1. Complete classes OneCard and CardThread. Modify them as needed. You can add more variables & methods, but do not change the visibility of existing ones
1. Complete classes OneCard and CardThread. Modify them as needed. You can add more variables & methods, but do not change the visibility of existing ones
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%
JAVA:

Transcribed Image Text:1. Complete classes OneCard and CardThread. Modify them as needed. You can add more
variables & methods, but do not change the visibility of existing ones
class OneCard {
private int
private int
score;
suit;
private int
rank;
private boolean drawn;
public int getScore()
public int getSuit()
public int getRank()
public boolean isDrawn()
} // end OneCard
// 0-51
// clubs, diamonds, hearts, spades
// ace, 2-10, jack, queen, king
// whether this card is drawn
{ return score; }
{ return suit; }
{ return rank; }
{ return drawn; }
class Card Thread extends Thread {
private PrintWriter out;
private ArrayList<OneCard> allCards;
private int targetScore;
public CardThread (String n, int t)
public void run() {
// 1. Put all 52 cards in the ArrayList
}
} // end CardThread
// each thread writes to a separate file
// a deck of all cards
// score of target card
// 2. Keep drawing a card until finding the target
// 3. Every time a card is drawn, print round number & that card to output file
// 4. After finding the target, report #rounds to screen
// 5. Each card can be drawn only once, so max #rounds
= 52
Note: To use java.util. Random object
Random random = new Random();
random.nextInt (10);
{ super(n); targetScore = t; }
// an integer in [0, 10) range
2. Write another class that acts as the main class. In its main method
2.1 Ask user for #threads
2.2 Random a target card
2.3 Create CardThreads to perform the tasks in (1)

Transcribed Image Text:exec-maven-plugin:3.0.0:exec (default-cli)
Number of threads =
3
Target card = 4 of Diamonds
Thread 1 finishes in 35 rounds
Thread 2 finishes in 52 rounds
Thread_0 finishes in 49 rounds
---
BUILD SUCCESS
Thread_0.txt
Round 1 random card = Q of Spades
Round 2 random card = 5 of Hearts
Round 3 random card = 2 of Clubs
Round 48: random card =
Round 49 random card =
Thread_2.txt
Round 1 random card =
Round 2 random card =
Round 3 random card =
Round 51 random card =
Round 52: random card =
A of Hearts
4 of Diamonds
3 of Spades
K of Spades
7 of Hearts
A of Clubs
4 of Diamonds
In different runs, the finishing order
between threads should be different. If it
is always Thread_0, Thread_1, Thread_2, ...,
then you may not do multithreaded program
properly
Threads also have to compete for System.out.
If #rounds are close, the one who finishes
first may get System.out later
Thread_1.txt
1 random card=
random card =
random card =
Round
Round 2
Round 3
Round 34
Round 35
random card =
random card =
J of Hearts
4 of Hearts
7 of Diamonds
8 of Spades
4 of Diamonds
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 4 images

Recommended textbooks for you
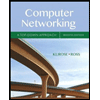
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
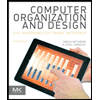
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
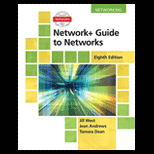
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
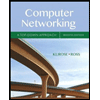
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
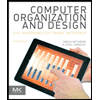
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
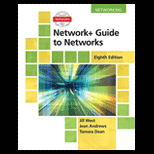
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
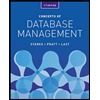
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
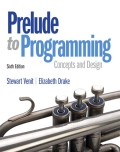
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
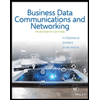
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY