12:34 A cs61a.org Implement add_d_leaves, a function that takes in a Tree instance t and a number v. II
12:34 A cs61a.org Implement add_d_leaves, a function that takes in a Tree instance t and a number v. II
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:**Implementing `add_d_leaves` Function for Trees**
The goal is to implement `add_d_leaves`, a function that takes in a `Tree` instance `t` and a number `v`.
### Understanding Tree Depth
- The *depth* of a node in `t` is defined as the number of edges from the root to that node.
- The root of the tree has a depth of 0.
### Function Objective
For each node in the tree:
- Add `d` leaves to the node, where `d` is its depth.
- Each added leaf should be labeled with `v`.
- If the node at this depth already has branches, append the new leaves to the end of that list.
**Example:**
- At depth 1, add 1 leaf labeled `v`.
- At depth 2, add 2 leaves, and so on.
### Visualization
- A tree `t` is shown with nodes 1 connected to branches 3 and 2, with further nodes extending downward.
- After applying `add_d_leaves` with `v` as 5, the tree extends further as described by the function.
### Diagram Explanation
1. **Initial Tree**
- Root node `1` has two branches: `3` and `2`.
- Branch `3` further extends to `4`.
2. **Modified Tree with `add_d_leaves` (v = 5)**
- Node `1` at depth 0: No additional leaves.
- Node `3` at depth 1: Adds one leaf labeled 5.
- Node `2` at depth 1: Adds one leaf labeled 5.
- Node `4` at depth 2: Adds two leaves labeled 5.
**Hint:** Use a helper function to keep track of the depth!
### Sample Code Structure
```python
def add_d_leaves(t, v):
"""Add d leaves containing v to each node, where d is the depth."""
# Function logic here
```
This approach utilizes a helper function to manage the depth as the tree is traversed, ensuring that the correct number of leaves are added based on the node's depth.
![```python
def add_d_leaves(t, v):
"""Add d leaves containing v to each node in t."""
>>> t_one_to_four = Tree(1, [Tree(2), Tree(3), Tree(4)])
>>> print(t_one_to_four)
1
2
3
4
>>> add_d_leaves(t_one_to_four, 5)
>>> print(t_one_to_four)
1
2
5
3
5
4
5
>>> t1 = Tree(1, [Tree(3)])
>>> add_d_leaves(t1, 4)
>>> t1
Tree(1, [Tree(3, [Tree(4)])])
>>> t2 = Tree(2, [Tree(5), Tree(6)])
>>> t3 = Tree(3, [t1, Tree(0), t2])
>>> print(t3)
3
1
3
4
0
2
5
6
>>> add_d_leaves(t3, 10)
>>> print(t3)
3
1
3
4
10
10
10
0
10
2
5
6
10
10
10
"*** YOUR CODE HERE ***"
```
**Explanation:**
This Python code defines a function `add_d_leaves` that is supposed to add a specified number of leaves containing a given value `v` to each node in a tree `t`.
- **Example 1:**
- Before calling the function, the `t_one_to_four` tree is structured with a root node `1`, and child nodes `2`, `3`, and `4`.
- After calling `add_d_leaves(t_one_to_four, 5)`, a leaf node containing `5` is added to each existing child node.
- **Example 2:**
- A tree `t1` is initialized with a root `1` and a child `3`.
- After `add_d_leaves(t1, 4)`, a leaf](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fda4a7445-4cc9-4690-867b-b0862e018c2b%2Fffc97401-db45-434f-9e94-6b320df33aa9%2Fmlhhd0l_processed.jpeg&w=3840&q=75)
Transcribed Image Text:```python
def add_d_leaves(t, v):
"""Add d leaves containing v to each node in t."""
>>> t_one_to_four = Tree(1, [Tree(2), Tree(3), Tree(4)])
>>> print(t_one_to_four)
1
2
3
4
>>> add_d_leaves(t_one_to_four, 5)
>>> print(t_one_to_four)
1
2
5
3
5
4
5
>>> t1 = Tree(1, [Tree(3)])
>>> add_d_leaves(t1, 4)
>>> t1
Tree(1, [Tree(3, [Tree(4)])])
>>> t2 = Tree(2, [Tree(5), Tree(6)])
>>> t3 = Tree(3, [t1, Tree(0), t2])
>>> print(t3)
3
1
3
4
0
2
5
6
>>> add_d_leaves(t3, 10)
>>> print(t3)
3
1
3
4
10
10
10
0
10
2
5
6
10
10
10
"*** YOUR CODE HERE ***"
```
**Explanation:**
This Python code defines a function `add_d_leaves` that is supposed to add a specified number of leaves containing a given value `v` to each node in a tree `t`.
- **Example 1:**
- Before calling the function, the `t_one_to_four` tree is structured with a root node `1`, and child nodes `2`, `3`, and `4`.
- After calling `add_d_leaves(t_one_to_four, 5)`, a leaf node containing `5` is added to each existing child node.
- **Example 2:**
- A tree `t1` is initialized with a root `1` and a child `3`.
- After `add_d_leaves(t1, 4)`, a leaf
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
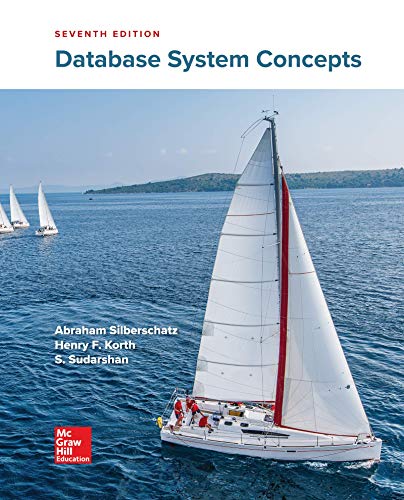
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
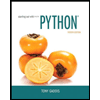
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
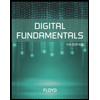
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
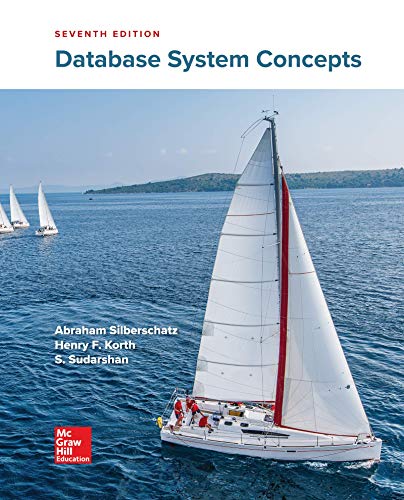
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
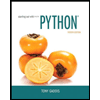
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
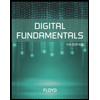
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
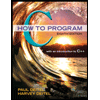
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
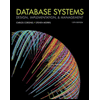
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
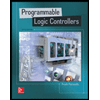
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education