Lab 8
pdf
keyboard_arrow_up
School
Western University *
*We aren’t endorsed by this school
Course
1000A
Subject
Statistics
Date
Jan 9, 2024
Type
Pages
4
Uploaded by SargentStingrayPerson1024
11/2/22, 5:35 PM
Lab 8
localhost:8888/nbconvert/html/Documents/Python_examples/Lab_8/Lab 8.ipynb?download=false
1/4
Lab 8
In this lab we discuss simple random sampling, systematic sampling, and stratified random sampling.
Simple Random Sampling
random.sample:
https://www.w3schools.com/python/ref_random_sample.asp
(https://www.w3schools.com/python/ref_random_sample.asp)
In [1]:
import
numpy
as
np
import
pandas
as
pd
import
random
In [2]:
# Random sampling using random.sample()
# random.sample returns unique random elements from a sequence or set. This is sampling without replacement.
random
.
seed(
52
)
## setting the seed so that we all get the same sample
names
=
[
"Roger"
,
"Jack"
,
"John"
,
"Jason"
,
"Laura"
,
"Mariya"
,
"Martina"
,
"Lauren"
]
sampled_list1
=
random
.
sample(names,
3
)
print
(sampled_list1)
In [3]:
# if we change the seed another sample is obtained
random
.
seed(
17
)
sampled_list2
=
random
.
sample(names,
3
)
print
(sampled_list2)
In [4]:
# an alternative way to sample is to assign a number to each name (or ID) in the list and then sample the numbers
# generating 8 consecutive numbers corresponding to the positions of each name in the list:
sequence_numbers
=
list
(
range
(
8
))
print
(sequence_numbers)
# Python starts with zero
random
.
seed(
17
)
# same seed as before to obtain the same sample of names as in the code cell above
sample_list3
=
random
.
sample(sequence_numbers,
3
)
# randomly sampling 3 number positions
print
(sample_list3)
In [5]:
[names[i]
for
i
in
sample_list3 ]
# getting the names corresponding to the sampled number positions
In [6]:
# Getting a sample array from a multidimensional array
array
=
np
.
array([[
2
,
5
,
7
], [
5
,
11
,
16
], [
6
,
13
,
19
], [
7
,
15
,
22
], [
8
,
17
,
25
]])
print
(
"2D array
\n
"
, array)
In [7]:
random
.
seed(
48
)
random_rows
=
random
.
sample(
range
(
5
),
2
)
# randomly selecting two row indices, without replacement
print
(random_rows)
In [8]:
array[random_rows, :]
Systematic Sampling
Systematic sampling is a type of sampling where we obtain a sample by going through a list of the population at fixed intervals from a randomly chosen starting point.
['Laura', 'Roger', 'Mariya']
['Martina', 'Lauren', 'John']
[0, 1, 2, 3, 4, 5, 6, 7]
[6, 7, 2]
Out[5]:
['Martina', 'Lauren', 'John']
2D array
[[ 2
5
7]
[ 5 11 16]
[ 6 13 19]
[ 7 15 22]
[ 8 17 25]]
[4, 2]
Out[8]:
array([[ 8, 17, 25],
[ 6, 13, 19]])
11/2/22, 5:35 PM
Lab 8
localhost:8888/nbconvert/html/Documents/Python_examples/Lab_8/Lab 8.ipynb?download=false
2/4
np.arange:
https://numpy.org/doc/stable/reference/generated/numpy.arange.html
(https://numpy.org/doc/stable/reference/generated/numpy.arange.html)
In [9]:
# Let's assume we are interested in sampling from a population of 15 students with the following ID list:
df_students
=
pd
.
DataFrame({
'ID'
:np
.
arange(
1
,
16
)
.
tolist()})
df_students
In [10]:
# Defining the function for systematic sampling
def
systematic_sampling
(df, starting_index, step):
indices
=
np
.
arange(starting_index,
len
(df), step
=
step)
systematic_sample
=
df
.
iloc[indices]
return
systematic_sample
In [11]:
# Obtaining a systematic sample of size 5
# Because 15/3=5, choose one of the first 3 IDs on the list at random and then every 3rd ID after that.
random
.
seed(
68
)
random_start
=
random
.
randint(
0
,
2
)
print
(random_start)
# another way
# random.seed(68)
# random_start = random.sample(range(3),1)
# print(random_start)
In [12]:
systematic_sample
=
systematic_sampling(df
=
df_students, starting_index
=
random_start, step
=3
)
systematic_sample
# recall that Python starts at position 0, so position 2 corresponds to ID = 3
Stratified Random Sampling
Another type of sampling is stratified random sampling, in which a population is split into groups and a certain number of members from each group are randomly
selected to be included in the sample.
Stratified Random Sampling Using Counts
Out[9]:
ID
0
1
1
2
2
3
3
4
4
5
5
6
6
7
7
8
8
9
9
10
10
11
11
12
12
13
13
14
14
15
2
Out[12]:
ID
2
3
5
6
8
9
11
12
14
15
11/2/22, 5:35 PM
Lab 8
localhost:8888/nbconvert/html/Documents/Python_examples/Lab_8/Lab 8.ipynb?download=false
3/4
In [13]:
# Suppose we have the following dataframe containing the ID of 8 students from 2 different undergrad programs.
# This is our population list.
df
=
pd
.
DataFrame({
'ID'
:np
.
arange(
1
,
9
)
.
tolist(),
'program'
:[
'Stats'
]
*4 +
[
'Math'
]
*4
})
# 4 students in Stats, 4 students in Math
df
In [14]:
# random sample of 2 Stats students
df_Stats
=
df[df[
'program'
]
==
'Stats'
]
df_Stats
random_rows
=
random
.
sample(
range
(
4
),
2
)
#randomly selecting 2 students from the 4 Stats students in the populatio
n
print
(df_Stats
.
iloc[random_rows])
In [15]:
# random sample of 2 Math students
df_Math
=
df[df[
'program'
]
==
'Math'
]
df_Math
random_rows
=
random
.
sample(
range
(
4
),
2
)
#randomly selecting 2 students from the 4 Math students in the population
print
(df_Math
.
iloc[random_rows])
In [16]:
# Alternative way using one line of code and additional Python functions
DataFrame.groupby:
https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.groupby.html
(https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.groupby.html)
DataFrame.apply:
https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.apply.html
(https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.apply.html)
DataFrame.sample:
https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.sample.html
(https://pandas.pydata.org/docs/reference/api/pandas.DataFrame.sample.html)
In [17]:
# Stratified random sampling by randomly selecting 2 students from each program to be included in the sample
df
.
groupby(
'program'
, group_keys
=
False
)
.
apply(
lambda
x:x
.
sample(
2
))
Stratified Random Sampling Using Proportions
Out[13]:
ID
program
0
1
Stats
1
2
Stats
2
3
Stats
3
4
Stats
4
5
Math
5
6
Math
6
7
Math
7
8
Math
ID program
3
4
Stats
2
3
Stats
ID program
4
5
Math
6
7
Math
Out[17]:
ID
program
4
5
Math
6
7
Math
0
1
Stats
1
2
Stats
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
11/2/22, 5:35 PM
Lab 8
localhost:8888/nbconvert/html/Documents/Python_examples/Lab_8/Lab 8.ipynb?download=false
4/4
In [18]:
# Now suppose we have the following population dataframe with 25% Stats and 75% Math students.
df
=
pd
.
DataFrame({
'ID'
:np
.
arange(
1
,
9
)
.
tolist(),
'program'
:[
'Stats'
]
*2 +
[
'Math'
]
*6
})
# 2 students in Stats, 6 students in Math
df
np.rint:
https://numpy.org/doc/stable/reference/generated/numpy.rint.html
(https://numpy.org/doc/stable/reference/generated/numpy.rint.html)
In [19]:
# Stratified random sampling such that the proportion of students in each program sample
# matches the proportion of students from each program in the population dataframe
N
= 4
# sample size
# So, the sample must contain 1 random student from Stats and 3 from Math to maintain the population proportions
In [20]:
# random sample of Stats students
df_Stats
=
df[df[
'program'
]
==
'Stats'
]
df_Stats
random_rows
=
random
.
sample(
range
(
2
),
1
)
#sampling 1 student from the 2 Stats students in the population
print
(df_Stats
.
iloc[random_rows])
In [21]:
# random sample of Math students
df_Math
=
df[df[
'program'
]
==
'Math'
]
df_Math
random_rows
=
random
.
sample(
range
(
6
),
3
)
#sampling 3 students from the 6 Math students in the population
print
(df_Math
.
iloc[random_rows])
Alternative way:
np.rint:
https://numpy.org/doc/stable/reference/generated/numpy.rint.html
(https://numpy.org/doc/stable/reference/generated/numpy.rint.html)
In [22]:
df
.
groupby(
'program'
, group_keys
=
False
)
.
apply(
lambda
x:x
.
sample(
int
(np
.
rint(N
*
len
(x)
/
len
(df)))))
In [ ]:
Out[18]:
ID
program
0
1
Stats
1
2
Stats
2
3
Math
3
4
Math
4
5
Math
5
6
Math
6
7
Math
7
8
Math
ID program
0
1
Stats
ID program
5
6
Math
7
8
Math
4
5
Math
Out[22]:
ID
program
4
5
Math
2
3
Math
3
4
Math
0
1
Stats
Related Documents
Related Questions
Give an statistical principle example for a surgeon
arrow_forward
PDo Homework - Section 7.2 - Personal - Microsoft Edge
https://www.mathxl.com/Student/PlayerHomework.aspx?homeworkld%3585708584&questionld31&flushed=false&cld%36320940...
Winter 2021 Statistics-Rio (1)
Alyssa Ruiz & | 01/22/21 12:54 PM
Homework: Section 7.2
Save
Score: 0 of 1 pt
7 of 8 (2 complete) ▼
HW Score: 20.83%, 1.67 of 8 pts
7.2.29-T
Question Help ▼
An IQ test is designed so that the mean is 100 and the standard deviation is 14 for the population of normal adults. Find the sample size necessary to estimate the mean IQ score of statistics students such that it can be said with 90% confidence that the
sample mean is within 2 IQ points of the true mean. Assume that o = 14 and determine the required sample size using technology. Then determine if this is a reasonable sample size for a reál world calculation.
The required sample size is. (Round up to the nearest integer.)
Enter your answer in the answer box and then click Check Answer.
Check Answer
1 part
remaining
Clear All
arrow_forward
QUESTIION 5
You may need to use the appropriate appendix table or technology to answer this question.
An air transport association surveys business travelers to develop quality ratings for transatlantic gateway airports. The maximum possible rating is 10. Suppose a simple random sample of 50 business travelers is selected and each traveler is asked to provide a rating for a certain airport. The ratings obtained from the sample of 50 business travelers follow.
6
4
7
8
7
7
6
3
3
9
10
4
8
7
8
8
5
9
4
8
4
3
8
5
6
4
4
4
8
3
5
5
2
5
9
9
8
4
8
9
9
4
9
7
8
3
10
9
9
6
Develop a 95% confidence interval estimate of the population mean rating for this airport. (Round your answers to two decimal places.)
to
arrow_forward
P Do Homework - HW 9.3 - Google Chrome
A mathxl.com/Student/PlayerHomework.aspx?homeworkld=605947537&questionld=6&flushed=false&cld=6632484¢erwin=yes
Math 127 - Section 1002 - Fall 2021
Alyssa Prendergast
| 12/05/21 5:50 PM
= Homework: HW 9.3
HW Score: 70%, 14 of 20 points
O Points: 0 of 3
Question 7, 9.3.55
Save
i0
Write the expression in rectangular form, x + y i, and in exponential form, re
(V5 - i)8
The rectangular form of the given expression is , and the exponential form of the given expression is
(Simplify your answers. Use integers or decimals for any numbers in the expressions. Round the final answer to three decimal places as needed. Round all intermediate values to
four decimal places as needed.)
i
e
More
Textbook
Ask my instructor
Print
Clear all
Check answer
ENG
5:50 PM
P Type here to search
51°F Mostly cloudy
US 12/5/2021
29
近
arrow_forward
Distinguish between random sampling and systematic sampling. For each sampling type, give an example where you can apply it.
arrow_forward
com/static/nb/ui/evo/index.html?deploymentld%3D57211919418147002668187168&elSBN=9781337114264&id%3D900392331&snapshotld%3D19233498
GE MINDTAP
Q Search this course
-ST 260
Save
Submit Assignment for Grading
ons
Exercise 08.46 Algorithmic
« Question 10 of 10
Check My Work (4 remaining)
eBook
The 92 million Americans of age 50 and over control 50 percent of all discretionary income. AARP estimates that the average annual expenditure on restaurants and carryout
food was $1,876 for individuals in this age group. Suppose this estimate is based on a sample of 80 persons and that the sample standard deviation is $550. Round your
answers to the nearest whole numbers.
a. At 99% confidence, what is the margin of error?
b. What is the 99% confidence interval for the population mean amount spent on restaurants and carryout food?
C. What is your estimate of the total amount spent by Americans of age 50 and over on restaurants and carryout food?
million
d. If the amount spent on restaurants and…
arrow_forward
Give real life examples of simple random sampling and stratified sampling.
arrow_forward
Can you please help with this question
“Lampshells” are special shellfish animals with fossil records going back 600 million years. Little information is available about present-day populations of lampshells. While generally rare, they are abundant around parts of New Zealand, especially a particular species called “mermaid toenails”. They are seen in shallow water or washed up on beaches. Using scuba diving equipment, the researchers selected a random quadrat (2m x 2m) from a surveyed beach and scooped up all the sediment in that quadrat to a depth of 30cm. On shore, the live and dead shells were sorted. They measured the length of every “mermaid toenail” in each group: live and dead. The dead shells may be expected to be older on average and so longer. There appeared to be a difference in length, maybe due to the longevity of the shells.
the data is presented in the image attached
a) Does this design use paired data or independent samples? Explain.
b) Are the conditions satisfactory…
arrow_forward
mathxl.com/Student/PlayerHomework.aspx?homeworkld3608090538&questionld31&flushed%=false&cld%=66690718
Joshua Mitc
P Do Homework- Section 3.3 Subtraction of Whole Numbel
Assignments
i openvellu
YouT
MAT 1723 FA21
pps M Gmail
HW Score: 0%, 0 of 23 pol
O Points: 0 of 1
Question 1, 3.3.A-3
E Homework: Section...
Part 2 of 2
Lab Math
MGCCC
One fact in a fact family is 6+7= 13. Complete parts (a) and (b) below.
a. What are the other three members of this fact family?
Select all that apply.
iupi Gall Cont Communty College
O B. 13+6=7
YA. 13-7=6
OC. 6-7=6+(-7)
My Courses
O D. 7-6= - (6- 7)
Course Home
YF. 13-6=7
O E. 6+7+13= 0
YG. 7+6=13
O H. 7+13= 13+7
eText Contents
b. Draw four number line models to illustrate each of the number facts in part (a).
Assignments
Identify the number fact that corresponds to the question mark in each graph.
Student Gradebook
6
Video & Resource
Library
6
13
13
StatCrunch
0 246 8 10 12
0246 8 10 12
0246 8 1012
Study Plan
Accessible Resources
Help Me Solve This…
arrow_forward
Give and explain one way to find minimum variance unbiased estimator.
arrow_forward
Give me an example of systematic sampling and simple random sample
arrow_forward
Edit View History Bookmarks People Window Help
nd Masteri
ps://open
Do Homework - Jaelyn Dancy
xl.com/Student/PlayerHomework.aspx?homeworkld-515742856&questionld-18flushed-false&cld-5
Wright MTH112 20217 E-Learning Spring 2019
Jaelyn Dancy
Homework: HW - 2.4
ath score: 0 of 1 pt
2.4.43
11 of 12 (10 complete)
HW Sco
2
Solve the equation x+2x -5x-6 0 given that 2 is a zero of fx)x
2x-5x -6
The solution set is. (Use a comma to separate answers as needed.)
Enter your answer in the answer box and then click Check Answer.
All parts showing
Clear All
5
MacBook Pro
arrow_forward
The variablilty of statistics is described by
arrow_forward
Study groups are equivalent means that they are
exactly the same number of male and female participants in each group
statistically the same because sampling error is eliminated
experimentally the same
statistically equal, with small differences between groups due to sampling
error
arrow_forward
Is sampling always reliable?
arrow_forward
It is not important to collect samples randomly.
True or false
arrow_forward
A'6
arrow_forward
Find the mean and variance of S2(attached)
arrow_forward
What is the difference? Please lay out the rules.
arrow_forward
8https://ezto.mheducation.com/ext/map/index.html?_con=con&external_browser=D0&launchUrl=https%253A%252F%252Fnet
omework: Chapter 8 (Sections 8.1 through 8.5) G
Saved
0
Calculate the standard error. May normality be assumed? (Round your answers to 4 decimal places.)
Standard Error
Normality
(a) n 27, T = 0.32
%3D
ts
(b) n= 58, 7 = 0.45
n = 119 , TT = 0.39
Skipped
(c)
n 359, T = 0.004
(d)
eBook
Ask
Print
References
Mc
Graw
Hill
arrow_forward
Do HomewOrk
mathxl.com/Student/PlayerHomework.aspx?homeworkld%3D608090538&questionld3D1&flushed%=false&cld36669071¢erwin:
MAT 1723 FA21
E Homework: Section 3.3 Subtraction of Whole Num...
Question 20, Mathematic...
Betsy found 518 – 49 = 469. She was not sure she was correct so she tried to check her answer by adding 518 + 49. How could you help her?
Choose the correct answer below.
O A. Betsy is checking her subtraction the correct way.
O B. Betsy should add 518 + 469 to check her subtraction.
O C. Betsy should add 469 + 49 to check her subtraction.
O D. The only way to check subtraction is to do the original problem again.
Textbook
Ask My Instructor
P Type here to search
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
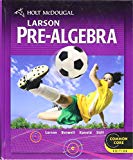
Holt Mcdougal Larson Pre-algebra: Student Edition...
Algebra
ISBN:9780547587776
Author:HOLT MCDOUGAL
Publisher:HOLT MCDOUGAL
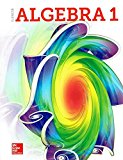
Glencoe Algebra 1, Student Edition, 9780079039897...
Algebra
ISBN:9780079039897
Author:Carter
Publisher:McGraw Hill
Related Questions
- Give an statistical principle example for a surgeonarrow_forwardPDo Homework - Section 7.2 - Personal - Microsoft Edge https://www.mathxl.com/Student/PlayerHomework.aspx?homeworkld%3585708584&questionld31&flushed=false&cld%36320940... Winter 2021 Statistics-Rio (1) Alyssa Ruiz & | 01/22/21 12:54 PM Homework: Section 7.2 Save Score: 0 of 1 pt 7 of 8 (2 complete) ▼ HW Score: 20.83%, 1.67 of 8 pts 7.2.29-T Question Help ▼ An IQ test is designed so that the mean is 100 and the standard deviation is 14 for the population of normal adults. Find the sample size necessary to estimate the mean IQ score of statistics students such that it can be said with 90% confidence that the sample mean is within 2 IQ points of the true mean. Assume that o = 14 and determine the required sample size using technology. Then determine if this is a reasonable sample size for a reál world calculation. The required sample size is. (Round up to the nearest integer.) Enter your answer in the answer box and then click Check Answer. Check Answer 1 part remaining Clear Allarrow_forwardQUESTIION 5 You may need to use the appropriate appendix table or technology to answer this question. An air transport association surveys business travelers to develop quality ratings for transatlantic gateway airports. The maximum possible rating is 10. Suppose a simple random sample of 50 business travelers is selected and each traveler is asked to provide a rating for a certain airport. The ratings obtained from the sample of 50 business travelers follow. 6 4 7 8 7 7 6 3 3 9 10 4 8 7 8 8 5 9 4 8 4 3 8 5 6 4 4 4 8 3 5 5 2 5 9 9 8 4 8 9 9 4 9 7 8 3 10 9 9 6 Develop a 95% confidence interval estimate of the population mean rating for this airport. (Round your answers to two decimal places.) toarrow_forward
- P Do Homework - HW 9.3 - Google Chrome A mathxl.com/Student/PlayerHomework.aspx?homeworkld=605947537&questionld=6&flushed=false&cld=6632484¢erwin=yes Math 127 - Section 1002 - Fall 2021 Alyssa Prendergast | 12/05/21 5:50 PM = Homework: HW 9.3 HW Score: 70%, 14 of 20 points O Points: 0 of 3 Question 7, 9.3.55 Save i0 Write the expression in rectangular form, x + y i, and in exponential form, re (V5 - i)8 The rectangular form of the given expression is , and the exponential form of the given expression is (Simplify your answers. Use integers or decimals for any numbers in the expressions. Round the final answer to three decimal places as needed. Round all intermediate values to four decimal places as needed.) i e More Textbook Ask my instructor Print Clear all Check answer ENG 5:50 PM P Type here to search 51°F Mostly cloudy US 12/5/2021 29 近arrow_forwardDistinguish between random sampling and systematic sampling. For each sampling type, give an example where you can apply it.arrow_forwardcom/static/nb/ui/evo/index.html?deploymentld%3D57211919418147002668187168&elSBN=9781337114264&id%3D900392331&snapshotld%3D19233498 GE MINDTAP Q Search this course -ST 260 Save Submit Assignment for Grading ons Exercise 08.46 Algorithmic « Question 10 of 10 Check My Work (4 remaining) eBook The 92 million Americans of age 50 and over control 50 percent of all discretionary income. AARP estimates that the average annual expenditure on restaurants and carryout food was $1,876 for individuals in this age group. Suppose this estimate is based on a sample of 80 persons and that the sample standard deviation is $550. Round your answers to the nearest whole numbers. a. At 99% confidence, what is the margin of error? b. What is the 99% confidence interval for the population mean amount spent on restaurants and carryout food? C. What is your estimate of the total amount spent by Americans of age 50 and over on restaurants and carryout food? million d. If the amount spent on restaurants and…arrow_forward
- Give real life examples of simple random sampling and stratified sampling.arrow_forwardCan you please help with this question “Lampshells” are special shellfish animals with fossil records going back 600 million years. Little information is available about present-day populations of lampshells. While generally rare, they are abundant around parts of New Zealand, especially a particular species called “mermaid toenails”. They are seen in shallow water or washed up on beaches. Using scuba diving equipment, the researchers selected a random quadrat (2m x 2m) from a surveyed beach and scooped up all the sediment in that quadrat to a depth of 30cm. On shore, the live and dead shells were sorted. They measured the length of every “mermaid toenail” in each group: live and dead. The dead shells may be expected to be older on average and so longer. There appeared to be a difference in length, maybe due to the longevity of the shells. the data is presented in the image attached a) Does this design use paired data or independent samples? Explain. b) Are the conditions satisfactory…arrow_forwardmathxl.com/Student/PlayerHomework.aspx?homeworkld3608090538&questionld31&flushed%=false&cld%=66690718 Joshua Mitc P Do Homework- Section 3.3 Subtraction of Whole Numbel Assignments i openvellu YouT MAT 1723 FA21 pps M Gmail HW Score: 0%, 0 of 23 pol O Points: 0 of 1 Question 1, 3.3.A-3 E Homework: Section... Part 2 of 2 Lab Math MGCCC One fact in a fact family is 6+7= 13. Complete parts (a) and (b) below. a. What are the other three members of this fact family? Select all that apply. iupi Gall Cont Communty College O B. 13+6=7 YA. 13-7=6 OC. 6-7=6+(-7) My Courses O D. 7-6= - (6- 7) Course Home YF. 13-6=7 O E. 6+7+13= 0 YG. 7+6=13 O H. 7+13= 13+7 eText Contents b. Draw four number line models to illustrate each of the number facts in part (a). Assignments Identify the number fact that corresponds to the question mark in each graph. Student Gradebook 6 Video & Resource Library 6 13 13 StatCrunch 0 246 8 10 12 0246 8 10 12 0246 8 1012 Study Plan Accessible Resources Help Me Solve This…arrow_forward
- Give and explain one way to find minimum variance unbiased estimator.arrow_forwardGive me an example of systematic sampling and simple random samplearrow_forwardEdit View History Bookmarks People Window Help nd Masteri ps://open Do Homework - Jaelyn Dancy xl.com/Student/PlayerHomework.aspx?homeworkld-515742856&questionld-18flushed-false&cld-5 Wright MTH112 20217 E-Learning Spring 2019 Jaelyn Dancy Homework: HW - 2.4 ath score: 0 of 1 pt 2.4.43 11 of 12 (10 complete) HW Sco 2 Solve the equation x+2x -5x-6 0 given that 2 is a zero of fx)x 2x-5x -6 The solution set is. (Use a comma to separate answers as needed.) Enter your answer in the answer box and then click Check Answer. All parts showing Clear All 5 MacBook Proarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Holt Mcdougal Larson Pre-algebra: Student Edition...AlgebraISBN:9780547587776Author:HOLT MCDOUGALPublisher:HOLT MCDOUGALGlencoe Algebra 1, Student Edition, 9780079039897...AlgebraISBN:9780079039897Author:CarterPublisher:McGraw Hill
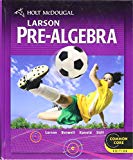
Holt Mcdougal Larson Pre-algebra: Student Edition...
Algebra
ISBN:9780547587776
Author:HOLT MCDOUGAL
Publisher:HOLT MCDOUGAL
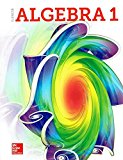
Glencoe Algebra 1, Student Edition, 9780079039897...
Algebra
ISBN:9780079039897
Author:Carter
Publisher:McGraw Hill