1. Write a program in C/C++ that takes a set of parentheses and braces from the standard input terminal and determines whether the set of parentheses and braces is balanced or not. A set of parentheses and braces is called balanced if for each opening parenthesis or brace these is a corresponding closing parenthesis or brace in correct order In your program a user will enter ‘#’ to indicate the end of the data entry. Your program must use a linked list to store the parentheses and braces entered by the user. Your output format should be as shown in the test runs Your program must contain the following user-defined functions: - makeList – this function creates a linked list from the user input. - printList – this function prints the user inputted data. - push – this function performs push operation of a stack - pop – this function performs pop operation of the stack - stackEmpty – this function returns true is the stack is empty and returns false otherwise - balanceCheck – this function reads data from the linked list that contains user input and utilize the stack to determine whether the user inputted data is balanced or not. Test Run 1: Enter an expression: (())# Input Expression: ( ( ) ) The expression is balanced Test Run 2: Enter an expression: {(}(()))# Input Expression: { ( } ( ( ) ) ) The expression is not balanced Test Run 3: Enter an expression: (# Input Expression: ( The expression is not balanced Test Run 4 Enter an expression: {}()(({}))# Input Expression: { } ( ) ( ( { } ) ) The expression is balanced
1. Write a program in C/C++ that takes a set of parentheses and braces from the standard input terminal and determines whether the set of parentheses and braces is balanced or not. A set of parentheses and braces is called balanced if for each opening parenthesis or brace these is a corresponding closing parenthesis or brace in correct order In your program a user will enter ‘#’ to indicate the end of the data entry.
Your program must use a linked list to store the parentheses and braces entered by the user. Your output format should be as shown in the test runs
Your program must contain the following user-defined functions:
-
- makeList – this function creates a linked list from the user input.
-
- printList – this function prints the user inputted data.
-
- push – this function performs push operation of a stack
-
- pop – this function performs pop operation of the stack
- stackEmpty – this function returns true is the stack is empty and returns false otherwise
-
- balanceCheck – this function reads data from the linked list that contains user input and utilize the stack to determine whether the user inputted data is balanced or not.
Test Run 1:
Enter an expression: (())#
Input Expression: ( ( ) )
The expression is balanced
Test Run 2:
Enter an expression: {(}(()))#
Input Expression: { ( } ( ( ) ) )
The expression is not balanced
Test Run 3:
Enter an expression: (#
Input Expression: (
The expression is not balanced
Test Run 4
Enter an expression: {}()(({}))#
Input Expression: { } ( ) ( ( { } ) )
The expression is balanced

Step by step
Solved in 3 steps with 7 images

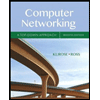
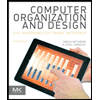
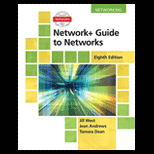
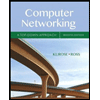
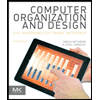
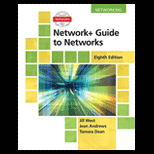
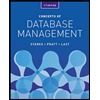
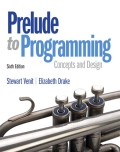
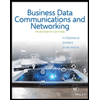