1 write c++ program to calculate the area of 5 rectangles using for loop, 2 then using while loop 4 define length, width, area 6 input length, width
1 write c++ program to calculate the area of 5 rectangles using for loop, 2 then using while loop 4 define length, width, area 6 input length, width
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:## Programming Task: Calculating Rectangle Areas Using Loops
### Objective
To create a C++ program that calculates the area of 5 rectangles. First, using a `for` loop, and then using a `while` loop.
### Instructions
Follow the steps below to accomplish this task.
### Steps
1. **Write C++ Program to Calculate Areas of Rectangles**
- **For Loop:**
- Initialize variables for length, width, and area.
- Use a `for` loop to iterate through the process 5 times.
- In each iteration, input the length and width of the rectangle.
- Calculate the area.
- Output the area.
- **While Loop:**
- Initialize variables for length, width, area, and a counter.
- Use a `while` loop to iterate until the counter reaches 5.
- In each iteration, input the length and width of the rectangle.
- Calculate the area.
- Output the area.
- Increment the counter.
### Code Outline
```cpp
// C++ Program to Calculate the Area of 5 Rectangles using For Loop
#include <iostream>
using namespace std;
int main() {
int length, width, area;
for (int i = 0; i < 5; ++i) {
cout << "Enter the length and width of rectangle " << i+1 << ": ";
cin >> length >> width;
area = length * width;
cout << "Area of rectangle " << i+1 << ": " << area << endl;
}
return 0;
}
// C++ Program to Calculate the Area of 5 Rectangles using While Loop
#include <iostream>
using namespace std;
int main() {
int length, width, area;
int counter = 0;
while (counter < 5) {
cout << "Enter the length and width of rectangle " << counter+1 << ": ";
cin >> length >> width;
area = length * width;
cout << "Area of rectangle " << counter+1 << ": " << area << endl;
++counter;
}
return 0;
}
```
### Explanation
#### Code on the Educational Website:
```
// Instruction text on the website
write c++ program to calculate the area of 5 rectangles using for loop, then using while loop
define
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Recommended textbooks for you
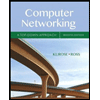
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
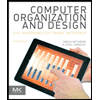
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
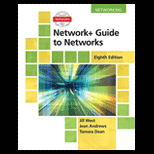
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
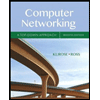
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
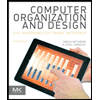
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
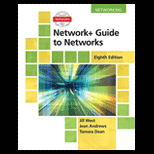
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
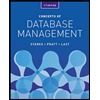
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
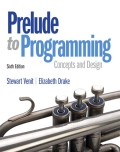
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
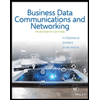
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY