[Coding Exercise 4] Billboard Top 100 Songs Charts
pdf
keyboard_arrow_up
School
Simon Fraser University *
*We aren’t endorsed by this school
Course
120
Subject
Computer Science
Date
Oct 30, 2023
Type
Pages
9
Uploaded by xiaofanfan
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
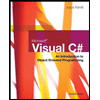
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
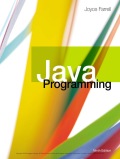
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
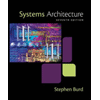
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
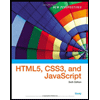
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
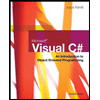
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
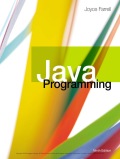
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
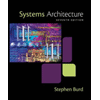
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
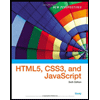
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Browse Popular Homework Q&A
Q: HO
dil. H3PO4
heat
Q: The Amish community has less deviancy and crime than large urban cities like Los Angeles. Why ?
Q: A stream of water from a fire hose with a flow rate of 320 gallons
per minute and a speed of 35 m/s…
Q: Remember to keep tabs on any and all databases that could include your
personal information. For how…
Q: Question 7
Which of the following compounds is an Arrhenius acid?
Ba(OH)2
KOH
Ca(OH)2
HBr
Q: Hello. Can you hand write the solution. I'm not understanding. It's not really clear. Thank you
Q: Which mechanisms are used by miRNAs to regulate
expression?
O Targeted degradation of mRNAs
O…
Q: % Create a program to plot the motion of the ping pong ball with drag.
% The program will take…
Q: 30.
1
[² ( 1/3 + 1 = 0 + 1 = 10²/3) dw
8
Q: 6) dilation of 2 about the origin
H.
Q: Solid sodium reacts with liquid water to form hydrogen gas according to the equation 2 Na(s) + 2…
Q: FALL QUARTER 2017
Letter Grade Credits
3
2
Course
CHEM 140
CHEM 141
ENGL 101
MATH&151
F
B
D
B
5…
Q: 데
2
This is a Matrix multiplication of C with the transpose of C
Given C
=
find CCT.
Q: N
To measure the amount of nickel in some industrial waste fluid, an analytical chemist adds 0.190M…
Q: This depicts the probability the mans runs between 4.36 and 6.2 mph.
Q: Presented below is the trial balance of Flounder Corporation at December 31, 2020.
Cash
Sales
Debt…
Q: Use Newton's method to approximate a root of the equation 5x^3+5^x+2=0 as follows.
Let x1=−2 be the…
Q: 48. f" (t) = t² + 1/t², t>0, f(2)=3, f' (1)=2
Q: Phosphoric acid, a mild acid used among other things as a rust inhibitor, food additive, and etching…
Q: Consider the equilibrium system described by the chemical reaction
below. For this reaction, Kc =…
Q: (a)
Br
LDA
-78deg
1.) 03'
2.) Me₂S
NaBH4
ethanol
Q: What are the fixed and variable cost?
Q: Using the given graph of the function f, find the following.
Q: Write the balanced NET ionic equation for the reaction when (NH₄)₂CO₃ and CaCl₂ are mixed in aqueous…
Q: O
osoft C
To measure the amount of citric acid
(C,H,O(CO,H),)
water and titrates this solution to…