1 // Start with a penny 2 |/ double it every day 3 // how much do you have in a 30-day month? 4 public class DebugSix1 5 { public static void main(String args[]) 8. final int DAYS = 30; 9. double money 0.01; 10 double moneyAmt = 2 * money; 11 for(int x = 2; x <= DAYS; x++){ 12 System.out.println("After day " + x + " you have + moneyAmt); 13 } 14 15 }
1 // Start with a penny 2 |/ double it every day 3 // how much do you have in a 30-day month? 4 public class DebugSix1 5 { public static void main(String args[]) 8. final int DAYS = 30; 9. double money 0.01; 10 double moneyAmt = 2 * money; 11 for(int x = 2; x <= DAYS; x++){ 12 System.out.println("After day " + x + " you have + moneyAmt); 13 } 14 15 }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Start with a penny, double it every day. How much do you have in a 30-day month?
***How do I fix my code? I want the output to look the expected output in red.***
![### Java Code: Doubling a Penny
This code aims to simulate the process of starting with a penny and doubling its value every day for a 30-day month. The logic is implemented in a simple Java program.
#### Code Explanation
```java
// Start with a penny
// double it every day
// how much do you have in a 30-day month?
public class DebugSix1
{
public static void main(String args[])
{
final int DAYS = 30;
double money = 0.01;
double moneyAmt = 2 * money;
for(int x = 2; x <= DAYS; x++)
{
System.out.println("After day " + x + " you have " + moneyAmt);
}
}
}
```
1. **Start with a Penny**: The variable `money` is initialized to 0.01, representing the initial amount.
2. **Calculating Amount**: The variable `moneyAmt` is meant to double the initial amount (`money`) every day.
3. **Loop through Each Day**:
- A `for` loop starts from day 2 up to 30.
- It prints the message "After day x you have y" indicating the amount after each day.
- There seems to be a logical error since `moneyAmt` is not updated within the loop, leading to incorrect output.
#### Output Analysis
- The output column in the terminal shows the series of printed statements, but all values remain at 0.02, indicating no actual doubling is occurring. This is due to `moneyAmt` not being recalculated for each iteration.
#### Corrective Action
To implement the intended doubling, `moneyAmt` should be updated inside the loop:
```java
for(int x = 2; x <= DAYS; x++)
{
moneyAmt *= 2; // Correctly doubles the amount
System.out.println("After day " + x + " you have " + moneyAmt);
}
```
### Key Takeaways
- Ensure variables intended to update are manipulated inside the loop.
- Properly test and debug code to achieve expected results.
- Understanding the logic flow in loops is essential for programming tasks such as financial computations.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffc7dcf27-7da0-4176-9a7f-7dcd8fce94e7%2Fe653eba3-8f32-4d37-9ef8-7aa182713473%2Fr9gxqko_processed.png&w=3840&q=75)
Transcribed Image Text:### Java Code: Doubling a Penny
This code aims to simulate the process of starting with a penny and doubling its value every day for a 30-day month. The logic is implemented in a simple Java program.
#### Code Explanation
```java
// Start with a penny
// double it every day
// how much do you have in a 30-day month?
public class DebugSix1
{
public static void main(String args[])
{
final int DAYS = 30;
double money = 0.01;
double moneyAmt = 2 * money;
for(int x = 2; x <= DAYS; x++)
{
System.out.println("After day " + x + " you have " + moneyAmt);
}
}
}
```
1. **Start with a Penny**: The variable `money` is initialized to 0.01, representing the initial amount.
2. **Calculating Amount**: The variable `moneyAmt` is meant to double the initial amount (`money`) every day.
3. **Loop through Each Day**:
- A `for` loop starts from day 2 up to 30.
- It prints the message "After day x you have y" indicating the amount after each day.
- There seems to be a logical error since `moneyAmt` is not updated within the loop, leading to incorrect output.
#### Output Analysis
- The output column in the terminal shows the series of printed statements, but all values remain at 0.02, indicating no actual doubling is occurring. This is due to `moneyAmt` not being recalculated for each iteration.
#### Corrective Action
To implement the intended doubling, `moneyAmt` should be updated inside the loop:
```java
for(int x = 2; x <= DAYS; x++)
{
moneyAmt *= 2; // Correctly doubles the amount
System.out.println("After day " + x + " you have " + moneyAmt);
}
```
### Key Takeaways
- Ensure variables intended to update are manipulated inside the loop.
- Properly test and debug code to achieve expected results.
- Understanding the logic flow in loops is essential for programming tasks such as financial computations.

Transcribed Image Text:**Results Explanation:**
The results display a pattern of exponential growth over a period of 30 days. The value approximately doubles each day, illustrating how quickly exponential growth can increase a quantity over time. This is a common mathematical concept used in various fields such as finance, biology, and computer science.
Here is the detailed transcription of the daily growth:
- After day 2 you have 0.02
- After day 3 you have 0.04
- After day 4 you have 0.08
- After day 5 you have 0.16
- After day 6 you have 0.32
- After day 7 you have 0.64
- After day 8 you have 1.28
- After day 9 you have 2.56
- After day 10 you have 5.12
- After day 11 you have 10.24
- After day 12 you have 20.48
- After day 13 you have 40.96
- After day 14 you have 81.92
- After day 15 you have 163.84
- After day 16 you have 327.68
- After day 17 you have 655.36
- After day 18 you have 1310.72
- After day 19 you have 2621.44
- After day 20 you have 5242.88
- After day 21 you have 10485.76
- After day 22 you have 20971.52
- After day 23 you have 41943.04
- After day 24 you have 83886.08
- After day 25 you have 167772.16
- After day 26 you have 335544.32
- After day 27 you have 671088.64
- After day 28 you have 1342177.28
- After day 29 you have 2684354.56
- After day 30 you have 5368709.12
These numbers illustrate the rapid increase due to exponential growth. Initially, increases are small, but eventually, they become extremely large. This example is often used to demonstrate concepts like compound interest and population growth.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
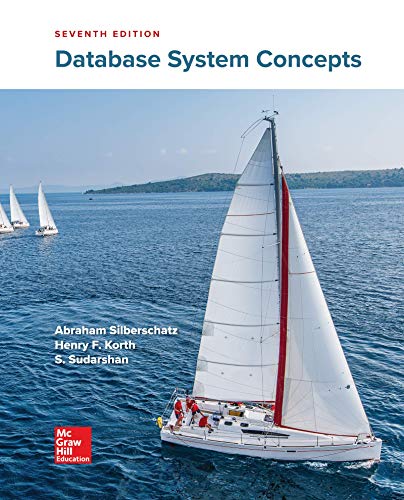
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
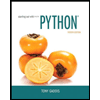
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
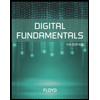
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
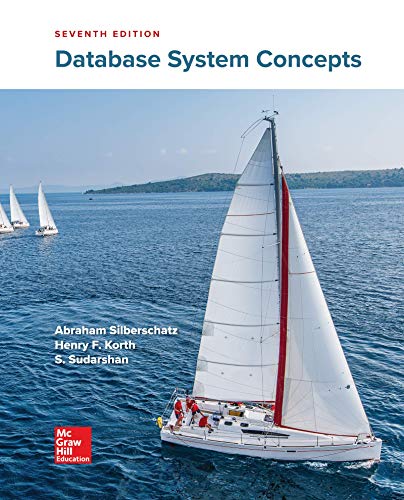
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
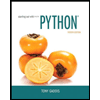
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
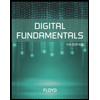
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
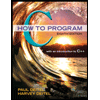
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
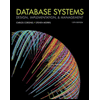
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
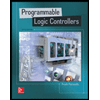
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education