++. Please do not change the existing code. The instructions are in the image that is provided. Please zoom in or you can download the png file. Can you please provide every section of code that is needed there is Time.cpp, Time.h, Date.cpp, Date.h, Event.cpp, Event.h, and main.cpp. Thank you!
C++. Please do not change the existing code. The instructions are in the image that is provided. Please zoom in or you can download the png file. Can you please provide every section of code that is needed there is Time.cpp, Time.h, Date.cpp, Date.h, Event.cpp, Event.h, and main.cpp. Thank you!
Time.cpp
#include "Time.h"
//Default Constructor
//Constructor with parameters
int Time::getHour() const { return hour; }
int Time::getMinute() const { return minute; }
int Time::getSecond() const { return second; }
void Time::setHour(int h) { hour = h; }
void Time::setMinute(int m) { minute = m; }
void Time::setSecond(int s) { second = s; }
int Time::timeToSeconds() const
{
return (getSecond() + getMinute() * 60 + getHour() * 3600);
}
const Time Time::secondsToTime(int s) const
{
int resultS = s % 60;
s /= 60;
int resultM = s % 60;
s /= 60;
int resultH = s % 24;
return Time(resultH, resultM, resultS);
}
//toString
// +
// -
// <
// >
// ==
------
Time.h
#ifndef TIME
#define TIME
#include <string>
using namespace std;
class Time
{
private:
int hour;
int minute;
int second;
int timeToSeconds() const;
const Time secondsToTime(int s) const;
public:
Time();
Time(int h, int m, int s);
string toString() const;
int getHour() const;
void setHour(int h);
int getMinute() const;
void setMinute(int m);
int getSecond() const;
void setSecond(int s);
const Time operator+(const Time &other) const;
const Time operator-(const Time &other) const;
bool operator<(const Time &other) const;
bool operator>(const Time &other) const;
bool operator==(const Time &other) const;
};
#endif
------
Date.cpp
#include "Date.h"
//Default Constructor
//Constructor with parameters
int Date::getDay() const { return day; }
int Date::getMonth() const { return month; }
int Date::getYear() const { return year; }
void Date::setDay(int h) { day = h; }
void Date::setMonth(int m) { month = m; }
void Date::setYear(int s) { year = s; }
//dateToDays
//daysToDate
//toString
// +
// -
// <
// >
// ==
------
Date.h
#ifndef DATE
#define DATE
#include <string>
using namespace std;
class Date
{
private:
int day;
int month;
int year;
int dateToDays() const;
const Date daysToDate(int ndays) const;
public:
Date();
Date(int d, int m, int y);
string toString() const;
int getDay() const;
void setDay(int d);
int getMonth() const;
void setMonth(int m);
int getYear() const;
void setYear(int y);
const Date operator+(int ndays) const;
const Date operator-(int ndays) const;
bool operator<(const Date &other) const;
bool operator>(const Date &other) const;
bool operator==(const Date &other) const;
};
#endif
------
Event.cpp
------
Event.h
------
main.cpp
#include <iostream>
#include "Time.h"
#include "Date.h"
#include "Event.h"
using namespace std;
int main() {
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

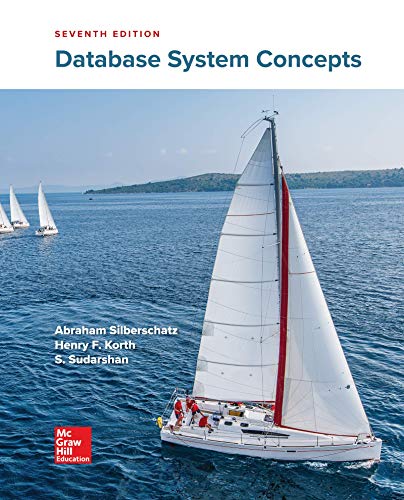
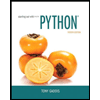
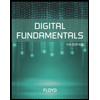
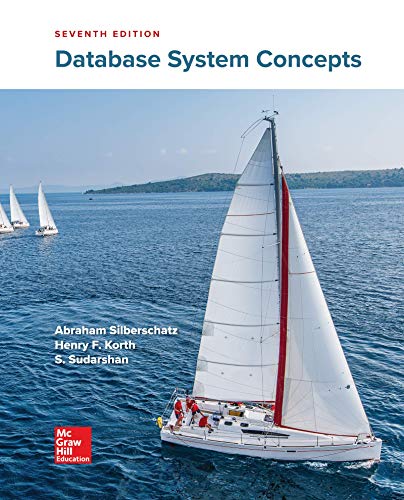
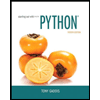
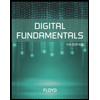
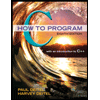
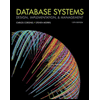
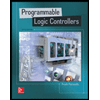