pdf
keyboard_arrow_up
School
Simon Fraser University *
*We aren’t endorsed by this school
Course
120
Subject
Computer Science
Date
Oct 30, 2023
Type
Pages
8
Uploaded by xiaofanfan
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
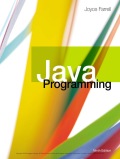
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
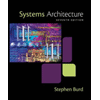
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:9780357392607
Author:FREUND
Publisher:Cengage
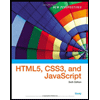
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNp Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:Cengage
- Microsoft Windows 10 Comprehensive 2019Computer ScienceISBN:9780357392607Author:FREUNDPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
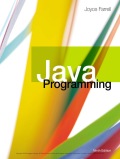
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
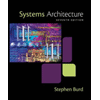
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:9780357392607
Author:FREUND
Publisher:Cengage
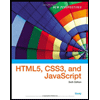
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
Browse Popular Homework Q&A
Q: In Python, write a function named cipher that takes a string.
The function returns that string in…
Q: 3 in..
Fig. P5.120
1.6 in.
4 in.
2.5 in.
Q: A coffee maker is rated at 0.99 kW when connected to a 120 V source.
(a) What current (in A) does…
Q: Identify for each table, the followings:
Foreign keys
Candidate keys
Primary key
Alternate keys
Q: A grade 36 round steel bar with a diameter of 0.5 inches and a gauge length of 2 inches was…
Q: A company has operating income of $300,000, revenues of $1,500,000, total assets of $2,000,000…
Q: 9. True or False? In the production model we studied, TFP can be greater than 1.
A. True
B. False
Q: d. x² + 5x - 24
e. 4x³6x² - 10x
f. x² - 10x + 25
Q: Let y = 4x?.
Find the change in y, Ay when x = 5 and Aæ
= 0.1
Find the differential dy when x =
5…
Q: Let Z be a standard normal random variable. Use the z‐table to find P(Z > ‐1.30)?
Q: How to determine if this is N- ethylsaccharin or O-ethylsaccharin or a mixture of both based on…
Q: Solve this linear programming problem using graphical methods. Restrict
x ≥ 0
and
y ≥ 0.
Maximize…
Q: Why do we have a Law / Technology Delta – what causes the gap between our laws and our technology?…
Q: 115
Find the lenath of the side adiacent to the given angle. (Round vour answer to two decimal plac
Q: Similarly, approaching along the y-axis yields a limit equal to 0. Since these two limits are the…
Q: Let y = 4x?.
Find the change in y, Ay when x = 5 and Aæ
= 0.1
Find the differential dy when x =
5…
Q: eeting today with the office manager at the law firm Abercrombie and Wilson (A&W). A&W is a la
ve…
Q: The expression (x-7)(x²+2x+5) equals Ax³ + Bx² + C + D
where A equals:
and Bequals:
and C equals:…
Q: What concept did James Hutton and Charles Lyell introduce?
Q: What term is used to describe the checkpoints (Go/No-go decision points) that occur during a project…
Q: Organotin compounds play a significant role in diverse industrial applications. They have been used…
Q: Consider the function f(x)=2x3−3x2−36x+54f(x)=2x3-3x2-36x+54.
Find the first derivative.…
Q: You plan to buy a house in three years and would like to put $100,000 down at the time of buying.…
Q: a. f(t) = cos(2t). Find F(s)
b. g(t) = e²t sin(3t). Find G(s)
c. H(s)
=
3s +1
s² - 2s + 5*
Find h(t)
Q: The processing of incoming information in the central nervous system and deciding what to do with…