11) What would the following program print? String searchstring = "Tom Jones"; Person current = first; while ( (not(current.equals(searchstring)) && (current.next != null) ) current = current.next; System.out.println(current); Question 11 - What would the following program print? String searchstring = "Tom Jones";Person current = first;while ( (not(current.equals(searchstring)) && (current.next != null) ) current = current.next; System.out.println(current); Blank "Tom Jones" if an only if it is the first element. "Tom Jones" if it is in the list Nothing 12) What is the name of the following search? public static > boolean mySearch (T[] data, int min, int max, T target) { int index = min; boolean found = false; while (!found && index <= max) { if (data[index].compareTo(target) == 0) found = true; index++; } return found; } Question 12 - What is the name of the following search?public static > booleanmySearch (T[] data, int min, int max, T target){ int index = min; boolean found = false; while (!found && index <= max) { if (data[index].compareTo(target) == 0) found = true; index++; }return found;} Exponential Search Binary Search Linear Search Quick Search 13) Asymptotic complexities of some of the algorithms are given below. Please select the slowest one. Question 13 - Asymptotic complexities of some of the algorithms are given below. Please select the slowest one. O(nlogn) O(logn) O(1) O(n) 14) ____ is a Binary Search Tree that ensures the balance is maintained. Question 14 - ____ is a Binary Search Tree that ensures the balance is maintained. Decision Tree Balance Tree Linked list AVL 15) In a Binary Tree with height 5, what is the max and min number of nodes in that Tree? Question 15 - In a Binary Tree with height 5, what is the max and min number of nodes in that Tree? 32 and 6, respectively 31 and 5, respectively 63 and 6, respectively 64 and 5, respectively 16) Two vertices are said to be ________ if there is an edge connecting them. Question 16 - Two vertices are said to be ________ if there is an edge connecting them. conjugate linked adjacent directed 17) Think of a k-ary tree which has k children or no child in every internal node. What is the number of leaves in this tree with n internal nodes? Question 17 - Think of a k-ary tree which has k children or no child in every internal node. What is the number of leaves in this tree with n internal nodes? n(k-1) (n-1)k+1 nk n(k-1)+1 18) Consider a node Y in a Binary Tree. Given that Y has two children, let X be Inorder successor of Y. Which of the following is true about X? Question 18 - Consider a node Y in a Binary Tree. Given that Y has two children, let X be Inorder successor of Y. Which of the following is true about X? X has both children X has no left child X has no right child None of the above 19) What is the complexity of the following code snippet? for (int count = 0; count < n; count++) { printsum (count); } (Select 1) Question 19 - What is the complexity of the following code snippet? for (int count = 0; count < n; count++){ printsum (count);} O(Log N) O(N) O(1) We need to know the complexity of the printsum() function. 20) What does the following function do? public T doSomething() throws EmptyCollectionException{ if (isEmpty()) throw new EmptyCollectionException(“Stack”); T result = top.getElement(); top = top.getNext(); count--; return result; } 21) If you have 3 unlabelled nodes how many binary trees can you create? (3pts) Question 21 - If you have 3 unlabelled nodes how many binary trees can you create? 4 3 1 5 Question 20 - What does the following function do? public T doSomething() throws EmptyCollectionException{ if (isEmpty()) throw new EmptyCollectionException(“Stack”); T result = top.getElement(); top = top.getNext(); count--; return result; } Peek() Pop() Push() isEmpty()
What would the following program print?
String searchstring = "Tom Jones";
Person current = first;
while ( (not(current.equals(searchstring)) && (current.next != null) )
current = current.next;
System.out.println(current);
Blank
|
|
"Tom Jones" if an only if it is the first element.
|
|
"Tom Jones" if it is in the list
|
|
Nothing
|
What is the name of the following search?
public static <T extends Comparable<? super T>> boolean
mySearch (T[] data, int min, int max, T target)
{
int index = min;
boolean found = false;
while (!found && index <= max)
{
if (data[index].compareTo(target) == 0)
found = true;
index++;
}
return found;
}
Exponential Search
|
|
Binary Search
|
|
Linear Search
|
|
Quick Search
|
Asymptotic complexities of some of the
|
|
O(nlogn)
|
|
O(logn)
|
|
O(1)
|
|
O(n)
|
____ is a Binary Search Tree that ensures the balance is maintained.
Decision Tree
|
|
Balance Tree
|
|
Linked list
|
|
AVL
|
32 and 6, respectively
|
|
31 and 5, respectively
|
|
63 and 6, respectively
|
|
64 and 5, respectively
|
Two vertices are said to be ________ if there is an edge connecting them.
conjugate
|
|
linked
|
|
adjacent
|
|
directed
|
Think of a k-ary tree which has k children or no child in every internal node. What is the number of leaves in this tree with n internal nodes?
n(k-1)
|
|
(n-1)k+1
|
|
nk
|
|
n(k-1)+1
|
Consider a node Y in a Binary Tree. Given that Y has two children, let X be Inorder successor of Y. Which of the following is true about X?
X has both children
|
|
X has no left child
|
|
X has no right child
|
|
None of the above
|
What is the complexity of the following code snippet?
for (int count = 0; count < n; count++)
{
printsum (count);
}
O(Log N)
|
|
O(N)
|
|
O(1)
|
|
We need to know the complexity of the printsum() function.
|
What does the following function do?
public T doSomething() throws EmptyCollectionException{
if (isEmpty())
throw new EmptyCollectionException(“Stack”);
T result = top.getElement();
top = top.getNext();
count--;
return result;
}
If you have 3 unlabelled nodes how many binary trees can you create?
4
|
|
3
|
|
1
|
|
5
|
Peek()
|
|
Pop()
|
|
Push()
|
|
isEmpty()
|

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

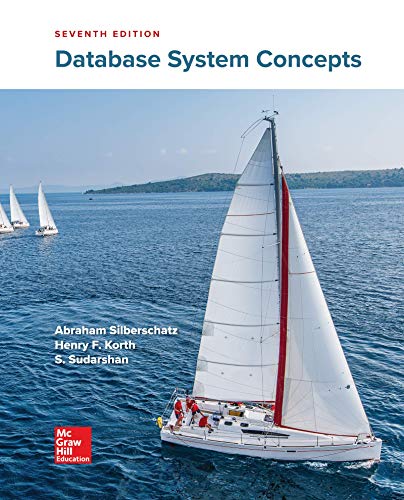
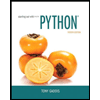
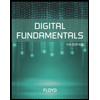
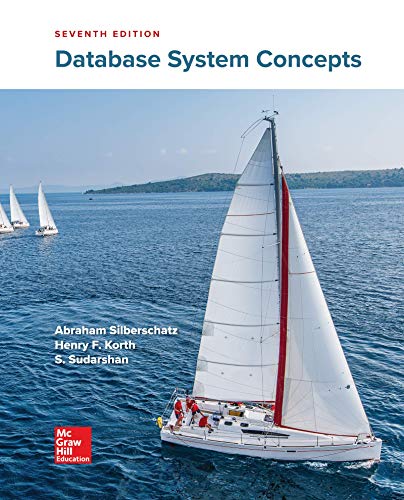
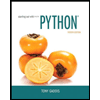
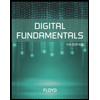
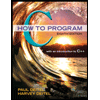
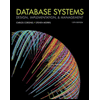
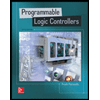