1. Create an application named StudentsStanding.java that allows you to enter student data that consists of an ID number, first name, last name, and grade point average. Have the program accept input until ZZZ is entered for the ID number. Depending on whether the student's grade point average is at least 2.0, output each record either to a file of students in good standing or those on academic probation.
1. Create an application named StudentsStanding.java that allows you to enter student data that consists of an ID number, first name, last name, and grade point average. Have the program accept input until ZZZ is entered for the ID number. Depending on whether the student's grade point average is at least 2.0, output each record either to a file of students in good standing or those on academic probation.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Since this is a 2 part question, I will ask them separately per Bartleby policy. Can you go ahead and do Part 1 please. Thanks

Transcribed Image Text:**1. Create an application named StudentsStanding.java**
- The application allows you to enter student data consisting of:
- ID number
- First name
- Last name
- Grade point average (GPA)
- The program accepts input until 'ZZZ' is entered for the ID number.
- Based on the student's GPA:
- If GPA is **at least 2.0**, classify the student as in "good standing".
- Otherwise, classify them as on "academic probation".
**2. Create an application named StudentsStanding2.java**
- This application displays records from the files created by the StudentsStanding application.
- For each record, display:
- ID number
- First name
- Last name
- GPA
- The amount by which the GPA exceeds or falls short of the 2.0 cutoff.
- Example output formatting:
**Probationary Standing**
- **ID #10** Mike Green GPA: 1.9 \(-0.1\) from 2.0 cutoff
**Good Standing**
- **ID #100** Jill Green GPA: 2.0 \(0.0\) from 2.0 cutoff
- **ID #50** Jane Doe GPA: 3.7 \(+1.7\) from 2.0 cutoff
![### Java Code: File Path Initialization
The image displays a Java code snippet from a file named `StudentsStanding.java`. This code is part of a program that likely manages student academic data, categorizing students based on their academic standing.
#### Code Explanation
1. **Imports:**
- `java.nio.file.*`: Provides classes for file and directory operations.
- `java.io.*`: Includes classes for input and output functionality.
- `java.nio.file.StandardOpenOption.*`: Contains options for opening files.
- `java.util.Scanner`: Allows parsing of primitive types and strings using regular expressions.
- `java.nio.channels.FileChannel`: Supports reading, writing, mapping, and manipulating file content.
2. **Class Definition:**
- **Class Name:** `StudentsStanding`
- The class contains a `main` method which is the entry point of any Java application.
3. **Main Method:**
- `public static void main(String[] args)`: The main method is defined as public and static, allowing JVM to invoke it without object instantiation.
- **Variables:**
- **`goodFile`:** A `Path` object assigned to the path `"/root/sandbox/StudentsGoodStanding.txt"`.
- **`probFile`:** A `Path` object assigned to the path `"/root/sandbox/StudentsAcademicProbation.txt"`.
4. **Comments Section:**
- A placeholder comment `// Write your code here` is included, indicating where additional logic should be implemented.
This code sets up file paths for handling data related to students in good standing and those on academic probation. The next steps would likely involve writing code to read from these files, process the data, and perhaps save changes or outputs to new files.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F7d848391-a5b1-45af-b291-d5739fd6d88e%2F0d7c37e9-614f-4ae4-abd4-84a101ad80ce%2F0dhxvei_processed.png&w=3840&q=75)
Transcribed Image Text:### Java Code: File Path Initialization
The image displays a Java code snippet from a file named `StudentsStanding.java`. This code is part of a program that likely manages student academic data, categorizing students based on their academic standing.
#### Code Explanation
1. **Imports:**
- `java.nio.file.*`: Provides classes for file and directory operations.
- `java.io.*`: Includes classes for input and output functionality.
- `java.nio.file.StandardOpenOption.*`: Contains options for opening files.
- `java.util.Scanner`: Allows parsing of primitive types and strings using regular expressions.
- `java.nio.channels.FileChannel`: Supports reading, writing, mapping, and manipulating file content.
2. **Class Definition:**
- **Class Name:** `StudentsStanding`
- The class contains a `main` method which is the entry point of any Java application.
3. **Main Method:**
- `public static void main(String[] args)`: The main method is defined as public and static, allowing JVM to invoke it without object instantiation.
- **Variables:**
- **`goodFile`:** A `Path` object assigned to the path `"/root/sandbox/StudentsGoodStanding.txt"`.
- **`probFile`:** A `Path` object assigned to the path `"/root/sandbox/StudentsAcademicProbation.txt"`.
4. **Comments Section:**
- A placeholder comment `// Write your code here` is included, indicating where additional logic should be implemented.
This code sets up file paths for handling data related to students in good standing and those on academic probation. The next steps would likely involve writing code to read from these files, process the data, and perhaps save changes or outputs to new files.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
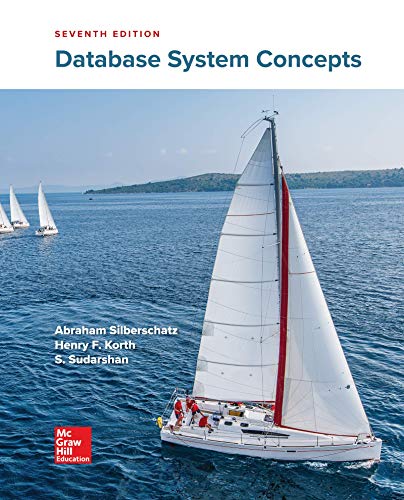
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
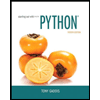
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
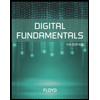
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
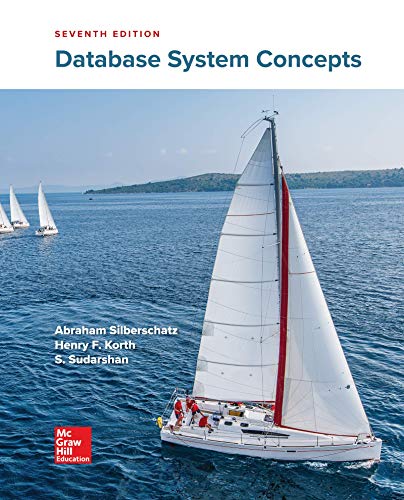
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
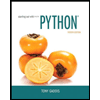
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
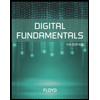
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
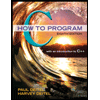
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
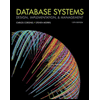
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
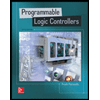
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education