HW10-solutions
html
keyboard_arrow_up
School
University of Texas *
*We aren’t endorsed by this school
Course
230
Subject
Statistics
Date
Apr 3, 2024
Type
html
Pages
9
Uploaded by sjobs3121
Homework 10 for ISEN 355 (System Simulation)
¶
(C) 2023 David Eckman
Due Date: Upload to Canvas by 9:00pm (CDT) on Friday, April 21.
In [ ]:
# Import some useful Python packages.
import numpy as np
import matplotlib.pyplot as plt
import scipy.stats
import pandas as pd
Problem 1. (23 points)
¶
This problem concerns generating Beta($a=6, b=3$) random variates via acceptance-
rejection.
(a) (4 points)
Use scipy.stats.beta.rvs()
to generate 1000 Beta random variables
with parameters $a=6$ and $b=3$. Documentation for the Beta distribution in scipy.stats
can be found here
. Plot a histogram of the 1000 random variates with 30 bins and density=True
. Superimpose the probability density function (pdf) of a Beta ($a=6, b=3$) random variable.
In [ ]:
# Generate 1000 Beta(a=6, b=3) random variates.
beta_rvs = scipy.stats.beta.rvs(a=6, b=3, size=1000)
plt.hist(beta_rvs, bins=30, density=True)
# Evalute pdf of Beta (a=6, b=3) on a fine grid.
x = np.linspace(0,1,101)
pdf = scipy.stats.beta.pdf(x, a=6, b=3)
plt.plot(x, pdf)
plt.xlabel(r"$x$")
plt.ylabel(r"Relative Frequency / pdf $f(x)$")
plt.title(r"PDF of Beta($a=6, b=3$) and Histogram")
plt.show()
(b) (5 points)
Calculate $M = \max_{x \in [0, 1]} f(x)$ where $f(\cdot)$ is the pdf of the Beta($a=6, b=3$) distribution. You may use the fact that the mode
of the Beta distribution is $(a-1)/(a+b-2)$ provided $a > 1$ and $b > 1$. The scipy.stats.beta.pdf()
function may prove useful. As a means of double-checking your answer, plot the pdf $f$ (the same pdf you plotted in part (a)) and superimpose a horizontal line at a height $M$ using matplotlib.pyplot.hlines()
; documentation found here
.
In [ ]:
a = 6
b = 3
x_mode = (a - 1) / (a + b - 2)
# Evalute pdf at the mode.
M = scipy.stats.beta.pdf(x_mode, a=6, b=3)
plt.plot(x, pdf)
plt.hlines(M, 0, 1, 'r')
plt.xlabel(r"$x$")
plt.ylabel(r"pdf $f(x)$")
plt.title(r"pdf of Beta(a=6, b=3) with upper bound")
plt.show()
(c) (2 points)
Recall that a Beta random variable (with any values of $a$ and $b$) takes values on the interval $[0, 1]$. Using your answer from part (b), what is the expected number of Uniform[0, 1] random variates needed by an acceptance-rejection
algorithm (with the choice of $M$ you specified in part(b)) to generate a single Beta($a=6, b=3$) random variate?
In [ ]:
# From class notes, expected number of uniforms needed to generate one RV is 2 * c
# where c = M * (upper bound - lower bound) is the area of the box.
# Lower and upper bounds of Beta rv are 0 and 1.
c = M * (1 - 0)
print(f"The expected number of Uniform[0, 1] random variates needed is {np.round(2 * c,
3)}.")
The expected number of Uniform[0, 1] random variates needed is 5.1.
(d) (3 points)
For the acceptance-rejection method described in part (c), what is the probability that it terminates on the first iteration (i.e., that it uses only 2 Uniform[0, 1]
random variates) AND returns Beta random variate $X$ having a value less than or equal to 0.5?
\begin{align*} P(\text{success on first iteration and } X \leq 0.5) &= P(Z_2 \leq f(Z_1) \
text{ and } Z_1 \leq 0.5) \\ &= P(Z_1 \leq 0.5 | Z_2 \leq f(Z_1)) \times P(Z_2 \leq f(Z_1))
\\ &= F(0.5) \times (1/c) \end{align*}
This is essentially the numerator term in the proof of the validity of the acceptance-
rejection method.
In [ ]:
print(f"The requested probablity is {np.round(scipy.stats.beta.cdf(0.5, a=6, b=3) / c, 3)}.")
The requested probablity is 0.057.
(e) (6 points)
Write a function that generates a single Beta($a=6, b=3$) random
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
variate via acceptance-rejection, as described in Lecture 13. You may either work directly with the random variates $Z_1$ and $Z_2$ discussed in the slides, or with the more primitive Uniform[0, 1] random variates $U_1$ and $U_2$.
In [ ]:
def generate_beta(a, b):
# Optional... can use global variable M from part (b).
x_mode = (a - 1) / (a + b - 2) M = scipy.stats.beta.pdf(x_mode, a=a, b=b)
accept = False
while not accept:
# Generate 2 Uniform[0,1] random variates.
U1 = scipy.stats.uniform.rvs()
U2 = scipy.stats.uniform.rvs()
# Transform into Z1 ~ Uniform[0,1] and Z2 ~ Uniform[0,M].
Z1 = 0 + (1 - 0) * U1 # Z1 = U1 in this case
Z2 = 0 + (M - 0) * U2 # Z2 = MU2
# Alternatively, directly generate Z1 and Z2.
# Z1 = scipy.stats.uniform.rvs(loc=0, scale=1)
# Z2 = scipy.stats.uniform.rvs(loc=0, scale=M) if Z2 <= scipy.stats.beta.pdf(Z1, a=a, b=b):
# accept Z1 as Beta(a,b) random variable
return U1 # This will break the loop.
(f) (3 points)
Use your function from part (e) to generate 1000 Beta($a=6, b=3$) random variates via acceptance rejection. Plot a histogram of the 1000 random variates with 30 bins and density=True
. Superimpose the probability density function (pdf) of a Beta ($a=6, b=3$) random variable.
In [ ]:
beta_AR_rvs = [generate_beta(a=6, b=3) for _ in range(1000)]
plt.hist(beta_AR_rvs, bins=30, density=True)
x = np.linspace(0,1,101)
pdf = scipy.stats.beta.pdf(x, a=6, b=3)
plt.plot(x, pdf)
plt.xlabel(r"$x$")
plt.ylabel(r"Relative Frequency / pdf $f(x)$")
plt.title(r"PDF of Beta($a=6, b=3$) and Histogram")
plt.show()
Problem 2. (27 points)
¶
Recall the file arrival_counts.csv
from Homework 8. It contains counts of the number of vehicles that arrived in 1-hour periods from 8am-4pm over the course of 30 days. The code below loads the data and stores it in a numpy ndarray
, which you can think of as a matrix. Each row in the matrix corresponds to a day and each column corresponds to a 1-hour period, starting with 8am-9am in Column 0 (remember that Python indexes from 0) and 3-4pm in Column 7.
In [ ]:
# Import the dataset.
mydata = pd.read_csv('arrival_counts.csv')
arrivalcounts = np.array(mydata)
print(arrivalcounts)
[[253 380 307 179 118 174 162 77]
[248 350 329 155 121 169 134 62]
[285 384 310 161 103 169 135 64]
[291 406 301 167 124 171 164 67]
[246 396 302 169 128 155 135 77]
[264 383 314 185 115 153 132 43]
[256 352 337 166 133 164 137 64]
[255 381 304 192 120 178 152 53]
[267 396 318 176 117 166 137 48]
[253 390 316 181 119 174 176 70]
[266 356 322 195 118 165 142 60]
[263 346 305 174 118 185 123 61]
[284 360 322 166 114 159 141 74]
[255 385 293 167 121 169 125 61]
[259 400 313 198 108 183 133 56]
[249 397 331 177 123 175 124 59]
[269 367 322 175 112 145 123 54]
[293 384 320 165 115 188 146 59]
[256 393 328 186 122 134 125 60]
[261 343 331 202 121 156 139 52]
[269 376 314 194 135 154 158 71]
[270 388 295 155 141 144 155 58]
[239 367 311 177 116 177 148 61]
[264 384 314 177 130 154 139 57]
[250 382 332 175 132 192 150 60]
[278 367 327 192 124 165 128 77]
[262 366 339 197 124 173 151 64]
[261 362 303 186 128 180 150 74]
[262 345 348 169 126 173 136 66]
[238 345 316 179 135 186 154 55]]
(a) (10 points)
Write a function that generate a day's worth of vehicle arrival times from the fitted NSPP using the thinning method discussed in class. Use the estimated arrival rate function that varies by hour
, i.e., the one you fitted in Problem 1(l) of Homework 8.
In [ ]:
def parking_NSPP_thinning():
hourly_rates = np.mean(arrivalcounts, axis=0)
lambda_star = max(hourly_rates)
T_star = 0 arrival_times = [] # Accepted arrival times
while T_star < 8: # Simulate arrival up until 4pm (=8).
A = scipy.stats.expon.rvs(scale = 1/lambda_star)
T_star = T_star + A
U = scipy.stats.uniform.rvs()
if T_star < 8: # T_star may have gone past 8 within the loop.
if U <= hourly_rates[int(np.floor(T_star))] / lambda_star:
arrival_times.append(T_star)
return arrival_times
(b) (5 points)
Run your function from part (a) 30 times to produce 30 days' worth of arrival times. For each day's worth of arrival times, use np.histogram()
to count the number of arrivals in each of the eight 1-hour intervals: 8am-9am, 9am-10am, etc. Using np.mean()
, calculate the mean counts for each 1-hour interval, averaged over 30 days. Plot the mean arrival count by interval as a piecewise-constant function with 8 pieces. Superimpose the fitted rate function, which is another piecewise-constant function with 8 pieces. Comment on what you see in the plot. NOTE: This problem and the rest in the assignment ask you to calculate statistics from your 30 days' worth of generated arrivals, not from the data in arrival_counts.csv
.
In [ ]:
all_arrival_times = [parking_NSPP_thinning() for _ in range(30)]
hourly_counts = [list(np.histogram(all_arrival_times[idx], bins=np.linspace(0, 8, 9))
[0]) for idx in range(30)]
mean_counts = np.mean(hourly_counts, axis=0) # Simulated data.
hourly_rates = np.mean(arrivalcounts, axis=0) # Original data.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
# Optional: Append duplicate end value to make plot look nice.
mean_counts_app = list(mean_counts) + [mean_counts[-1]]
hourly_rates_app = list(hourly_rates) + [hourly_rates[-1]]
t = np.arange(9)
plt.step(t, mean_counts_app, where='post')
plt.step(t, hourly_rates_app, where='post')
plt.legend(["Simulated", "Original"])
plt.xlabel("Time of Day")
plt.ylabel("Number of Arrivals")
plt.title("Mean Hourly Arrival Rates/Counts")
plt.xticks(ticks=[0, 1, 2, 3, 4, 5, 6, 7, 8], labels=["8a", "9a", "10a", "11a", "12p", "1p", "2p", "3p", "4p"])
plt.show()
The mean hourly arrival count and fitted hourly rate function look almost identical.
(c) (4 points)
Similar to what you did for Problem 1(i) on Homework 8, use np.var()
to compute the variance of the 30 generated arrival counts for each 1-hour period
. Use
plt.scatter()
to produce a scatter plot of (sample mean, sample variance) with 8 points, one for each 1-hour period. Superimpose a 45-degree line passing through the origin. Comment on whether your plot shows evidence that the arrival counts you generated satisfy the mean=variance assumption of a Poisson process.
In [ ]:
var_counts = np.var(hourly_counts, axis=0, ddof=1)
plt.scatter(mean_counts, var_counts)
plt.plot([0, 400], [0, 400], 'r')
plt.xlabel("Sample Means")
plt.ylabel("Sample Variances")
plt.title("Mean vs Variances")
plt.show()
The scatter points fall roughly along the 45 degree line through the origin. For a Poisson process, the number of arrivals in any interval is Poisson distributed, and so will have mean equal to variance. This scatterplot supports this, but with only 30 replications, there is still a fair amount of variability in the sample mean and sample variance.
(d) (3 points)
Similar to what you did for Problem 1(e-f), use the function np.corrcoef()
to compute the sample correlation of generated arrival counts. Print the correlation matrix and use the function np.round()
to round the entries to the nearest 3 digits. Comment on what you observe in the correlation matrix and what you
would expect to observe for a non-stationary Poisson process. (Hint: Think about the independent increments assumption.)
In [ ]:
hourly_counts= np.array(hourly_counts)
corr_matrix = np.corrcoef(hourly_counts, rowvar=False)
print(np.round(corr_matrix,3))
[[ 1. 0.019 -0.014 0.054 -0.075 -0.237 0.416 0.118]
[ 0.019 1. -0.184 0.244 -0.183 -0.432 -0.039 -0.115]
[-0.014 -0.184 1. -0.25 -0.026 -0.009 0.139 0.026]
[ 0.054 0.244 -0.25 1. -0.188 -0.143 -0.03 -0.063]
[-0.075 -0.183 -0.026 -0.188 1. 0.226 0.031 -0.029]
[-0.237 -0.432 -0.009 -0.143 0.226 1. -0.039 -0.203]
[ 0.416 -0.039 0.139 -0.03 0.031 -0.039 1. 0.024]
[ 0.118 -0.115 0.026 -0.063 -0.029 -0.203 0.024 1. ]]
Most off-diagonal correlations in the matrix are closer to zero than to +/-1. For a Poisson process, the increments are independent, so we should expect to see a
correlation matrix that is close to the identity matrix. We are seeing something that, but there is still amount of variability with only 30 replications.
(e) (5 points)
For this NSPP, on average, how many Uniform[0, 1] random variates does your thinning algorithm need to generate one arrival time? You may assume that in Step 2 of the thinning method, when an exponential random variate is needed, one can be generated (via inversion) from exactly one Uniform[0, 1] random variate. (Hint: Think about the ratio of the expected number of green stars to the expected number of black stars on Slide 6 of the Lecture 14 slides.)
The number of black stars is Poisson distributed with mean $8\lambda^*$, so the expected number of black stars is $8\lambda^*$. Similarly, the number of green starts
in time interval $i$ is Poisson distributed with mean $\lambda_i$ where $\lambda_i$ is the hourly rate associated with that interval. Thus the expected number of green stars in interval $i$ is $\lambda_i$, and the expected total number of green stars is $\
sum_{i=1}^8 \lambda_i$. We use one Uniform[0,1] random variate to generate each black star and another to decide whether it becomes a green star. Thus the average number of Uniform[0,1] random variates needed to generate one arrival time is
$$ \frac{2 \times 8 \times \lambda^*}{\sum_{i=1}^8 \lambda_i}. $$ In [ ]:
hourly_rates = np.mean(arrivalcounts, axis=0)
lambda_star = max(hourly_rates)
print(f"The average number of Uniform[0, 1] RVs per arrival is {round(2 * 8 * lambda_star / np.sum(hourly_rates), 3)}.")
The average number of Uniform[0, 1] RVs per arrival is 3.685.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
# 4
arrow_forward
Answer the question below in the picture
arrow_forward
In IBM SPSS, what does clicking on this icon do?
arrow_forward
Bb Upload Assignment: Final Exam X
Math 2207 Eve Final Exam Fall
Answered: Evaluate O A. None X
Mail - AURIBEL.GARCIACEBALI X
A dropbox.com/s/zsxcvpdj3nxsm10/Math%202207%20Eve%20Final%20Exam%20Fall%202020%20v2.pdf?dl=0
a
Math 2207 Eve Final Exam Fall 2020 v2.pdf
Let f(x) be given by
9x - 27
x 3
where K is an unknown constant. What value should K be replaced
with so that f(x) is continuous for all X?
O A. 18
There is no value of K that will work, because
O B. lim f(x)# lim f(x).
x + 3-
X + 3+
Oc 9
O D. 4
There is no value of K that will work, because
O E.
undefined when X = 3.
9x - 27
is
X- 3
...
+
arrow_forward
tleby
Dashboard - Chaffey College
HW 12
A Math 81, HW 12, Tavakoli, Sp22, X
Math 81, HW 12, Tavakoli, Sp22. X
PDF
File C:/Users/toria/Downloads/Math%2081,%20HW%2012,%20Tavakoli,%20Sp22,%20nc.pdf
Q To
rmissions. You may not have access to some features. View permissions
3.Find the rank and nullity of the matrix A by reducing it to row echelon
form.
1
-2
1
-1
-3
1
A =
-2
-1
1
-1
1
3 0 -4
3.
3.
arrow_forward
Please answer the question below in the photo
arrow_forward
can you please help with how to insert it into the calculator
arrow_forward
Please help
arrow_forward
M Inbox (219) - z5wei@ucsd.
A My Course - Math 20C - M X
1| Introduction to MATLA X
A MyLab and Mastering
O Do Homework - Homework x
Course Home
+
A mathxl.com/Student/PlayerHomework.aspx?homeworkld%3D611989718&questionld%3D1&flushed%3Dfalse&cld%3D6731743¢erwin=yes
海 2
Update
Math 18 Fall 2021
Zixin Wei & | 10/10/21 10:45 PM
HW Score: 34.78%, 8 of 23 points
O Points: 0 of 1
Question 9, 1.4.10
E Homework: Homework 2
Save
Part 1 of 2
Write the system first as a vector equation and then as a matrix equation.
4x1 - X2 = 9
6x, + 5x2 = 2
9x1 - X2 = 1
Write the system as a vector equation where the first equation of the system corresponds to the first row, the second equation of the system corresponds to the second row, and the third equation of the system corresponds to the third row. Select
the correct choice below and fill in any answer boxes to complete your choice.
O A. X1
+ X2
В.
X1 X2
X4
Oc.
X2
arrow_forward
Táb
Window
Help
mk
X X Goodbye from Edgenuity
Reading in Math Slides and For x
Gabriella Gascon - Reading in
tation/d/1gos78upXoYQQMOaOkp_0QPhYZQSZXdfe4WVQngHHXYo/edit#slide=id.SLIDES_API430018234_15
e - Roblox
P
Home - Peacock E Tubi
Harry Potter Shop...
W Wizarding World:..
Disney+ | Movies...
n the Middle School Math Classroom - Digital Version ☆ D
Slide Arrange Tools Add-ons Help
Last edit was 16 minutes ago
Background
Layout -
Theme
Transition
I II 2
3
6.
PRACTICE
Drag a circle to the correct answer.
3.
A $220 pair of shoes is on sale for $154. What is the percent change in the cost of the shoes?
A. 66% decrease
Did the price of the shoes go up or down? That should help you eliminate two
choices. So... Are you finding the change in the dolllar amount or the percent
B. 66% increase
change? (hint hint)
C. 30% increase
D. 30% decrease
Thn cort ofa $750 refriaerator was increased by 10%. It was later dropped by 10%. What is the new cost
arrow_forward
Sahar Rasoul-Math 7 End of Yea X Gspy ninjas book-Google
docs.google.com/spreadsheets/d/1j5MotWzsc0V1V3Qyl4rbP_OFOUotaNXCIIFax>
Copy of Copy of Col...
8.8
Sahar Rasoul - Math 7 End of Year Digital Task Cards Student Version ☆
File Edit View Insert Format Data Tools Extensions Help Last edit was 5 minu
$ % .0 .00 123 Century Go... ▼ 18 Y BIS
fx| =IF(B4="Question 1", Sheet2! H21, if(B4="Question 2", Sheet2! H22, IF(B4="
n
100%
36:816
A
B
C
6
16
A flashlight can light
a circular area of up
to 6 feet in diameter.
What is the maximum
area that can be lit?
Round to the nearest
tenth.
30x
0004
15
A Sheet1
https://www.google.com/url?sa=i&url=https%3A%2F%2Fwww.amazon.com%2FSpy-Ninjas-Ultimate-Guidebook-Scholastic%2Fdp
7
8
9
10
11
12
13
14
3
5.
7.
a
5
$9
A
arrow_forward
still having a hard time figuring out how to insert it into the calculator
arrow_forward
Answer 5
arrow_forward
O Course Home
Do Homework - Section 11.1 H X
b My Questions | bartleby
+
A mathxl.com/Student/PlayerHomework.aspx?homeworkld=598208830&questionld%3D6&flushed%3Dtrue&cld%3D6521194¢erwin=yes
Update
E Apps
O New Tab
O YouTube
O myLoneStar Login
y angel 2pi - - Imag.
E Reading List
Math 2412-5009 Summer Il-2021
Abdel Alhammouri & | 08/10/21 11:25 PM
= Homework: Section 11.1 Homework
Question 10, 11.1.49
Save
Next Question
Solve the system of equations. If the system has no solution, say that it is inconsistent.
X -
y -
z =
-x + 2y - 3z = -6
3x -
y - 11z = -7
Select the correct choice below and, if necessary, fill in any answer boxes within your choice.
A. The solution is x=
.y3D
and z =
(Type integers or simplified fractions.)
B. There are infinitely many solutions. The solution set is {(x,y,z) x=
(Simplify your answers. Type expressions using z as the variable.)
z is any real number}.
y3D
O C. There are infinitely many solutions. The solution set is (x,y,z) | x=
(Simplify your answer.…
arrow_forward
O Mail - Joshua Walters - Outlook x
Bb Assignment 6
Content
WA Homework 8.4 - MATH 2584, seci X
9 Fast Track It - Bidfta.com
+
i webassign.net/web/Student/Assignment-Responses/last?dep=25827866
* E
E Apps
O Uark Email 23 HotSchedules
GE Google News W Homework 3.2 - M...
6 Citrix Receiver
Blackboard
eAt
Need Help?
Read It
3. [-/1 Points]
DETAILS
ZILLDIFFEQ9 8.4.005.
MY NOTES
ASK YOUR TEACHER
PF
Use (1) in Section 8.4
X = eAtc (1)
to find the general solution of the given system.
1 0
X' =
8
X(t) =
Need Help?
Watch It
Read It
4. [-/1 Points]
DETAILS
ZILLDIFFEQ9 8.4.007.
MY NOΤ
ASK YOUR TEACHER
PF
Uce (1) in Soction O A
3:21 PM
O Type here to search
4/6/2021
arrow_forward
96
R.
%24
2.
6 Assignment Player
DI Mathway Algebra Problem Sof
6 Dashboard
Classwork for Algebra 2- 2nd Hr x
の
A bigideasmath.com/MRL/public/app/#/student/assessment;isPlayerWindow=true;assignmentld=6d65d536-44d7-41ba-959f-cu3
Maintenance Alert
The Big Ideas Math online platform will be unavailable on January 22nd, from 7:00 to 10:00 am ET. Thank you for your
patience while we update our system.
Myles Burrell
IG IDEAS MATH
#2
A juggler tosses a ball into the air. The ball leaves the juggler's hand 3 feet above the ground and has an initial velocity of 20
feet per second. The juggler catches the ball when it falls back to a height of 4 feet. How long is the ball in the air? Hint: Use
-167 + vt + h
Previous
Next
1.
N
BEANOCK452
Coa
f7
f8
OLI
f12
prt s
LL
break
%5
& 7 7
%24
80
8.
66
4.
5.
*-
9O
K 2 L3
H.
oW N
目
arrow_forward
e Mind My Courses
Classes
C A
a westerly.agilemind.com/LMS/Imswrapper/LMS.html#/C/course_ms_math7_ccss/CCSS%20Mathematics%207/a/9ebf2ace-9d45-11eb-9958-0cc47a69..
Central
6 Test Player
Bb Westerly Middle Sc..
Login- Powered by..
A Classes
K! Play Kahootl - Ente...
M Agile Mind
M McGraw-Hill Educat...
Free Google Slic
Geometry Topic 12 MP 6-10
More practice (page 9)
Two sides of a triangle have lengths 15 and 20. The length of the third side can be any number greater than 35
A and less than
B.
Hint
Subn
The Triangle Inequality Conjecture says that the sum of the lengths of any two sides of a triangle is greater than the length of the third side. What is the I
third side could be? What is the greatest value?
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
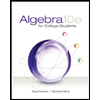
Algebra for College Students
Algebra
ISBN:9781285195780
Author:Jerome E. Kaufmann, Karen L. Schwitters
Publisher:Cengage Learning
Algebra & Trigonometry with Analytic Geometry
Algebra
ISBN:9781133382119
Author:Swokowski
Publisher:Cengage
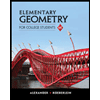
Elementary Geometry for College Students
Geometry
ISBN:9781285195698
Author:Daniel C. Alexander, Geralyn M. Koeberlein
Publisher:Cengage Learning
Related Questions
- Bb Upload Assignment: Final Exam X Math 2207 Eve Final Exam Fall Answered: Evaluate O A. None X Mail - AURIBEL.GARCIACEBALI X A dropbox.com/s/zsxcvpdj3nxsm10/Math%202207%20Eve%20Final%20Exam%20Fall%202020%20v2.pdf?dl=0 a Math 2207 Eve Final Exam Fall 2020 v2.pdf Let f(x) be given by 9x - 27 x 3 where K is an unknown constant. What value should K be replaced with so that f(x) is continuous for all X? O A. 18 There is no value of K that will work, because O B. lim f(x)# lim f(x). x + 3- X + 3+ Oc 9 O D. 4 There is no value of K that will work, because O E. undefined when X = 3. 9x - 27 is X- 3 ... +arrow_forwardtleby Dashboard - Chaffey College HW 12 A Math 81, HW 12, Tavakoli, Sp22, X Math 81, HW 12, Tavakoli, Sp22. X PDF File C:/Users/toria/Downloads/Math%2081,%20HW%2012,%20Tavakoli,%20Sp22,%20nc.pdf Q To rmissions. You may not have access to some features. View permissions 3.Find the rank and nullity of the matrix A by reducing it to row echelon form. 1 -2 1 -1 -3 1 A = -2 -1 1 -1 1 3 0 -4 3. 3.arrow_forwardPlease answer the question below in the photoarrow_forward
- can you please help with how to insert it into the calculatorarrow_forwardPlease helparrow_forwardM Inbox (219) - z5wei@ucsd. A My Course - Math 20C - M X 1| Introduction to MATLA X A MyLab and Mastering O Do Homework - Homework x Course Home + A mathxl.com/Student/PlayerHomework.aspx?homeworkld%3D611989718&questionld%3D1&flushed%3Dfalse&cld%3D6731743¢erwin=yes 海 2 Update Math 18 Fall 2021 Zixin Wei & | 10/10/21 10:45 PM HW Score: 34.78%, 8 of 23 points O Points: 0 of 1 Question 9, 1.4.10 E Homework: Homework 2 Save Part 1 of 2 Write the system first as a vector equation and then as a matrix equation. 4x1 - X2 = 9 6x, + 5x2 = 2 9x1 - X2 = 1 Write the system as a vector equation where the first equation of the system corresponds to the first row, the second equation of the system corresponds to the second row, and the third equation of the system corresponds to the third row. Select the correct choice below and fill in any answer boxes to complete your choice. O A. X1 + X2 В. X1 X2 X4 Oc. X2arrow_forward
- Táb Window Help mk X X Goodbye from Edgenuity Reading in Math Slides and For x Gabriella Gascon - Reading in tation/d/1gos78upXoYQQMOaOkp_0QPhYZQSZXdfe4WVQngHHXYo/edit#slide=id.SLIDES_API430018234_15 e - Roblox P Home - Peacock E Tubi Harry Potter Shop... W Wizarding World:.. Disney+ | Movies... n the Middle School Math Classroom - Digital Version ☆ D Slide Arrange Tools Add-ons Help Last edit was 16 minutes ago Background Layout - Theme Transition I II 2 3 6. PRACTICE Drag a circle to the correct answer. 3. A $220 pair of shoes is on sale for $154. What is the percent change in the cost of the shoes? A. 66% decrease Did the price of the shoes go up or down? That should help you eliminate two choices. So... Are you finding the change in the dolllar amount or the percent B. 66% increase change? (hint hint) C. 30% increase D. 30% decrease Thn cort ofa $750 refriaerator was increased by 10%. It was later dropped by 10%. What is the new costarrow_forwardSahar Rasoul-Math 7 End of Yea X Gspy ninjas book-Google docs.google.com/spreadsheets/d/1j5MotWzsc0V1V3Qyl4rbP_OFOUotaNXCIIFax> Copy of Copy of Col... 8.8 Sahar Rasoul - Math 7 End of Year Digital Task Cards Student Version ☆ File Edit View Insert Format Data Tools Extensions Help Last edit was 5 minu $ % .0 .00 123 Century Go... ▼ 18 Y BIS fx| =IF(B4="Question 1", Sheet2! H21, if(B4="Question 2", Sheet2! H22, IF(B4=" n 100% 36:816 A B C 6 16 A flashlight can light a circular area of up to 6 feet in diameter. What is the maximum area that can be lit? Round to the nearest tenth. 30x 0004 15 A Sheet1 https://www.google.com/url?sa=i&url=https%3A%2F%2Fwww.amazon.com%2FSpy-Ninjas-Ultimate-Guidebook-Scholastic%2Fdp 7 8 9 10 11 12 13 14 3 5. 7. a 5 $9 Aarrow_forwardstill having a hard time figuring out how to insert it into the calculatorarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Algebra for College StudentsAlgebraISBN:9781285195780Author:Jerome E. Kaufmann, Karen L. SchwittersPublisher:Cengage LearningAlgebra & Trigonometry with Analytic GeometryAlgebraISBN:9781133382119Author:SwokowskiPublisher:CengageElementary Geometry for College StudentsGeometryISBN:9781285195698Author:Daniel C. Alexander, Geralyn M. KoeberleinPublisher:Cengage Learning
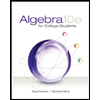
Algebra for College Students
Algebra
ISBN:9781285195780
Author:Jerome E. Kaufmann, Karen L. Schwitters
Publisher:Cengage Learning
Algebra & Trigonometry with Analytic Geometry
Algebra
ISBN:9781133382119
Author:Swokowski
Publisher:Cengage
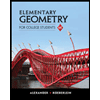
Elementary Geometry for College Students
Geometry
ISBN:9781285195698
Author:Daniel C. Alexander, Geralyn M. Koeberlein
Publisher:Cengage Learning