1. (Practice) Enter the data for the info.txt file in Figure 9.1 or download it from this book’s Web site. Then enter and execute Program 9.5 and verify that the backup file was written. 2. (Modify) Modify Program 9.5 to use a getline() function in place of the get() method currently in the program. =============1 - 9.1========== #include #include #include // needed for exit() #include using namespace std; int main() { string fileOne = "info.txt"; // put the filename up front string fileTwo = "info.bak"; char ch; ifstream inFile; ofstream outfile; try //this block tries to open the input file { // open a basic input stream inFile.open(fileOne.c_str()); if (inFile.fail()) throw fileOne; } // end of outer try block catch (string in) // catch for outer try block { cout << "The input file " << in << " was not successfully opened." << endl << " No backup was made." << endl; exit(1); } try // this block tries to open the output file and { // perform all file processing outfile.open(fileTwo.c_str()); if (outfile.fail())throw fileTwo; while ((ch = inFile.get())!= EOF) outfile.put(ch); inFile.close(); outfile.close(); } catch (string out) // catch for inner try block { cout << "The backup file " << out << " was not successfully opened." << endl; exit(1); } cout << "A successful backup of " << fileOne << " named " << fileTwo << " was successfully made." << endl; return 0; }
1. (Practice) Enter the data for the info.txt file in Figure 9.1 or download it from this book’s Web site. Then enter and execute
2. (Modify) Modify Program 9.5 to use a getline() function in place of the get()
method currently in the program.
=============1 - 9.1==========
#include <iostream>
#include <fstream>
#include <cstdlib> // needed for exit()
#include <string>
using namespace std;
int main()
{
string fileOne = "info.txt"; // put the filename up front
string fileTwo = "info.bak";
char ch;
ifstream inFile;
ofstream outfile;
try //this block tries to open the input file
{
// open a basic input stream
inFile.open(fileOne.c_str());
if (inFile.fail()) throw fileOne;
} // end of outer try block
catch (string in) // catch for outer try block
{
cout << "The input file " << in
<< " was not successfully opened." << endl
<< " No backup was made." << endl;
exit(1);
}
try // this block tries to open the output file and
{ // perform all file processing
outfile.open(fileTwo.c_str());
if (outfile.fail())throw fileTwo;
while ((ch = inFile.get())!= EOF)
outfile.put(ch);
inFile.close();
outfile.close();
}
catch (string out) // catch for inner try block
{
cout << "The backup file " << out
<< " was not successfully opened." << endl;
exit(1);
}
cout << "A successful backup of " << fileOne
<< " named " << fileTwo << " was successfully made." << endl;
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 4 images

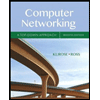
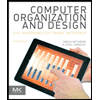
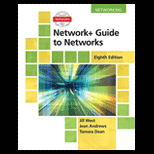
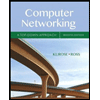
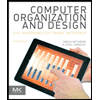
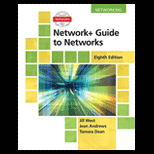
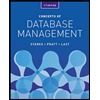
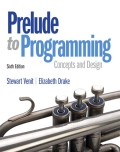
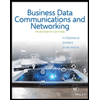