Assessment 1 Guidelines
docx
keyboard_arrow_up
School
TAFE NSW - Sydney Institute *
*We aren’t endorsed by this school
Course
ICT50178
Subject
Computer Science
Date
Jan 9, 2024
Type
docx
Pages
22
Uploaded by AdmiralPheasantPerson135
Assessment 1 Guidelines:
Task 1:
New dynamic type that avoid compile time type checking
The dynamic types are resolved at runtime instead of compile time
Compiler skips the type checking for dynamic type
It does not give any error about dynamic types at compile time
A method can have parameters of the dynamic type
Data can be declare dynamic type Output: Your result is 21 X 12= 252
Task 2:
It deals with automatic garbage collections.
It has a lot of standard libraries.
Some properties and events can make programming smarter.
Delegates and concepts of event management can also help in making the language strong.
It supports Multi-threading.
It has the concepts of Indexers
Generic concepts are easy to use in C
Task 3:
Flowchart:
START
Get the price
Calculate Total price
Get Discount %
Calculate Discount Price
Pseudocode:
Start
Get the price list
Calculate total price
Get discount percentage Calculate discount price
Calculate discount payable price
Print price
End C# programming code:
using
System;
class
MainClass
{
public
static
void
Main
(
string
[] args) {
Console.WriteLine(
"Enter bill amount:"
);
int
bill = Convert.ToInt32(Console.ReadLine());
Console.WriteLine(
"Enter discount percentage:"
);
int
discount = Convert.ToInt32(Console.ReadLine());
int
afterDiscount = bill - (bill * discount / 100
);
Console.WriteLine(
"After discount your bill is: "
+ afterDiscount);
}
}
Output:
Calculate Total Bill
Print Bill
END
Enter bill amount:
5000
Enter discount percentage:
10
After discount your bill is: 4500
Task 4: C# is a strongly typed language. It means we must declare the type of a variable which indicates the kind of values it is going to store such as integer, float, decimal, text, etc. The following declares and initialised variables of different data types. Example: Variables of Different
Data Types
string stringVar = "Hello there!!";
int intVar = 100;
float floatVar = 10.2f;
char charVar = 'A';
bool boolVar = true; There are a number of commonly used data types in C#.
int int stands for integer (i.e. numbers with no decimal or fractional parts) and holds numbers from - 2,147,483,648 to 2,147,483,647. Examples include 15, 407, -908, 6150 etc. byte
byte also refers to integral numbers but has a narrower range from 0 to 255. Most of the time, we use int instead of byte for integral numbers. However, if you are programming for a machine that has limited memory space, you should use byte if you are certain the value of the variable will not exceed the 0 to 255 range. For instance, if you need to store the age of a user, you can use the byte data type as it is unlikely that the user’s age will ever exceed 255 years old. Float
float refers to floating point numbers, which are numbers with decimal places such as 12.43, 5.2 and - 9.12. float can store numbers from -3.4 x 1038 to +3.4 x 1038. It uses 8 bytes of storage and has a precision of approximately 7 digits. This means that if you use float to store a number like 1.23456789 (10 digits), the number will be rounded off to 1.234568 (7 digits). Double
double is also a floating-point number but can store a much wider range of numbers. It can store numbers from (+/-)5.0 x 10-324 to (+/-)1.7 x 10308 and has a precision of about 15 to 16 digits. double is the default floating point data type in C#. In other words, if you write a number like 2.34, C# treats it as a double by default. decimal
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
decimal stores a decimal number but has a smaller range than float and double. However, it has a much greater precision of approximately 28-29 digits. If your program requires a high degree of precision when storing non integral numbers, you should use a decimal data type. An example is when you are writing a financial application where precision is very important. Char
char stands for character and is used to store single Unicode characters such as ‘A’, ‘%’, ‘@’ and ‘p’ etc. bool bool stands for boolean and can only hold two values: true and false. It is commonly used in control flow statements.
Task 5:
The main
benefits
one can gain from
using asynchronous programming
are improved
application
performance and responsiveness.
One particularly well suited
application
for the
asynchronous pattern
is providing a responsive UI in a client
application
while running a computationally or resource expensive Asynchronous programming is
a
means
of parallel
programming
in which a unit of work runs separately from the main application thread and notifies the calling thread of its completion, failure or progress
synchronous programming
static
void
Main
(
string
[] args)
{
Coffee cup = PourCoffee();
Console.WriteLine(
"coffee is ready"
);
Egg eggs = FryEggs(2);
Console.WriteLine(
"eggs are ready"
);
Bacon bacon = FryBacon(3);
Console.WriteLine(
"bacon is ready"
);
Toast toast = ToastBread(2);
ApplyButter(toast);
ApplyJam(toast);
Console.WriteLine(
"toast is ready"
);
Juice oj = PourOJ();
Console.WriteLine(
"oj is ready"
);
Console.WriteLine(
"Breakfast is ready!"
);
}
Asynchronous programming
static
async
Task Main
(
string
[] args)
{
Coffee cup = PourCoffee();
Console.WriteLine(
"coffee is ready"
);
Egg eggs = await
FryEggs(2);
Console.WriteLine(
"eggs are ready"
);
Bacon bacon = await
FryBacon(3);
Console.WriteLine(
"bacon is ready"
);
Toast toast = await
ToastBread(2);
ApplyButter(toast);
ApplyJam(toast);
Console.WriteLine(
"toast is ready"
);
Juice oj = PourOJ();
Console.WriteLine(
"oj is ready"
);
Console.WriteLine(
"Breakfast is ready!"
);
}
Task 6:
C# 8.0 adds the following features and enhancements to the C# language:
Readonly members
Default interface methods
Pattern matching enhancements:
Switch expressions
Property patterns
Tuple patterns
Positional patterns
Using declarations
Static local functions
Disposable ref structs
Nullable reference types
Asynchronous streams
Indices and ranges
Null-coalescing assignment
Unmanaged constructed types
Stackalloc in nested expressions
Enhancement of interpolated verbatim strings
There are two main themes to the C# 7.3 release
.
One theme provides features that enable safe code to be as performant as unsafe code. The second theme provides incremental improvements to existing features. In addition, new compiler options were added in this release.
The following new features support the theme of better performance for safe code:
You can access fixed fields without pinning.
You can reassign ref local variables.
You can use initializers on stackalloc arrays.
You can use fixed statements with any type that supports a pattern.
You can use additional generic constraints.
The following enhancements were made to existing features:
You can test == and != with tuple types.
You can use expression variables in more locations.
You may attach attributes to the backing field of auto-implemented properties.
Method resolution when arguments differ by in has been improved.
Overload resolution now has fewer ambiguous cases.
The new compiler options are:
-publicsign to enable Open Source Software (OSS) signing of assemblies.
-pathmap to provide a mapping for source directories.
Task 7:
Software prototype Tools Usually, there are few common types of software prototyping:
Software prototyping is much the same as prototyping in the border product design field. It is a necessary step involved in daily software design. Normally, after doing enough UX research like gathering idea, data, information, demands, evaluation, then it’s time to build a prototyping.
The basic software stage including:
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Idea Generation;
Demand Generation;
Function and Structure;
Prototyping Stage;
PRD (replaced by prototyping for most occasions);
Road Map
1.
Paper prototyping
- this is mostly for self-usage, to record ideas;
2.
Rapid prototyping
- the most demanding prototypes, wide range of application;
3.
High-fidelity prototyping
- emphasis interactions and user interface, for stockholders & development team;
4.
Low-fidelity prototyping
- emphasis interactions and thoughts, for design teams;
As you see, except paper prototyping, all the rest need to use a prototyping tool. For your better decisions, I will introduce the tool from several aspects:
Cost:
How much will you spend?
Compatibility:
Is the tool for Mac or Windows or both?
Key features:
What can it do?
Learning Curve:
How long it takes you to get started?
Usage pattern:
Are you prototyping websites, mobile apps, desktop apps, or all of the above?
Speed:
How quick can you finish design on the prototyping tool?
Fidelity:
What is the requirement of your prototypes fidelity? Wireframes or low-fidelity or high-fidelity?
Sharing:
Collaboration is key when it comes to design. A must consideration.
User’s feedback:
See what others say.
To help you select the best prototyping tool for you and your business, here’s a list of five of the most popular, affordable, and accessible solutions you can use today. By no means is this list comprehensive, but we’ve compiled some of our favorites for your
consideration.
1.
InVision
: best prototyping tool for building exceptional products in one, connected workflow
InVision makes it easy to create interactive prototypes. Simply upload static screenshots and create clickable prototypes your users can interact with and understand. The app runs on the web and works well simplifying the workflow between designers and other stakeholding teams.
Create rich interactive prototypes that allow for rapid iteration
Seamlessly communicate, gather feedback, and advance projects
Compatibility with most popular graphics file formats like PNG, GIF, PSD, JPG, and others
Pricing: Free to $100/month
2.
Balsamiq
: best prototyping tool for building wireframes
Balsamiq helps you quickly design mockups that are great for sketching and wireframing. With excellent ease of use, a great widget library, and its cloud-based software, it makes team collaboration easy.
Allows for quick wireframing so you can learn fast and fail faster
Minimal learning curve with powerful technology
Drag and drop simplicity
Pricing: $9-$199/month, discounts for annual subscriptions
3.
Justinmind
: best all-in-one prototyping tool for web and mobile apps
Justinmind is an all-in-one prototyping tool for web and mobile apps that helps you build wireframes to highly interactive prototypes without any coding. Justinmind lets you design from scratch and leverage a full range of web interactions and mobile gestures, so you can focus on building exceptional user experiences.
Responsive prototyping that ensures your designs adapt to fit multiple screen resolutions
Prototype smart forms and data lists without writing code
Free, ready-made, regularly-updated UI kits for web and mobile
Pricing: $19-$49/month, discounts for annual subscriptions\
4.
Figma
: best prototyping tool for designing collaboratively
Figma is truly a one-tool solution for all of your design needs. Thanks to real-time collaboration, web-based functionality, and exceptional price-to-value, Figma is rising through the ranks and gaining traction with design teams.
Easy switching between design and prototype modes
Quick sharing and real-time feedback
Powerful editing features
Pricing: Free to $45/month. Some plans are available with annual pricing only.
5.
Adobe XD
: best prototyping tool for enterprise business
Wireframing, designing, prototyping, presenting, and sharing amazing experiences for web, mobile, voice, and more— Adobe
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
XD is your all in one app. Adobe XD provides you access to all your assets in one place, eliminates tedious manual tasks, create experiences that are adaptable to any size screen, and
integrates seamlessly with the UserTesting platform
.
High-fidelity interactions
Integration with the other Adobe products you already use
Real-time viewing, commenting, and sharing capabilities
Task 8:
Quality assurance
(
QA
) fundamentals must be in place, including the
development
of a
quality
plan.
QA's
role is to ensure that the project is
developed
in compliance with the defined processes and that the processes defined are adequate for the project. ... It enhances communication among project members and customers.
-
Best
Quality Assurance Practices
in an Agile
Software Development
Process. it makes the
development
process time and
cost
-effective. and faster in a new system and
development
cycles become
rapid
.
Agile
development methods
are similar to
rapid application
... segments of the
development
life cycle, such as
development
,
quality assurance
, and operations. That produces a high-quality system with low investment
costs
.
Task 9:
The success of Rapid Application Development is contingent upon the involvement
of
people with the right skills and talents
. ...
Rapid development
usually allows each
person involved
to play several different roles, so a
RAD
project mandates a great degree of cooperative effort among a relatively small
group of people
.
By utilizing a rapid application development method, designers and developers can aggressively utilize knowledge and discoveries gleaned during the development process itself to shape the design and or alter the
software direction entirely.
User Involvement: which requires the design team to discuss with users what features or implementations might be required and plan specifications around those ideas, a rapid prototype allows users to actually use the software and provide feedback on a live system, rather than attempting to provide abstract evaluations of a design document.
Feasibility: development team require feasibility analysis to quickly evaluate the feasibility of a particularly complex or risky component right
out of the gate. By recognizing and working on complicated systems early in the development lifecycle, the software will be more robust, less error-
prone, and better structured for future design additions.
Error Reduction & Debugging: Team require Testing and quality assurance skills With rapid prototype releases during a project,
it is far more likely that errors will be both discovered (and subsequently squashed) far earlier in the development cycle than with a typical waterfall approach.
Task 10: It depends on some of the following factors:
1.
Which type of the project?
2.
What are the requirements?
3.
What will be the time duration?
4.
What is the problem and what is the solution for that problem?
5.
What are the tools to be used?
6.
Enhancements
and so on…
Following are some of the methodologies for software development
Waterfall Model
Prototype Methodology
Agile Software Development Methodology
Rapid Application Development
Dynamic System Development Model Methodology
Spiral Model
Extreme Programming Methodology
Feature Driven Development
Joint Application Development Methodology
Lean Development Methodology
...
Task 11
Technological Fit
First, we identify any unique business or technical requirements that lend themselves to a particular language. All programming languages have trade-offs, so our primary focus is understanding what a client’s specific business challenges are and then selecting a language that best aligns with their individual needs.
Architectural Environment
We take into account what, if any, physical environment the client is currently operating in or what they may aspire to be in. For example, if they already own a large Microsoft-based server environment, that may push us towards choosing a Microsoft-based solution. If the client is looking to move to a cloud/
PaaS
environment, we may suggest a platform that can more easily be containerized. In addition, since the software language can dictate size and scalability options
in the long run, we will also factor those concerns into our decision.
Overall Client Fit
While we like to engage with our clients long-term, we understand that at some point we may need to transition a codebase to another team. We take into account access to and availability of
the necessary skill sets to ensure the long-term sustainability of the codebase.
Key Differences Amongst Languages
With so many programming languages to choose from, it’s best to start thinking in broad functional terms before narrowing in on specific language. We’ve highlighted some of the key differences and use cases for a few of the most popular languages.
Mobile App Development
When it comes to mobile, we’ll start by discussing trade-offs between a native options and hybrid/JS solutions. We recommend native languages (like Objective-C/Swift for iOS or Java for Android) in order to provide the best user experience and app longevity combined with less maintenance. On the other hand, many clients opt for hybrid/JS solutions (like React-Native) as they can reduce the overall cost and time-to-market.
Web App Development
For a web application that has unique needs, but also common components like identity management, we will likely discuss a C# or JS-based framework leveraging standard web frameworks like .Net or Node/Express. Need to solve very unique business challenges, or rapidly
prototype? We might start with Python or Go to speed up the time-to-market. If we’re working on embedded firmware, then we’re likely using C++.
Other Factors to Consider
The selection of the programming language itself is only part of the equation. As you move up the technology stack, you can have very different uses of the programming language with different frameworks. For example, AngularJS (Client side MVC framework) is very different than Node.JS (server side runtime environment). Typically, the decision as to what language we use is as much dictated by the frameworks chosen.
It’s important to note that in a post-monolithic software world, these decisions aren’t mutually exclusive within an application ecosystem. We are increasingly implementing microservice architectures so that we can run individual services built on disparate programing languages and frameworks. In that way, we can ensure that each specific task is being served by the best possible platform. With our breadth of experience and expertise, we feel confident that we can guide our clients to the best software platform.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
The variety of programming languages available to software developers today is really impressive. While their popularity and usage change from year to year, leaders stay the same: JavaScript, PHP, Java, C# and Python. The picture below demonstrates coding languages ranking as of today based on Stack Overflow stats:
Needless to say that each language has own advantages and disadvantages and it's business critical to choose the right language for your project depending on your mid and long-term goals and overall expectations.
I've compiled a list of recommendations for non-tech companies and startups that are looking to kick off their development project while still having doubts about what language / technology to choose for it.
What Are the Questions to Ask When Choosing a Programming
Language?
This is by no means a comprehensive list of questions. But it’s a list that can serve as a starting point to choose the programming language that you’ll need.
1. Does the language have proper ecosystem support? Will the language survive for the long haul? Is there vendor support provided for the language?
2. What’s the environment that the project will run on — web, mobile, etc.?
3. Are there infrastructure considerations to using the programming language, such as new hardware required? What are the deployment considerations?
4. Does the client prefer a certain programming language to be used?
5. Are there specific libraries, features, and tools for a programming language that are the industry standard for this type of project?
6. Can our developer program in this language? Do we need to hire new developers? Can our developers learn the new programming language quickly?
7. What are the constraints of your project that are non-negotiable — time, budget, resources?
8. What are the performance considerations of the project? Will the language accommodate the benchmarks and the performance?
9. Are there any legacy codebase considerations for the project?
10.
Are there any interface issues with upstream and downstream systems or external systems?
11.
Does it need to integrate with third-party tools?
12.
Are there any security considerations?
Task 12:
Rapid application development (RAD
)
is a type of agile development methodology. While waterfall development methods focus on requirement assessment and strict planning, RAD is more about incorporating user feedback into every step of the development process.
With a waterfall model, it becomes extremely difficult to modify any core features of the software once it is in the testing phase.
RAD helps solve that very problem by offering more flexibility. It prioritizes rapid prototyping and feedback during the development and testing phases. It is also much
easier to make multiple revisions to the applications without the need to redo everything and start from scratch.
Some of the many benefits of a RAD platform include:
Quick iterations lead to faster delivery
Swift changes and modifications during development
Lower development and maintenance costs, as compared to traditional development methods
Easier integrations with other software
How to Choose The Right RAD Platform for Your Organization
While picking RAD over other methodologies is an obvious choice, picking the right RAD platform can be a rather overwhelming task. A good way to start is by identifying all the key requirements that an ideal RAD platform should offer. Here are
six that you can’t afford to ignore.
1. Visual development support
The RAD platform you choose should have an accessible UI that allows you to develop applications with little to
no coding. In fact, it’s best to pick a RAD tool that has visual drag-and-drop features along with UI integration.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
At the same time, the tool shouldn’t completely exclude frontend developers, and allow them to make changes wherever required.
2. Quick deployment, modification, and prototyping
The RAD tool should be able to automatically deploy applications from the development environment to production, saving your team a lot of time. It should also be able to support different staged activities for testing purposes.
The tool should make it easy for you to check all the revisions made by the different team members, and rollback any changes with a simple click of a button.
At the same time, there should be a built-in provision for automated prototyping and wireframing so that you can have a clear idea about how the design will look before finalizing anything.
3. Easy reusability
In order to decrease the development time and deploy applications quickly, the RAD tool you choose should have the option to reuse previously built applications or its parts including workflows, forms, components, services, and templates.
4. Support for mobile apps
While the exact type of mobile support offered will differ from one RAD tool to another, it should at least be able to provide at least minimal support for mobile web apps. However, it may be best to pick RAD platforms that provide support for JavaScript and HTML5 in order to ensure your mobile hybrid applications run smoothly.
5. Seamless team collaboration
Choose a RAD platform that focuses on agile teams to make it easier for your IT and
business teams to collaborate while developing applications. From managing tasks to reviewing code, messaging, and reporting, there are a lot of collaboration features offered by RAD platforms and the right one will depend on your exact requirements.
6. Real-time analytics
User feedback is an essential part of rapid application development and that is why it’s important to choose a RAD platform that offers features like built-in analytics, reporting, traceability, and app management which would, in turn, help you improve adoption and usability.
Next Step: Find Your Perfect RAD Platform
The next step is to evaluate RAD platforms carefully and choose the one that meets your organization’s current and future needs best.
Kissflow
is a no-code RAD platform that allows you to automate business processes.
It offers all the necessary features you will require to develop and deploy applications
at a fast pace.
Here are five rapid application development tools that stand out from the crowd.
1. Zoho Creator
Zoho Creator is one of the most popular rapid development tools on the market, and for good reason. It’s got a solid list of features to create business web tools and customize them any way you like.
One of the biggest features is a drag-and-drop interface, allowing even layman business users to create apps
without having to know how to code. Inexperienced users use pre-built modules that have specific functionalities they want.
There are also tools for collecting data, designing personal workflows, and setting up
unique rules for each new application.
All of this makes Zoho Creator one of the most popular rapid application development tools for web development on the market.
2. Kissflow One of the best rapid application development tools on the market,
Kissflow
is a complete business process management suite
designed to let you create your own processes and workflows, or customize pre-built templates according to your needs.
Kissflow believes in keeping everything as simple and easy to use as possible. That’s reflected in the software, which is designed from the ground up to be easy to use for everyone.
It’s got a single sign-on feature, allowing you to work from anywhere and using any device.It even has Android and iOS apps that you can access through your smartphone.
Built-in advanced reporting and analytical tools give you all the information you need.
This removes the need for additional reporting tools when you need metrics to calculate and measure performance.
Another great Kissflow feature is the
ability to integrate with third-party tools
using Zapier. So, if you like using a particular tool, Kissflow will seamlessly integrate with it,
without
needing any additional coding.
In the ‘workflow management and business process management suite’ space, few alternatives do it as well as Kissflow does.
3. OutSystems
OutSystems is a platform designed to work with
RAD methodology
. It gives developers the high-end tools they need to create, deploy, and manage enterprise-
grade applications quickly and efficiently.
One thing that makes OutSystems stand out from other rapid application development tools is the fact that it takes full advantage of integrations, and makes it
easy to connect with other tools. If you prefer using multiple systems for other parts of your work, OutSystems will easily integrate with those tools to give you an easier development experience.
When it comes to rapid application development tools, OutSystems shines because it encompasses the rapid application development philosophy of quick prototyping with constant feedback and communication to build each function and feature.
OutSystems’ ability to create enterprise applications also means that it provides total end-to-end security for the applications you create and run through the platform. This
means no complicated intranet setup or hassling keys. All you need is a single user ID and password to log in, and you’re ready to go.
4. Bizagi
A portmanteau of the words “business” and “agility”, Bizagi certainly lives up to its name. It’s an extremely useful rapid application development tool. Bizagi is a
BPM suite
used to automate business processes and create customized workflows.
It’s loaded with a plethora of benefits–a process simulator, designer, tools for collaborating with your team, form builder, and more. You can also create your own custom reports and analytics to measure your productivity growth.
If you’re in the market for a business process management suite that covers your rapid application development needs as well, this is worth looking into.
5. Appian
Appian gives a lot of importance to quick and easy development. This makes it one of the most well-known rapid application development tools on the market.
As a BPM suite, it offers solutions that are more drag-and-drop than sit-and-code. This makes it incredibly convenient for developers and users who aren’t familiar with coding.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
In addition to this, it also allows seamless integration with third-party tools of your choosing. Appian is scalable according to your needs, whether you’re a company with 10 users or 10,000 users.
Conclusion
Rapid application development tools are a dime a dozen in the market, but the trick is to find something that works. Some tools aren’t tailored to your workflow, and some tools don’t offer particular features that you’d be looking for. And that’s where you have to do this the old-fashioned way, trying out one product at a time until you find something that clicks.
Kissflow
is a great place to start your search. You’re getting a rapid application development tool that can be customized to your particular workflows, and you can create and customize apps according to your specific needs.
Disclaimer: All registered trademarks, company names and brand names used on this website are the property of their respective owners.
Task 13:
int[,] array = new int[4, 2]; Task 14:
Binary search
in
C#
Binary search works
on a sorted array. The value is compared with the middle element of the array. If equality is not found, then the half part is eliminated in which the value is not there.
Binary search works on a sorted array. The value is compared with the middle
element of the array. If equality is not found, then the half part is eliminated in which
the value is not there. In the same way, the other half part is searched.
Here is the mid element in our array. Let’s say we need to find 62, then the left part
would be eliminated and the right part is then searched:
Binary search
.
Binary search
is an efficient algorithm for finding an item from a sorted list of items. It
works
by repeatedly dividing in half the portion of the list that
could
contain the item, until you've narrowed down the possible locations to just one.
Worst-case space complexity. O(1) In computer science,
binary search
, also known as half-
interval
search
, logarithmic
search
, or
binary
chop, is a
search algorithm
that finds the position of a target value within a sorted array.
Binary search
compares the target value to the middle element of the array.
Task 15:
If you want to write multiple classes in separate source code files, you can compile them together
as shown in this tutorial.
1. Write the "Util" class in a source code file called "Util.cs":
// Util.cs
// Copyright (c) 2015, HerongYang.com, All Rights Reserved.
public class Util {
public static void Swap(ref string x, ref string y) {
string o = x;
x = y; y = o;
}
}
2. Write the "SwapTest" class in a source code file called "SwapTest.cs":
// SwapTest.cs
// Copyright (c) 2015, HerongYang.com, All Rights Reserved.
using System;
public class SwapTest {
public static void Main() {
string a = "Herong";
string b = "Yang";
Console.WriteLine("{0} {1}", a, b);
Util.Swap(ref a, ref b);
Console.WriteLine("{0} {1}", a, b);
}
}
3. If you compile "SwapTest.cs" by itself, you will get an error:
C:\herong>\Windows\Microsoft.NET\Framework\v4.0.30319\csc SwapTest.cs
SwapTest.cs(10,7): error CS0103: The name 'Util' does not exist in the
current context
4. If you compile "SwapTest.cs" with "Util.cs" together, you will get the executable file generated correctly:
C:\herong>\Windows\Microsoft.NET\Framework\v4.0.30319\csc SwapTest.cs Util.cs
5. Run the executable file "SwapTest.exe", you will get the program output
C:\herong>SwapTest.exe
Herong Yang
Yang Herong
C# Program compilation steps are as follows.
1.
Compiling Source Code into Managed Module.
2.
Combining newly created managed module into the assembly / assemblies.
3.
Loading the CLR.
4.
Executing the assembly in & by CRL.
Every .NET program first compiles with an appropriate compiler like if we write a program in C# language then it get compiled by C# compiler (i.e. csc.exe).
In .NET framework every program executes (communicate) in an operating system by using CLR (Common Language Runtime).
Task 16:
Visual Studio is the best IDE for C#. C# and Visual Studio are products of Microsoft. It is designed to work perfectly with C#. It has many tools that work very well for C#. That being said, Visual Studio comes in free and paid versions. The free version is called the community edition and the paid version
is called the Enterprise and Professional edition. The Community edition is full of features and everything an independent developer needs. And in case you need a powerful IDE for your company, just get the Enterprise edition.
Experienced programmer and beginners are advised to look no further than just this. This is the best software that is out there to develop in any platform let alone C#. And since this is the best IDE for C# this is what everyone wants. Microsoft
Visual Studio
is an integrated development environment (IDE) from Microsoft. It is used to develop computer programs, as well as websites, web apps, web services and mobile apps. ...
Visual Studio
includes a code editor supporting IntelliSense (the code completion component) as well as code refactoring
Task 17:
Test case
writing is a major activity and considered as one of
the
most
important
parts of
software testing
.
It is
used by
the testing
team,
development team as well as
the
management. If
there
is no documentation for an application, we can use
test case
as a baseline document.
The key purpose of a
test case
is to ensure if different features within an application are working as expected. It helps
tester
, validate if the
software
is free of defects and if it is working as per the expectations of the end users.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Test case writing is a major activity and considered as one of the most important parts of software testing. It is used by the testing team, development team as well as the management. If there is no documentation for an application, we can use test case as a baseline document.
So why are test cases important?
A typical definition for test case is a ‘set of conditions under which a tester will determine whether an application or software system or one of its features is working as expected.’
It means that test cases clarify what needs to be done to test a system. It gives us the steps which we execute in a system, the input data values which we enter in the system along with the expected results when we execute a particular test case.
Test cases bring together the whole testing process. If the test cases are ready, they are really helpful to measure weather client expectations were fulfilled or not. When we execute test cases, we can get more defects which may be skipped in ad-hoc testing
.
-----------------------------------------
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Recommended textbooks for you
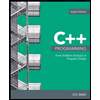
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
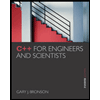
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
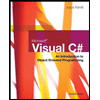
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
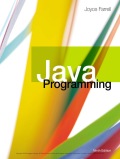
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
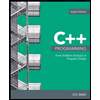
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
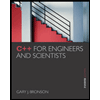
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
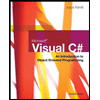
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
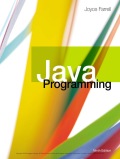
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage