11.9 LAB: Radioactive decay Complete the functions in the template to implement the formulas shown below. The formula to calculate how much of a radioactive isotope with half-life T will remain after time t is: Nt=N0e−0.693tT The formula can be rearranged to calculate the half-life of an isotope given how much remains after decay: T=−0.693tlnNtN0 The notation used in the formulas is: e = Euler's number accessible as math.e. t = the length of time (in years) during which an isotope decays T = the half-life (in years) of the isotope N0 = the initial amount of the isotope Nt the amount of the isotope remaining after time t Then, complete the main program to: Read a choice Read 3 numbers from one line: Choice N: N0, t, and T Choice T: N0, Nt, and t Call compute_Nt() if the choice is N, compute_T if the choice is T Output the result with four digits after the decimal point. code I have so far: import math def compute_Nt(N0, t, T): return N0 * (math.exp(-0.693*t / T)) def compute_T(N0, Nt, t): return -0.693 * t / math.log(Nt/N0) if __name__ == "__main__": choice = input() if choice == 'N' : N0, t, T = map(float, input().split(" ")) Nt = compute_Nt(N0, t, T) print(f'Nt ={Nt : .4f}' ) elif choice == 'T' : N0, Nt, t = map(float, input().split(" ")) T = compute_T(N0, Nt, t) print(f'T ={T: .4f}' ) else: print("Invalid choice.") error I am getting: 1: Unit testkeyboard_arrow_up 0 / 2 Test compute_Nt(100, 50, 28.94). Should return remaining quantity = 30.2007 (rounded to 4 sig. fig.) 2: Unit testkeyboard_arrow_up 0 / 2 Test compute_T(100, 30.2007, 50). Should return half-life = 28.94 (rounded to 2 sig. fig.)
11.9 LAB: Radioactive decay
Complete the functions in the template to implement the formulas shown below.
The formula to calculate how much of a radioactive isotope with half-life T will remain after time t is:
The formula can be rearranged to calculate the half-life of an isotope given how much remains after decay:
The notation used in the formulas is:
- e = Euler's number accessible as math.e.
- t = the length of time (in years) during which an isotope decays
- T = the half-life (in years) of the isotope
- N0 = the initial amount of the isotope
- Nt the amount of the isotope remaining after time t
Then, complete the main program to:
- Read a choice
- Read 3 numbers from one line:
- Choice N: N0, t, and T
- Choice T: N0, Nt, and t
- Call compute_Nt() if the choice is N, compute_T if the choice is T
- Output the result with four digits after the decimal point.
code I have so far:
import math
def compute_Nt(N0, t, T):
return N0 * (math.exp(-0.693*t / T))
def compute_T(N0, Nt, t):
return -0.693 * t / math.log(Nt/N0)
if __name__ == "__main__":
choice = input()
if choice == 'N' :
N0, t, T = map(float, input().split(" "))
Nt = compute_Nt(N0, t, T)
print(f'Nt ={Nt : .4f}' )
elif choice == 'T' :
N0, Nt, t = map(float, input().split(" "))
T = compute_T(N0, Nt, t)
print(f'T ={T: .4f}' )
else:
print("Invalid choice.")
error I am getting:


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

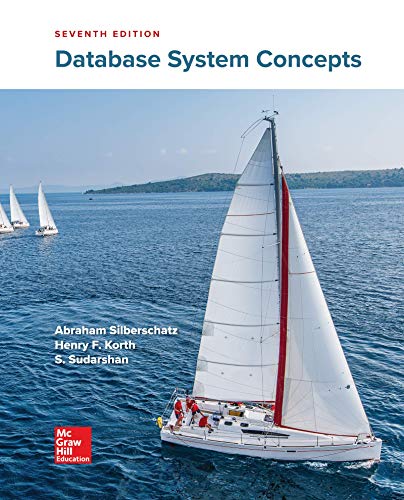
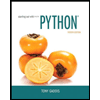
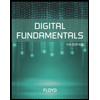
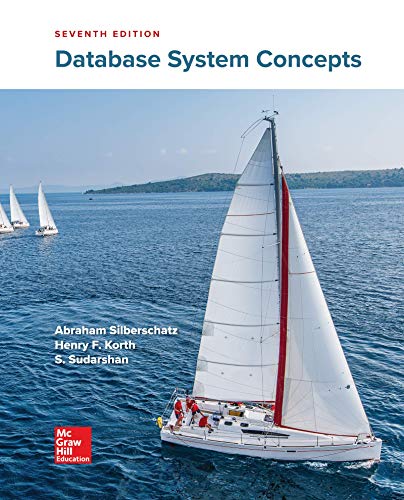
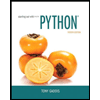
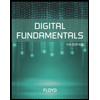
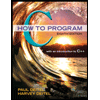
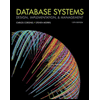
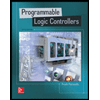