1. Sample Run 1 Amazing Combat Calculator v1 Enter ability name: Slap Enter base damage: 200.25 Enter ability category: N Slap dealt 240.3 boring normal damage to the target Sample Run 2 Amazing Combat Calculator vi Enter ability name: Rest Enter base damage: 100 Enter ability category: H Rest dealt -100.0 damage healing the target. 3. Sample Run 3 Amazing Combat Calculator v1 Enter ability name: FrostFire Enter base damage: 7777.77 Enter ability category: A FrostFire dealt 1944.4 area of effect damage to each targe 4. Sample Run 4 Amazing Combat Calculator v1 Enter ability name: Tomato Toss Enter base damage: 900 Enter ability category: N Ability Tomato Toss not implemented yet. 5. Sample Run 5 Amazing Combat Calculator v1 Enter ability name: Tomato Toss Enter base damage: -1 Enter ability category: H Ability Tomato Toss not implemented yet. 6. Sample Run 6 - Amazing Combat Calculator v1 Enter ability name : Rest Enter base damage: 9001 Enter ability category: H Only Goku can deal more than 9000 dmg. 7. Sample Run 7 Amazing Combat Calculator v1 Enter ability name: Slap Enter base damage: -10 Enter ability category: N All ability damage must be positive.
Hello I'm working on a combat calculator prototype in C++. I'm a bit lost on what to do next.
Program Requirements:
-
There are three valid abilities represented by the following string literals:
- "Rest"
- "Slap"
- "FrostFire"
-
If the ability entered is not one of the valid abilities, the program should output the invalid name entered and indicate the ability has not been implemented yet.
-
Ability names are case sensitive. "rest" should fail while "Rest" would not.
-
The base damage must be a positive numeric value no larger than 9000.
- Floating point values are allowed.
- 0 will be considered negative.
-
If the base damage entered is not positive or is larger than 9000, an error message should be printed out indicating what the problem is.
- A base damage of 0 or lower should output All ability damage must be positive.
- A base damage of over 9000 should output Only Goku can deal more than 9000 dmg.
-
There are three valid ability categories and these categories determine how the damage will be calculated.
- "A" - 'A' is for area of effect abilities. Their damage should be calculated by dividing the base damage by 4.
- "N" - 'N' is for normal ability. The damage should be calculated by multiplying the base damage by the value 1.2
- "H" - 'H' is for healing. So the damage dealt should be converted to a negative number.
-
If an invalid category is entered and all other values are valid, you should output the message Ability category X not implemented. where X is whatever the user entered for the category. If the user had typed "Potato" Then the message should say, Ability category Potato not implemented.
-
Use a switch statement to handle the ability category decision.
-
You may assume the user will input strings when asked and numeric values when asked. So, you do not need to handle program crashes due to someone typing a "word" for damage.
-
You may assume any combination of valid abilities and categories works even though they may not make sense. I.e. the inputs "Slap" "300.24" and "H" would Slap to heal the target even though it makes no sense.
-
Ensure that all numeric output always displays 1 decimal place.
-
When determining output error messages. Ability existence takes priority, then damage being in range, then category. I.e. If all values are invalid only the ability error message appears. If the ability is valid and both damage and category are invalid, only the damage message appears. If only the category is invalid, then we will see the error message for it.



Step by step
Solved in 4 steps with 9 images

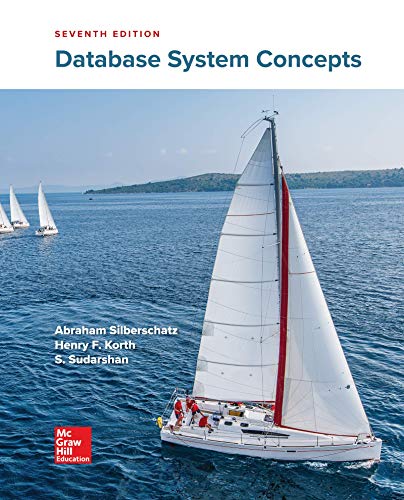
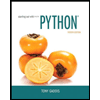
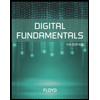
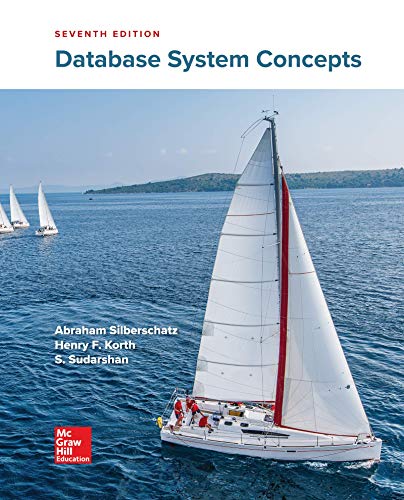
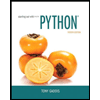
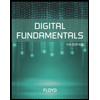
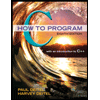
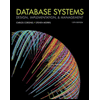
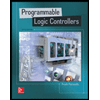