from 1 to 5
for j in range(6 - i): # Inner loop to control number of prints
print(i, end='') # Print the current number on the same line
print() # Move to the next line after inner loop completes
```
### Explanation:
- **Outer Loop (`for i in range(1, 6)`):**
- It starts from 1 and goes up to 5.
- The variable `i` represents the current number to be printed.
- **Inner Loop (`for j in range(6 - i)`):**
- For each iteration of the outer loop, the inner loop runs from `0` to `5 - i`.
- This ensures that `i` is printed `5 - (i - 1)` times:
- When `i=1`, it prints 5 times (6-1=5)
- When `i=2`, it prints 4 times (6-2=4)
- When `i=3`, it prints 3 times (6-3=3)
- When `i=4`, it prints 2 times (6-4=2)
- When `i=5`, it prints 1 time (6-5=1)
- **Print Statement (`print(i, end='')`):**
- The `end=''` argument ensures that the numbers are printed on the same line without any space.
- **New Line (`print()`):**
- Moves to the next line after the inner loop finishes execution for the current `i`.
By following this combination of
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
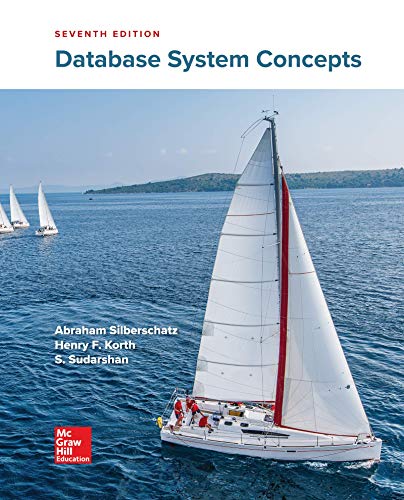
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
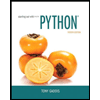
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
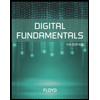
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
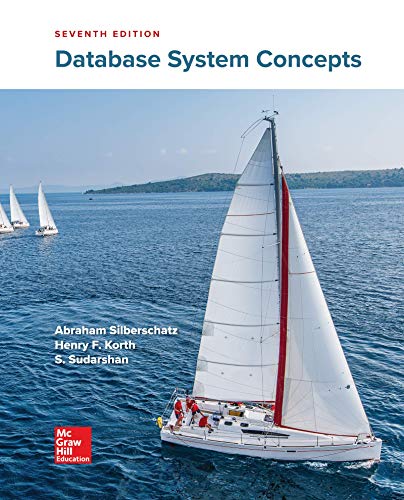
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
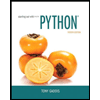
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
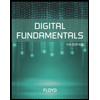
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
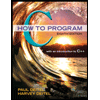
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
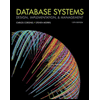
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
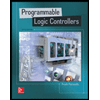
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education