CP4P_SDLC_Activity_Answers
docx
keyboard_arrow_up
School
Seneca College *
*We aren’t endorsed by this school
Course
101
Subject
Computer Science
Date
Apr 3, 2024
Type
docx
Pages
6
Uploaded by SargentWater35178
Computer Principles for Programmers APIs, SDLC, Version Control
Using Mozilla Firefox is strongly recommended for this Activity because it can transform JSON responses into a human readable format. (Raw Data > "Pretty Print") (#)
is
points for API and Time Zone questions.
1.
(5)
What is sent via the API from one system to another? What is sent back? API sends:
API stands for Application programming interface and allows different systems to communicate and interact with each other. One system sends a request to another system with API. This request includes specific information such as the request method, header, parameters, and body. Request methods can be GET, POST, PUT, DELETE, etc., and headers include metadata for the request. Parameters are additional data values sent along with the request to provide specific instructions. The
body contains the main data, and it usually is in a structured format like JSON or XML.
Sent back:
When a system receives the API request, processes it, and generates a response to it. This response
includes the status code, headers, and a body/payload. The status code is a number that shows the outcome of the request. For example, 200 means a successful request or 500 for server error. The header provides metadata about the response like content type, and authentication-related information. And body contains the actual data or message that is sent back as a response. 2.
(5)
Use api.agify.io to predict the age of a person using your given name and an ISO country code
API URL request:
https://api.agify.io/?name=Faeze&country_id=IR
JSON response:
{
"count": 86,
"name": "Faeze",
"age": 37,
"country_id": "IR"
}
3. (5)
Use the time zone API request at worldtimeapi.org API URL request:
http://worldtimeapi.org/api/timezone/America/Regina
JSON response:
{
"abbreviation": "CST",
"client_ip": "2607:fea8:3a9f:af70:bc47:ed72:e5d5:ca38",
"datetime": "2023-07-15T20:13:33.588872-06:00",
"day_of_week": 6,
"day_of_year": 196,
"dst": false,
"dst_from": null,
"dst_offset": 0,
"dst_until": null,
"raw_offset": -21600,
"timezone": "America/Regina",
"unixtime": 1689473613,
CPR101 Summer 2023 Week 9
Page 1 of 6
Computer Principles for Programmers APIs, SDLC, Version Control
"utc_datetime": "2023-07-16T02:13:33.588872+00:00",
"utc_offset": "-06:00",
"week_number": 28
}
4.
(16)
Using the above JSON data from worldtimeapi.org, fill in the JSON key / value pairs relating to the descriptions in the table below. See Response Schema
JSON key
JSON value
UTC date/time in ISO8601 format
utc_datetime
2023-07-
16T02:13:33.588872+00:00
Unix UTC timestamp
unixtime
1689473613
Unix UTC to location difference
utc_offset
-06:00
Location's daylight-saving time difference
dst
false
Location date/time in ISO8601 format
datetime
2023-07-15T20:13:33.588872-06:00
How do you calculate the location's
timestamp
from the UTC timestamp using JSON keys?
For converting the UTC timestamp to
the location's timestamp, we add the
raw offset to the UTC timestamp. In
this case, the raw offset is -21600
seconds (-06:00 hours). UTC timestamp: 1689473613
Raw offset: -21600 seconds
Unix UTC timestamp - Raw offset: 1689473613 + (-21600) = 1689452013
Calculated location timestamp value is:
1689452013
5.
(5)
How did you confirm that your location timestamp when converted to data/time was the same as the Location date/time in ISO8601 format in the JSON schema? Show your test and the result.
CPR101 Summer 2023 Week 9
Page 2 of 6
Computer Principles for Programmers APIs, SDLC, Version Control
SDLC – Software Development Life Cycle
54 points = 9 points × 6 items, 75+ words each
Determine
: First, I try to read and understand the assignment specification of the IPC class. For understanding, I
read the instruction carefully including any accompanying materials or guidelines. I try to take note of
any specific instructions or constraints mentioned like required language, or library. After that, I try to
identify key requirements and constraints. Make a list of the main tasks or actions that the program
should be able to perform. It means identifying input formats and noting any desired or mandatory
output formats or results that the program should generate. If I have any doubts or uncertainties
regarding the assignment requirements, I try to email my instructor to clear my doubts. By doing this, I
can identify the problem and methods that I can use to solve the problem or create a program. By doing these things, I can obtain a comprehensive understanding of the problem and identify the
key requirements. This will enable me to proceed with confidence and ensure that my solution aligns
with the assignment specifications.
Define: To completely understand the problem, I use the following stages to specify the exact requirements
and assure a clear understanding of all inputs, processes, and outputs. Analyze the workshop PDF
and break down it into smaller components or tasks to gain a better understanding of its structure. By
looking at the whole workshop and the expected output in the PDF, I try to identify the main things or
concepts involved in the workshop. I try to consider the relationships and interactions between
different components. For example, in workshop 8 for IPC, I did my best to understand the
relationship between main and functions and how can I implement them. Determine the inputs
required for the solution to function correctly. The most important part is identifying the sources of
these inputs, such as user input, file input, or API data. In IPC workshops input always are entered by
the user. Then I check the valid range for inputs by looking at the expected output and instruction.
Determine the expected format, structure, and range of valid inputs. Moreover, determining the
expected outputs or results that the solution should produce is very important. Design
: CPR101 Summer 2023 Week 9
Page 3 of 6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Computer Principles for Programmers APIs, SDLC, Version Control
The design step in the Software Development Life Cycle (SDLC) is the most important phase where
we can plan and outline the solution before diving into coding. It involves creating a blueprint or
roadmap for how the software will be developed. In the first step, I have to make the overall
architecture and structure of the workshop for myself. Identify the modules or components that need
to be developed and their relationships. The most important step is creating pseudocode or a
flowchart to document the algorithmic steps of my solution. Pseudocode is a high-level description of
the logic and functionality without getting into the syntax of a specific programming language. A
flowchart is a visual representation of the sequence of steps and decisions in the solution. Both tools
help me visualize and plan the implementation before writing actual code. Pseudocode and flowchart
enable me to understand how I should write code for my workshops. For example, how many loops
that I need or where can I define validation, etc. Writing comments before writing the actual code can
help me to clarify my thinking, articulate my intentions, and plan the structure of my code. By
commenting on the purpose of each function, module, or section of code, I can enhance the
readability and maintainability of my program.
Develop: In this stage, I have to use my design note, flowchart, or pseudocode and make them into actual
source code using C language. Based on my understanding I define variables at the first of the
program. Also, I try to include comments in my code to provide explanations, clarify the code's
purpose, and document any complex logic or algorithms. Comments make my code more
understandable and readable by other developers who may work on it in the future. Additionally, it is
useful because maybe I will want to refactor or change them in the future. For example, IPC
workshops include 3 parts. I use part one in part 2 and sometimes my comments help me to
understand the code and change it easier. After completing the code, testing is a critical aspect of the
process. I try to use outputs in the workshop and test my code. But in companies, I have to write unit
tests. But for my workshop, I use boundary values to test my code and program. If I face errors or
unexpected behavior during testing, it's time to debug my code. I try to use an IDE debugger and set
breakpoints. And stepping through the code execution to understand how the program behaves. Deliver
:
I try to follow these steps on the matrix server for submitting my project. I make sure that all necessary files, documentation, and any additional requirements are included. I always pay attention to submission deadlines and any specific instructions provided by my instructor. To submit IPC workshops, I use WinSCP and copy my file into the matrix. Then based on the instruction in PDF I run
a command in Putty to compile the code and with a command I sent it to my professor. If I face a problem with submitting, it means if I get an error, I download the desired output from WinSCP and compare it to my output. Most of the time my output has problems like space and uppercase letters. When I want to submit my workshop into the matrix, I have to enter some inputs to get the desired output. These user inputs are important as well for submission. We should enter the correct input same as in the PDF file. The submitter produces an outputmaster.txt and checks my output and all inputs that I enter together. It checks them character by character. If they do not match, the submitter cannot let me submit, and I try to enter the exactly input. Also, it gives us the line number of errors and we can check it. So, I run the command "~profName.proflastname/submit 144w6/NAA_p2". Then
I try to enter the correct input to make the desired output. So, the matrix will accept that, and I can submit my assignment. If I have another problem in my code, it shows me the number of lines and I can fix it. For the reflection part, there are some questions at the end of the workshop, and I answer them in a text file. These questions are about challenges, conceptual things, and technical obstacles. CPR101 Summer 2023 Week 9
Page 4 of 6
Computer Principles for Programmers APIs, SDLC, Version Control
And based on my code I try to answer these questions and explain how to implement this workshop and how can I fix errors or problems. D'oh
: To maintain a code, I try to provide a clear explanation of its functionality usage, and any
dependencies or assumptions. I write a document for my code and keep it beside my project. Also, I
tend to write comments inside my code for complex parts. Good documentation helps me to
understand what the program is, and it enables me to refactor my code in the future. Version control
is very helpful for maintaining projects, especially in companies. I utilized a version control system
(Git) and track the changes made to my code. For example, for my workshops from Wednesdays to
Mondays, I commit my changes regularly and provide a desire commit messages to highlight the
modifications made. So, after I complete the code, I can keep the last version of my code. Whenever I
need my code, I can fork it from Git and see my changes and comments. This allows you to revert to
previous versions if needed and provides a history of the code's evolution. I always try to Break down
my code into smaller, self-contained modules or functions that perform specific tasks. Each module
should have a well-defined responsibility, making it easier to understand, modify, and maintain.
Ensure that modules have clear interfaces and follow established design principles, such as low
coupling and high cohesion. All of these make my code more readable and make it easier to
maintain. Also, I try to review and refactor my code regularly to improve its design, decrease
redundancy, and enhance maintainability. Refactoring involves restructuring the code without
changing its external behavior. It helps keep the codebase clean, readable, and adaptable to future
changes.
Software Version
5 × 2 points each
A.
Question:
What is the name of the software and its current version? Answer:
The name of IDE that I am working with is Visual Studio. The version of this IDE is Version 16.11.26.
B. Question:
What do the components of the version number mean? Answer:
"16" represents the major version and indicates initial development. The major version number
typically represents significant updates, major releases, or major changes in the software. A change
in the major version often indicates compatibility issues with previous versions and may introduce
substantial feature enhancements or architectural changes.
"11" represents the minor. It usually includes new features, enhancements, and improvements.
"26" represents the Micro. It shows the specific build or release of the software.
C. Question:
CPR101 Summer 2023 Week 9
Page 5 of 6
Computer Principles for Programmers APIs, SDLC, Version Control
In what way would that software be forward-compatible? Answer:
The software, Visual Studio 2019, would be forward compatible in the sense that projects created or
developed using older versions of Visual Studio such as Visual Studio 2017 should be able to be
opened, compiled, and run without having problems in Visual Studio 2019. Visual Studio 2019 is
designed to be able to open and load projects created using older versions of Visual Studio. Also, it
supports the compilation of code written in various programming languages, such as C#, C++, and
Visual Basic. It is designed to handle code written in previous versions of these languages, ensuring
that projects created with older language versions can still be compiled and built successfully in
Visual Studio 2019. Moreover, Visual Studio 2019 includes support for different frameworks, libraries,
and platforms used in software development. It ensures to maintain compatibility with older versions
of these frameworks and platforms and this ability enables developers to continue working on projects
that rely on previous versions without major compatibility issues. Additionally, if you have an
application built with an earlier version, you can use Visual Studio 2019's debugging tools to analyze
and troubleshoot issues without having to migrate the entire project to the latest version.
D. Question:
In what way would that software be backward compatible? Answer:
Backward compatibility means that the software should be compatible with older versions or previous releases. For this case (Visual Studio), it means that projects and solutions created using older versions can be opened and worked on using version 16.11.26 without significant compatibility issues. The software ensures that it can handle and interpret code, project files, and dependencies from previous versions correctly.
E. Question:
Find the release notes (AKA changelog) for that software and include the URL, release date, and a description of one of the latest changes. Programmers wrote those notes. It is time someone read them, for example: Answer:
https://learn.microsoft.com/en-us/visualstudio/releases/2019/release-notes
released April 11th, 2023
- Fixed an issue in IIS Express that could cause a crash when updating telemetry data.
- Fixed a crash when invalid input is sent to the driver used during PGO training for kernel mode drivers.
CPR101 Summer 2023 Week 9
Page 6 of 6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Recommended textbooks for you
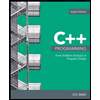
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
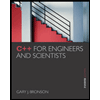
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
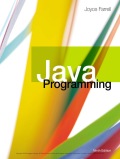
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
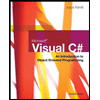
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
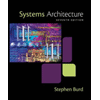
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
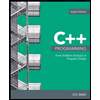
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
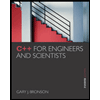
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
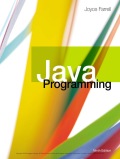
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
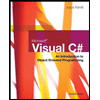
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
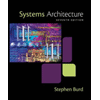
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage