12: guess.cpp) Irite a program that plays a guessing game with the user. The user should pick a letter, and the computer should try to guess the letter. After each guess, the user should tell the computer whether its guess was too high or too low. With this information, the computer should be able to guess the letter within five tries. The user should be able to give whole words or single upper or lower case letters as responses.
Please use C++ and only use libraries <iostream> and <cmath>. Also ultilize cin.ignore to let responses be recognized with a Y or Yes. Code in second pic needs to be included. Thank you!



The variables are declared to hold the starting letter, 'a', ending letter, 'z', and the guessed letter.
char start, end, guess;
start = 'a';
end = 'z';
Other variables are declared to hold user input for the actual letter, if the guessed letter is correct or not, how far is the letter, and the number of attempts of the computer, respectively.
char input;
char result, distance;
int tries;
The c++ code for the given scenario is as follows.
PROGRAM
#include <stdio.h>
#include <iostream>
#include <cmath>
using namespace std;
int main()
{
//variables to hold starting, ending and computer-guessed letter
char start, end, guess;
start = 'a';
end = 'z';
//variable to hold user input
char input;
//variable to hold number of tries
int tries = 0;
std::cout << "Enter a character: ";
cin >> input;
//loop continues till computer fails and attemps all 5 tries
while(tries<5)
{
guess = (start+end)/2;
std::cout << "The letter is " << guess << ". Is the guess correct (y for yes/ n for no)?" << std::endl;
char result;
cin >> result;
if(result == 'n')
{
tries++;
if(tries == 5)
break;
std::cout << "How far is the letter (l for lower than guessed letter/ h for higher)? ";
char distance;
cin >> distance;
if(distance == 'l')
end = guess;
if(distance == 'h')
start = guess;
}
if(result == 'y')
{
std::cout << "Congratulations! The computer wins." << std::endl;
break;
}
}
return 0;
}
Step by step
Solved in 3 steps with 1 images

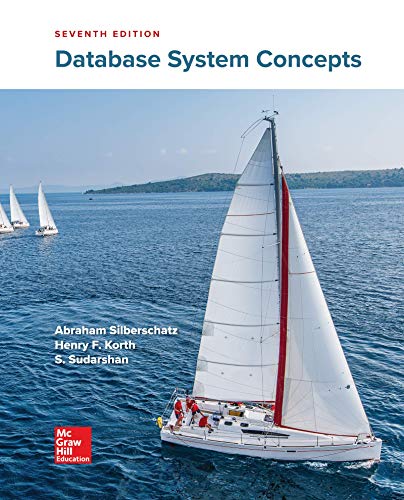
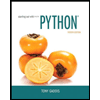
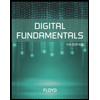
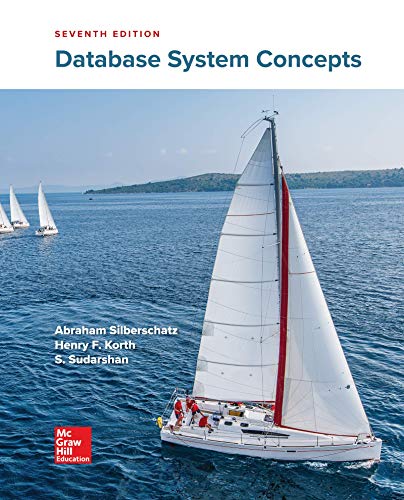
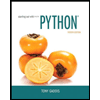
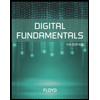
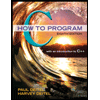
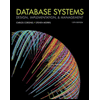
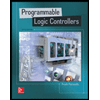